How to get/set a pandas index column title or name?
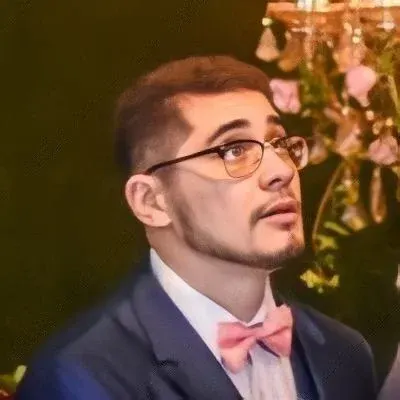

π’π Unlocking the Mystery of Pandas Index Column Titles or Names
Are you feeling puzzled about how to play with pandas index column titles? Don't worry, we've got you covered! In this blog post, we will explore the common issues and provide easy solutions to help you master the art of getting and setting pandas index column titles or names. Let's dive in! πΌπͺ
To better illustrate the problem at hand, let's take a look at a sample dataframe:
Column 1
Index Title
Apples 1
Oranges 2
Puppies 3
Ducks 4
The question is, "How do we get or set the index column title?"
One possible approach could be trying to assign the values of "Index Title" to the actual index column. Here's what it looks like:
import pandas as pd
data = {'Column 1' : [1., 2., 3., 4.],
'Index Title': ["Apples", "Oranges", "Puppies", "Ducks"]}
df = pd.DataFrame(data)
df.index = df["Index Title"]
del df["Index Title"]
But, wait! π€ This approach seems a bit off-target. Let's explore an easier way to get/set the index column title.
Getting the Index Column Title
To retrieve the index column title, we can use the index.name
attribute of the DataFrame. Here's an example:
import pandas as pd
data = {'Column 1' : [1., 2., 3., 4.],
'Index Title': ["Apples", "Oranges", "Puppies", "Ducks"]}
df = pd.DataFrame(data)
index_title = df.index.name
print(index_title) # Output: Index Title
In this snippet, index.name
returns the name/title of the index column, which is "Index Title" in our case. You can store this value in a variable or use it directly as your heart desires. π
Setting the Index Column Title
Now, let's move on to setting the index column title. We can use the rename_axis()
method to achieve this. Here's how it works:
import pandas as pd
data = {'Column 1' : [1., 2., 3., 4.],
'Index Title': ["Apples", "Oranges", "Puppies", "Ducks"]}
df = pd.DataFrame(data)
df.rename_axis("New Title", inplace=True)
print(df)
After executing this code snippet, you will see that the index column title has been successfully updated to "New Title". π
By chaining the inplace=True
parameter, we modify the DataFrame in place and avoid unnecessary assignments.
Conclusion and Call-to-Action
Congratulations! π You are now equipped with the knowledge to easily get and set pandas index column titles. Say goodbye to confusion and hello to productive pandas coding!
Go ahead, apply your newfound skills, and solve fascinating data manipulation problems using pandas. Don't hesitate to share your pandas πΌ success stories with us. We would love to hear how you put these techniques into action.
If you have any questions or just want to chat about all things pandas, feel free to connect with us in the comments section below. Happy coding! ππ»
π References:
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
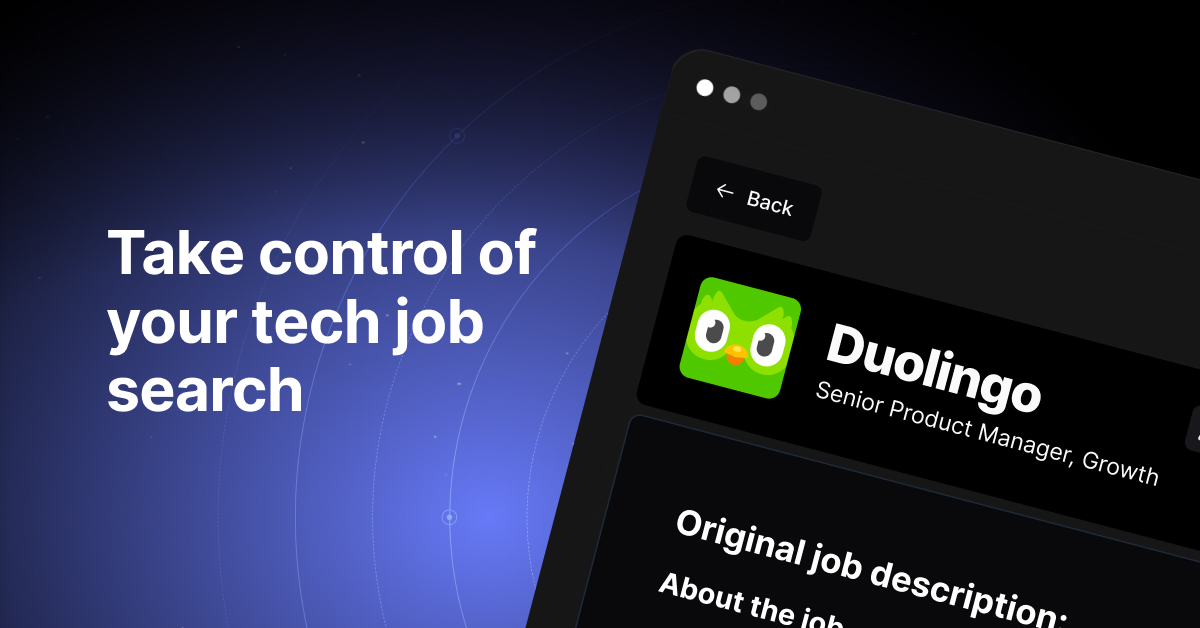