How to get POSTed JSON in Flask?
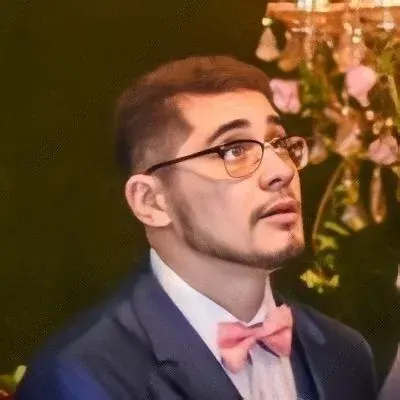
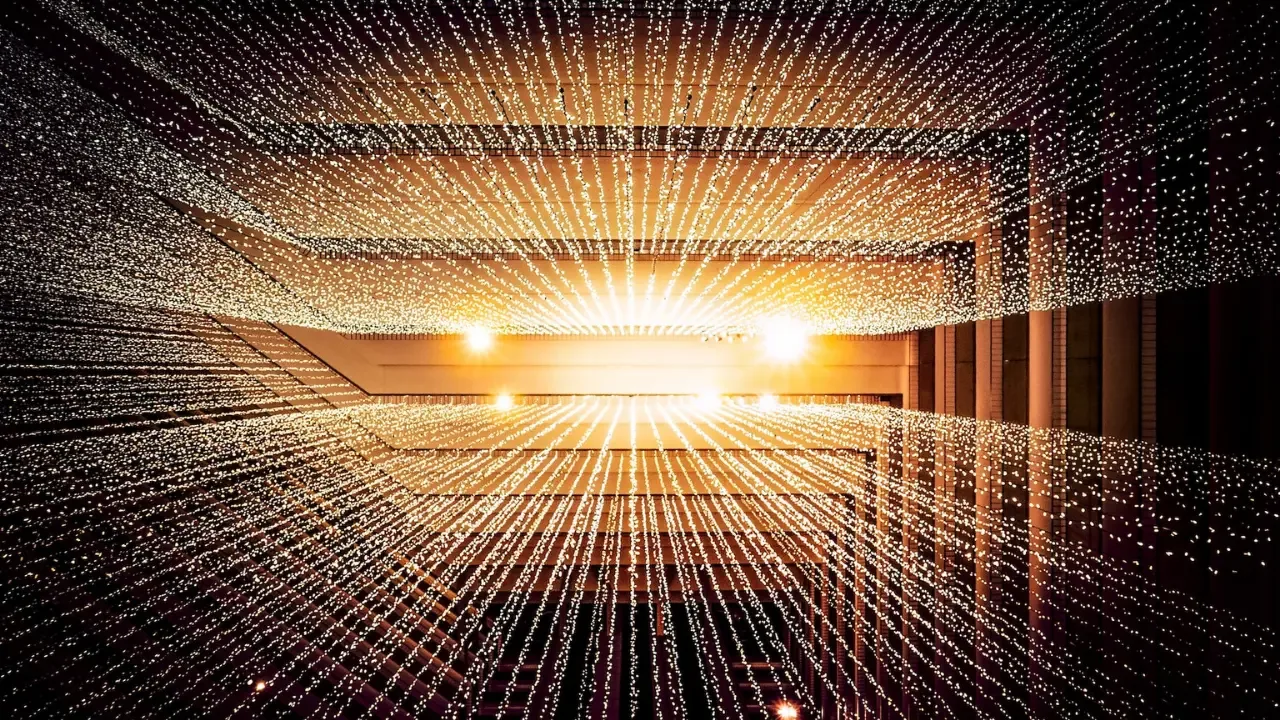
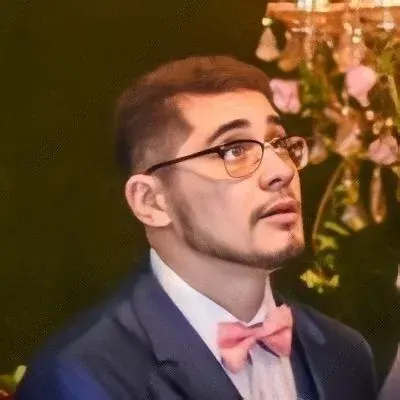
📝 How to Get POSTed JSON in Flask?
Are you building a simple API using Flask and facing difficulties in reading POSTed JSON? Don't worry, we've got you covered! In this easy-to-follow guide, we will address common issues and provide you with simple solutions to get that precious JSON data. So, let's dive in! 💪
The Problem
One of our fellow developers encountered a problem while trying to read POSTed JSON in Flask. They were using the Postman Chrome extension to send a POST request with JSON data. However, when they tried to access the JSON within the Flask method, it returned None
instead of the expected JSON object.
The Solution
To obtain the POSTed JSON data in Flask, you need to make sure you are using the right method and accessing the data correctly. Here's what you need to do:
Import the necessary Flask modules in your code:
from flask import Flask, request
Define the route for your API endpoint:
@app.route('/api/add_message/<uuid>', methods=['GET', 'POST']) def add_message(uuid): # Access the JSON data using request.get_json() content = request.get_json() # Check if content is not None before proceeding if content is not None: print(content) # Print the POSTed JSON data else: return 'Invalid JSON data' # Handle invalid JSON data return uuid
Now, when you send a POST request with JSON data, you should be able to retrieve it within the Flask method using
request.get_json()
.
Let's Break It Down
The
request
object in Flask gives you access to incoming request data. In our case, we need to userequest.get_json()
to retrieve the JSON data.It's important to check if the
content
variable contains the expected JSON object before performing any operations on it.If the JSON data is invalid or does not exist, you can handle it appropriately by returning an error message or taking alternative actions.
That's it! You can now easily get POSTed JSON in your Flask application. 🎉
If you have any further questions or face any issues, feel free to leave a comment below. We're here to help! 💬
Call to Action
Are you ready to unlock the power of Flask and build amazing APIs? Check out our API development guide for more tips and tricks to level up your skills. 🚀
Remember, learning together is more fun! Share this post with your fellow developers who might find it useful. Let's spread the knowledge! 👥✨
Happy coding! 💻