How to input a regex in string.replace?
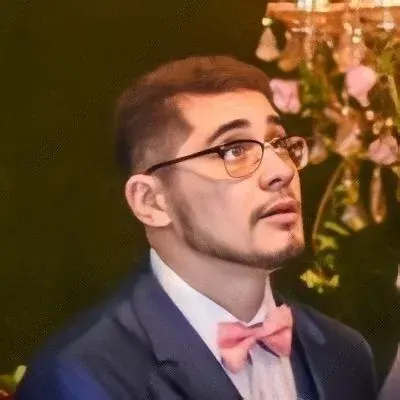
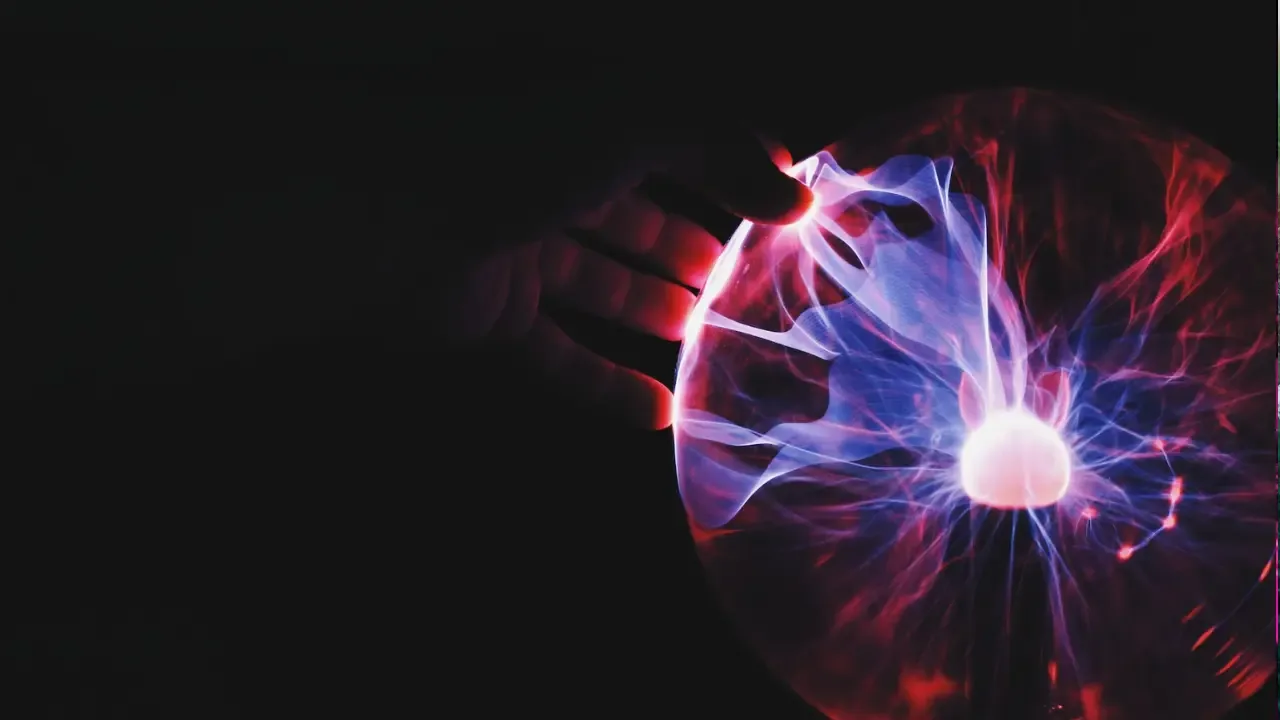
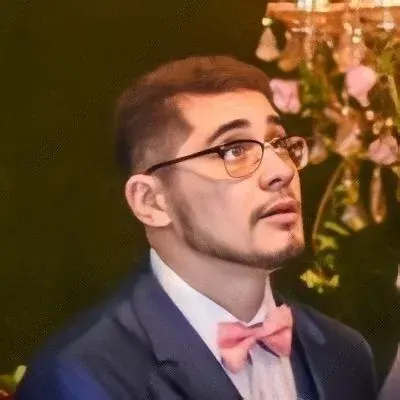
How to Input a Regex in string.replace?
Hey there tech enthusiasts! 👋 Are you struggling with declaring a regex while using string.replace
? Don't worry, you're not alone! 😅 In this blog post, we'll address this common issue and provide you with easy solutions to solve it. So let's dive in and conquer this regex challenge together! 💪
The Challenge
The challenge at hand is to replace specific patterns in a string using regex, without hard-coding the replacement values. 🤔 The example provided gives us a clear context: we need to remove certain tags, such as "<[1>", "</[1>", etc., from a string.
Solution 1: Using Multiple replace
Statements
The initial attempt demonstrated in the code snippet is on the right track, but it falls a bit short in terms of elegance and efficiency. It uses multiple replace
statements to remove the desired tags, but it is not scalable as it requires hard-coding values from 1 to 99. 🤷♂️ Is there a better way? Absolutely!
Solution 2: Utilizing Regular Expressions (Regex)
Regex to the rescue! 🚀 By leveraging the power of regex, we can achieve a dynamic and flexible solution. Instead of using multiple replace
statements, let's use a single regex pattern to match and replace the desired tags.
To accomplish this, we'll use the re.sub
function in Python, which accepts a regex pattern and allows us to specify a replacement string. Here's the updated code snippet:
import os
import re
import glob
for infile in glob.glob(os.path.join(os.getcwd(), '*.txt')):
with open(infile, 'r') as reader:
for line in reader:
line = re.sub(r'<\/?\[\d+\]>\s*', '', line)
print(line)
In this code, we've replaced the multiple replace
statements with a single re.sub
call. The regex pattern r'<\/?\[\d+\]>\s*'
matches the tags we want to remove, including the optional closing tag, such as "<[1>" or "</[1>". The \d+
part matches one or more digits (1-100 in this case), and \s*
matches any whitespace following the tag.
Voila! 🎉 This new approach allows for a simple and scalable solution using regex.
Take It Further
Now that you have gained a better understanding of using regex with string.replace
, it's time to put your newfound knowledge into practice! Try out this updated code snippet on your own data set and share your experience with us.
Feel free to share your thoughts, questions, or any other cool regex tricks you've discovered while working on this challenge. We'd love to hear from you! 😊
Happy regexing! 🎩🐇