How to get rid of "Unnamed: 0" column in a pandas DataFrame read in from CSV file?
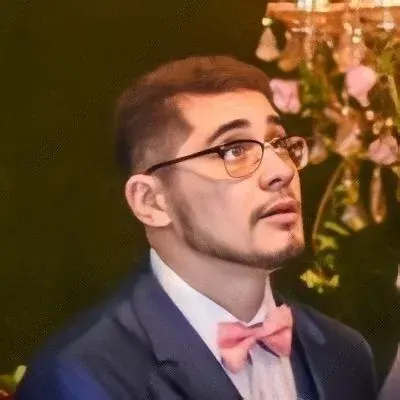
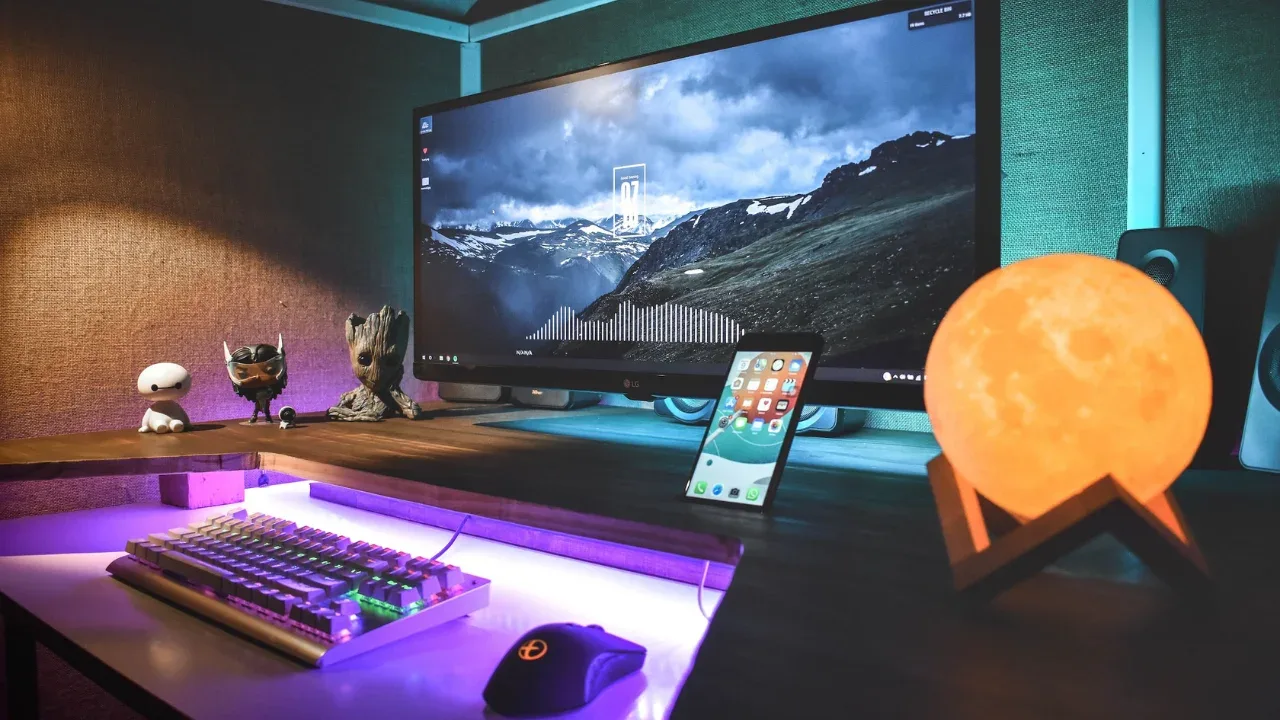
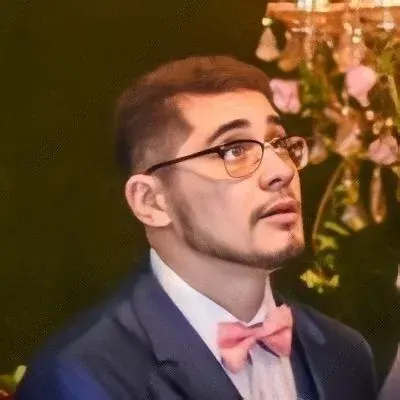
📝 Hello there readers! Are you tired of dealing with the pesky "Unnamed: 0" column that magically appears when you read a CSV into a pandas DataFrame? 🤔 Don't worry, you're not alone! It's a common issue that many pandas users face. But fear not, because I'm here to guide you through the process of getting rid of this unwanted column. Let's dive in! 💪
First, let's take a look at an example CSV file that has this "Unnamed: 0" column:
,A,B,C
0,1,2,3
1,4,5,6
2,7,8,9
When we read this CSV file using the pd.read_csv()
function, we end up with the following DataFrame:
>>> import pandas as pd
>>> df = pd.read_csv('file.csv')
>>> df
Unnamed: 0 A B C
0 0 1 2 3
1 1 4 5 6
2 2 7 8 9
🚫 Ah, the "Unnamed: 0" strikes again! But don't fret, because removing it is actually quite simple.
To get rid of the "Unnamed: 0" column, all you need to do is specify the index_col
parameter while reading the CSV file. This parameter allows you to set a specific column as the index, effectively removing the unwanted column.
Here's how you can do it:
>>> df = pd.read_csv('file.csv', index_col=0)
>>> df
A B C
0 1 2 3
1 4 5 6
2 7 8 9
🎉 Voila! The "Unnamed: 0" column is now gone, and you're left with the DataFrame you desired. Simple, right?
But what if your CSV file doesn't have a column name that you can specify as the index? Don't worry, we have a solution for that too! 🙌
You can use the usecols
parameter to explicitly select the columns you want to read from the CSV file. By excluding the "Unnamed: 0" column, you effectively get rid of it.
Here's an example:
>>> df = pd.read_csv('file.csv', usecols=['A', 'B', 'C'])
>>> df
A B C
0 1 2 3
1 4 5 6
2 7 8 9
✨ Amazing! The "Unnamed: 0" column is now history, and you have full control over which columns to include in your DataFrame.
Now it's your turn to give it a try! Go ahead and implement these solutions in your code to get rid of that annoying "Unnamed: 0" column once and for all. And if you have any other pandas-related questions or issues, feel free to reach out or explore more articles on our tech blog. We're here to help! 🤗
💡 Keep in mind that you can further customize the pd.read_csv()
function using various parameters to handle different scenarios and formats. You can refer to the pandas documentation for more details: pandas.read_csv()
So, let's bid farewell to the "Unnamed: 0" column and embrace cleaner DataFrames! Happy coding! 💻🐼✨
📢 Have you ever struggled with the "Unnamed: 0" column in pandas? Share your experience or any additional tips in the comments below! Let's help each other out and make our pandas journeys even smoother. 😊✍️