How to get absolute path of a pathlib.Path object?
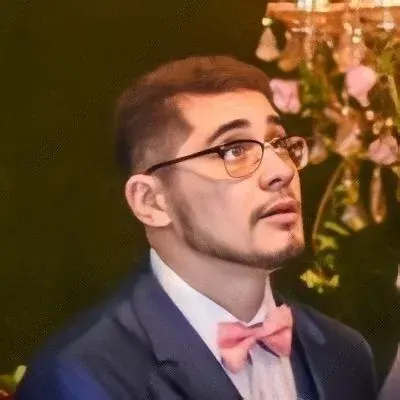
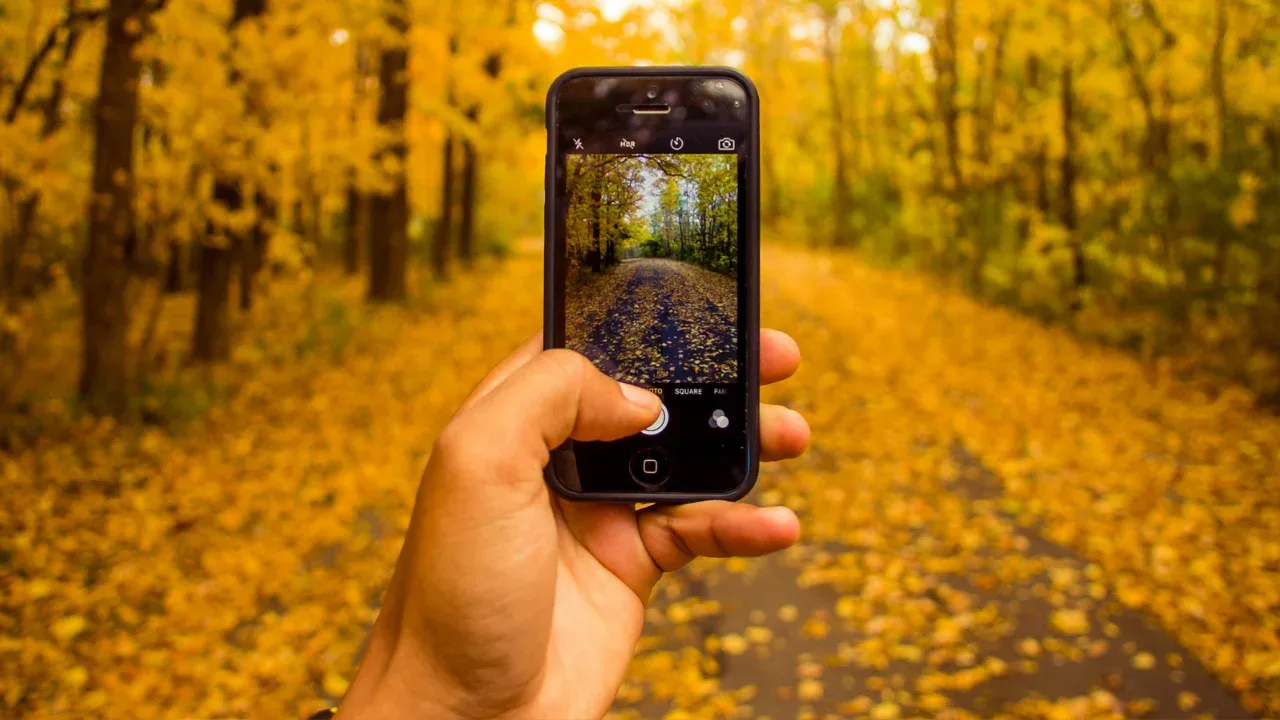
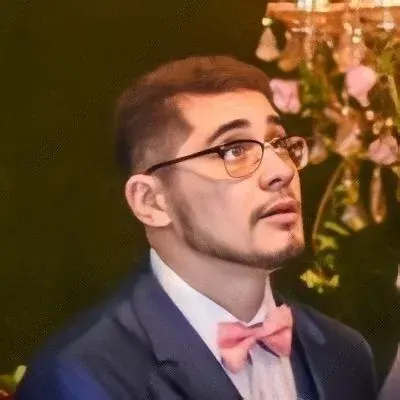
How to Get the Absolute Path of a pathlib.Path Object 📂
So, you have a pathlib.Path object that points to a file in the filesystem, and now you want to get the absolute path of that object in a string.
But wait, you're probably thinking - shouldn't there be a pathlib method to do this? After all, pathlib is supposed to be a replacement for os.path!
Well, fear not! While there doesn't seem to be a direct pathlib method, we can still easily get the absolute path using a simple workaround.
🧱 Solution:
The trick is to call the resolve()
method on the pathlib.Path object. Here's how you can do it:
import pathlib
p = pathlib.Path('file.txt')
absolute_path = p.resolve()
And that's it! The resolve()
method resolves the absolute path of the Path object and returns a new Path object representing it.
🤔 But Why?
You might be thinking, "Why do I have to call resolve()
instead of having a more straightforward method?"
Well, the reason is that pathlib.Path objects are designed to be path manipulators rather than file system accessors. They provide a lot of flexibility in handling paths, but the actual file operations are left to other methods and functions.
By separating these concerns, pathlib keeps things neat and organized. And don't worry - calling resolve()
is a simple and intuitive way to get the absolute path you need.
📝 Example:
Just to make things crystal clear, let's go through an example:
import pathlib
p = pathlib.Path('file.txt')
absolute_path = p.resolve()
print(absolute_path)
Output:
/home/user/file.txt
In this example, the absolute_path
variable now holds the absolute path of the p
Path object as a string.
📣 Call to Action:
And there you have it! Now you know how to get the absolute path of a pathlib.Path object using the resolve()
method. 😎
Next time you find yourself in a similar situation, remember this neat trick. Keep coding and exploring! ✨
If you found this blog post helpful, feel free to share it with your fellow developers. And don't forget to leave a comment if you have any questions or cool ideas! 👇🔥
Happy coding! 🚀