How to get the domain name of my site within a Django template?
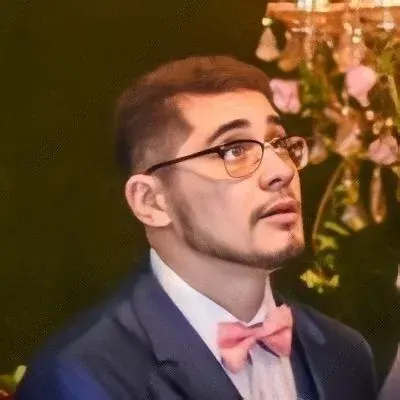
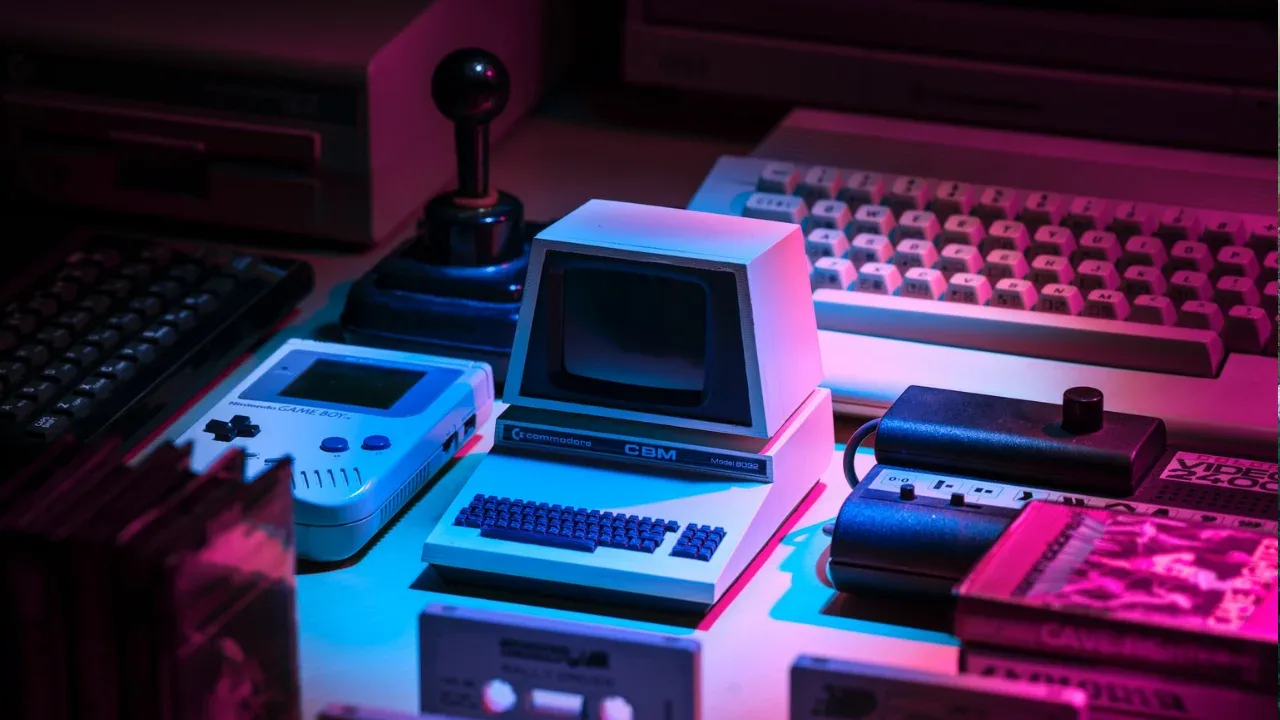
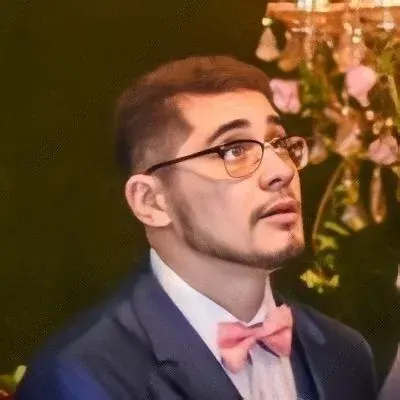
🌐🏠 How to Get the Domain Name of Your Site Within a Django Template
So you've built your awesome website using Django, and now you want to retrieve the domain name of your site within a Django template. 🤔 It may seem like a tricky task at first, but fear not! We've got you covered with some simple solutions. Let's dive right in! 💪
The Problem
A user approached us with the following query: "🤷♀️ How do I get the domain name of my current site from within a Django template? I've tried looking in the tag and filters but found nothing there. 😩"
The Solution
Fortunately, Django provides a built-in variable called request
that gives us access to the request object. By using this object, we can easily extract the domain name of our site. 🎉
Here's a step-by-step guide on how to get the domain name within a Django template:
Start by passing the
request
variable to your template context.In your view function or class-based view, make sure you include
'django.core.context_processors.request'
in your template context processors. For example:from django.shortcuts import render def my_view(request): return render(request, 'my_template.html', {'request': request})
Now, inside your Django template (
my_template.html
, in this case), you can access the domain name using therequest
variable like this:<p>The domain name of this site is: {{ request.get_host }}</p>
In the above code,
request.get_host
returns the domain name, including the protocol and any port number if present.
Voila! 🎈 That's all you need to do to obtain the domain name of your site from within a Django template. It's that simple!
Example Usage
Let's see an example to illustrate how this works in practice:
Assuming your website is hosted at https://www.example.com
, and you have a template called home.html
, you can get the domain name dynamically in your template using the request
variable:
<!-- home.html -->
<!DOCTYPE html>
<html>
<body>
<h1>Welcome to {{ request.get_host }}!</h1>
<p>Feel free to explore our awesome site!</p>
</body>
</html>
In this case, when a user visits https://www.example.com
, they will see:
Welcome to www.example.com!
Feel free to explore our awesome site!
Call-to-Action
We hope this guide has helped you efficiently retrieve the domain name within a Django template. If you have any more questions or need further assistance, feel free to reach out! Let us know in the comments below if you found this blog post helpful or if you have any other Django-related topics you'd like us to cover. Happy coding! 🚀✨