How to limit the maximum value of a numeric field in a Django model?
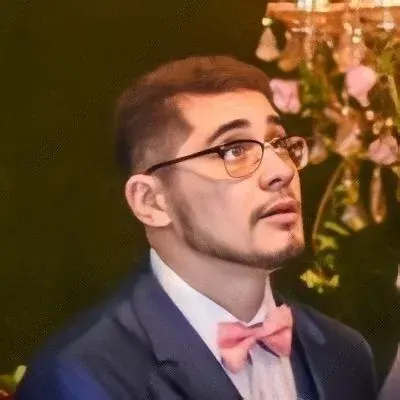
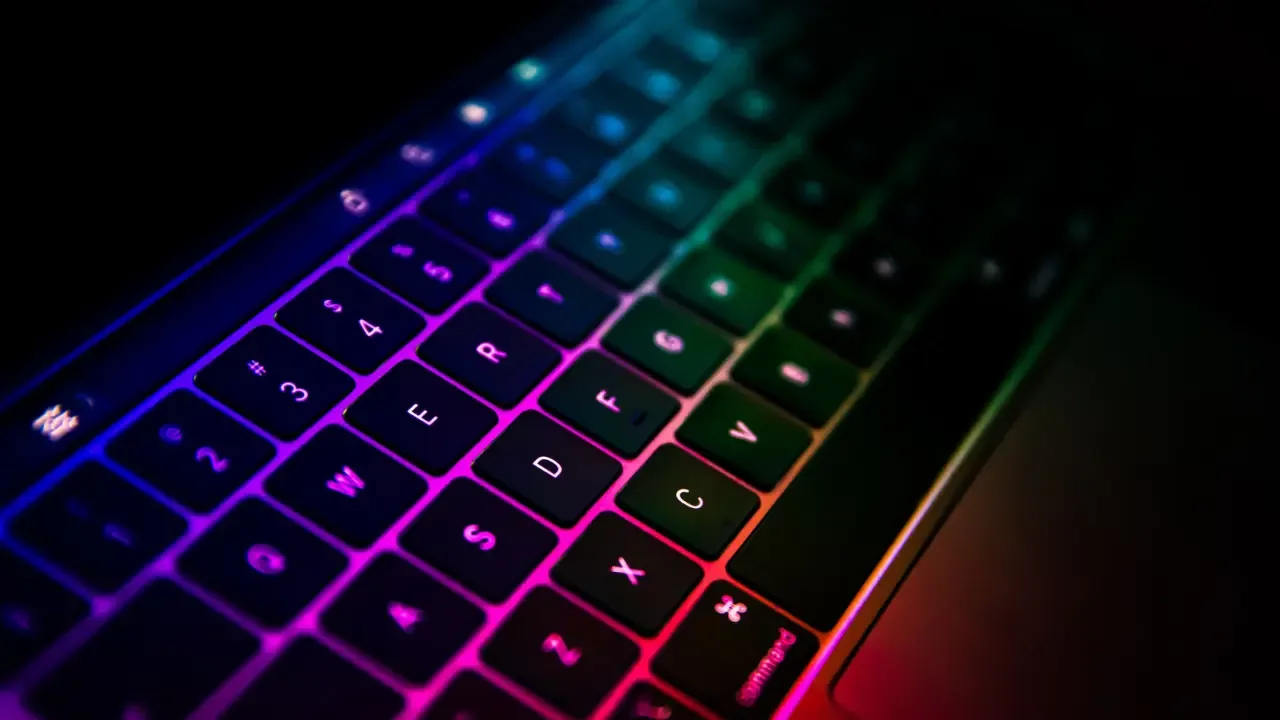
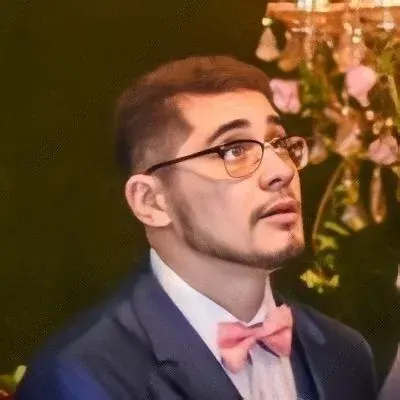
How to Limit the Maximum Value of a Numeric Field in a Django Model? π’π
So you're working on a Django project and you have a numeric field in one of your models. You want to restrict the values that can be entered into that field to a specific range or a maximum value. In this blog post, we'll explore the common issues faced when trying to achieve this and provide you with easy solutions to tackle this problem. Let's dive right in! πββοΈ
The problem: No built-in way to limit numeric fields π«
Django offers a range of numeric fields, such as DecimalField
and PositiveIntegerField
, but unfortunately, they don't natively provide a way to limit the maximum value of the field. π
For example, let's consider the DecimalField
which allows you to define the number of decimal places stored and the overall number of characters stored. But what if you want to go a step further and restrict the field to only store numbers within a certain range, like 0.0-5.0? π€
Similarly, the PositiveIntegerField
allows you to store only positive integers, but limiting it to a specific maximum value, like 50, seems to be a challenge. π©
The solution: Custom Validators to the rescue! π¦ΈββοΈβ¨
Fortunately, Django provides a way to add custom validators to model fields. By creating a custom validator, we can easily enforce the range or maximum value constraints on our numeric fields. π
Let's see how we can implement this solution for both DecimalField
and PositiveIntegerField
.
1. Limiting a DecimalField
to a specific range π―
To restrict a DecimalField
to a specific range, we can define a custom validator function. Here's an example:
from django.core.exceptions import ValidationError
def validate_range(value):
if value < 0.0 or value > 5.0:
raise ValidationError('Value must be between 0.0 and 5.0')
class YourModel(models.Model):
your_field = models.DecimalField(validators=[validate_range], max_digits=3, decimal_places=1)
In the above code, we define a validate_range
function that checks whether the given value falls within the desired range. If not, the function raises a ValidationError
with a user-friendly error message. We then apply the validator to your_field
by including it in the validators
attribute.
2. Limiting a PositiveIntegerField
to a maximum value β‘οΈ
For restricting a PositiveIntegerField
to a maximum value, we can again make use of a custom validator. Here's an example:
from django.core.exceptions import ValidationError
def validate_max_value(value):
if value > 50:
raise ValidationError('Value must be 50 or lower')
class YourModel(models.Model):
your_field = models.PositiveIntegerField(validators=[validate_max_value])
In this case, we define a validate_max_value
function that checks whether the given value is greater than 50. If so, it raises a ValidationError
with a user-friendly error message. We apply this validator to your_field
by including it in the validators
attribute.
Wrap up and start limiting those numeric fields! ππ
By using custom validators, you can easily limit the maximum value of numeric fields in Django models. Whether you want to restrict a DecimalField
to a specific range or a PositiveIntegerField
to a maximum value, these solutions have got you covered! πͺ
Now it's time to put this knowledge to practice and start enforcing those constraints on your numeric fields. Don't let your data exceed boundaries! π§
If you have any questions or suggestions, feel free to leave a comment below. Happy coding! π»π
Update: The StackOverflow question mentioned in the context has been closed, rendering this blog post moot. Nonetheless, the solutions provided can still be useful when dealing with similar issues in your Django projects.
Header image source: Pexels