How to get the position of a character in Python?
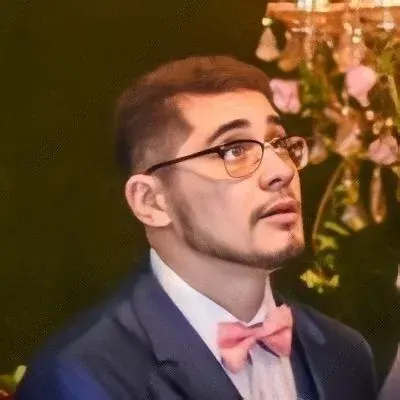
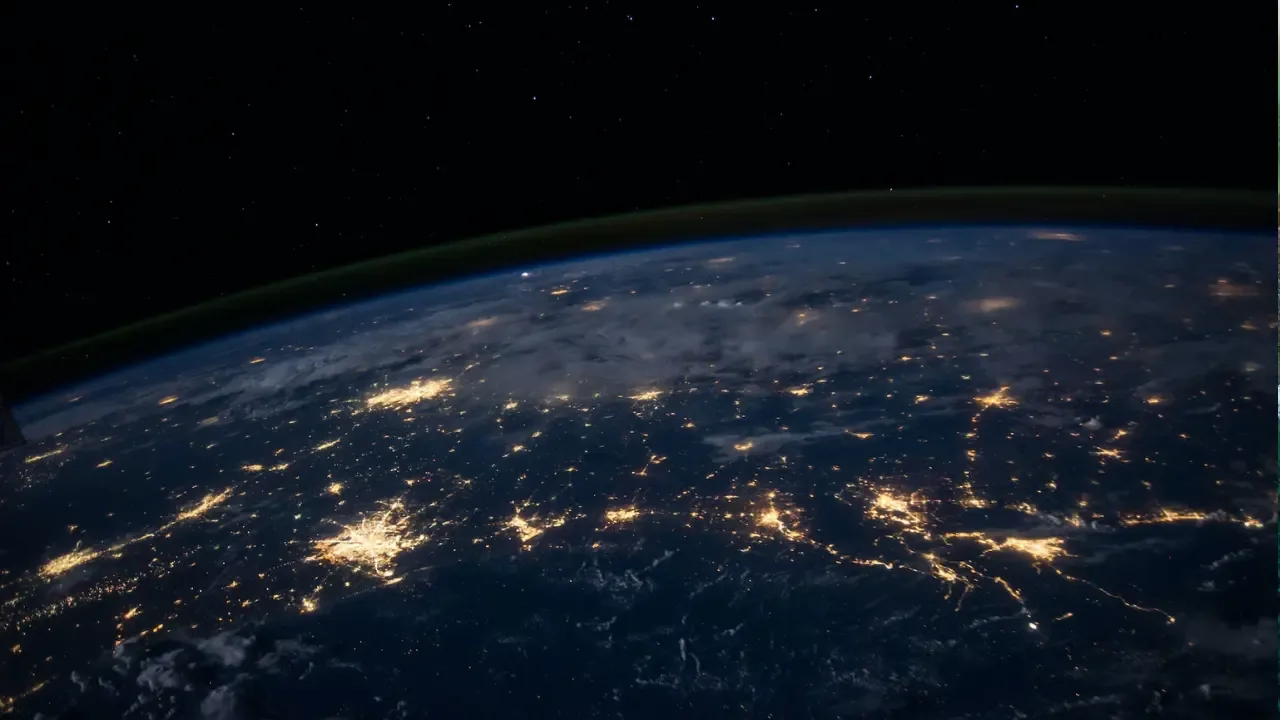
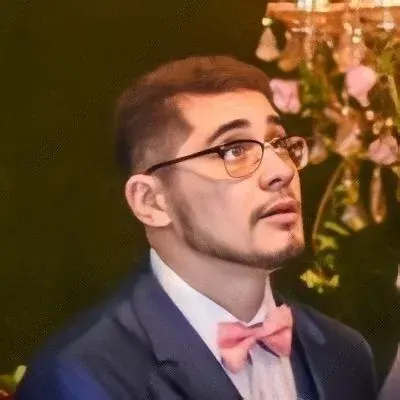
π How to Get the Position of a Character in Python? ππ»
Ever been stuck trying to figure out how to get the position of a character inside a string in Python? π€ Don't worry, you're not alone! It's a common challenge many developers face when manipulating strings.
So let's dive in and explore some easy solutions to this problem! π
The Index Method
Python provides a simple and straightforward method called index()
that can help us find the position of a character within a string. Here's an example:
string = "Hello, World!"
position = string.index("o")
print(position) # outputs 4
In the above code snippet, we create a string variable string
with the value "Hello, World!" π. Then, we use the index()
method to find the position of the letter "o" within the string. Finally, we print the result, which should be 4
, as the index positions in Python start from 0. π
π¨However, keep in mind that if the character you're searching for is not present in the string, the index()
method will raise a ValueError
. So it's a good practice to handle this situation using a try-except block.
string = "Hello, World!"
try:
position = string.index("z")
print(position)
except ValueError:
print("Character not found in string!")
The Find Method
Another option is to use the find()
method, which works similarly to index()
. The main difference is that find()
returns -1 if the character is not found, instead of raising a ValueError
. Check out this example:
string = "Hello, World!"
position = string.find("o")
print(position) # outputs 4
By leveraging the simplicity and power of these methods, we can effortlessly determine the position of a character within a string in Python. π
Your Turn! π£
Now it's your time to try it out! Open up your favorite Python IDE or code editor, and experiment with these methods to get comfortable using them. πͺ
And remember, don't hesitate to reach out if you have any questions or if you need further assistance. We're here to help you on your coding journey! π
Happy coding! π»π