How to get a function name as a string?
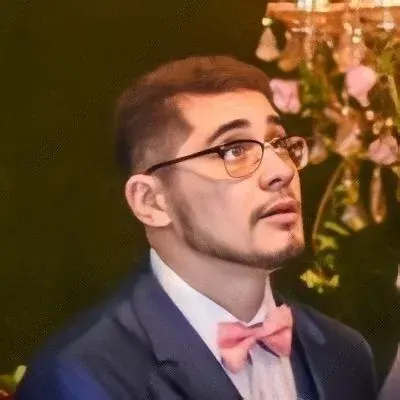
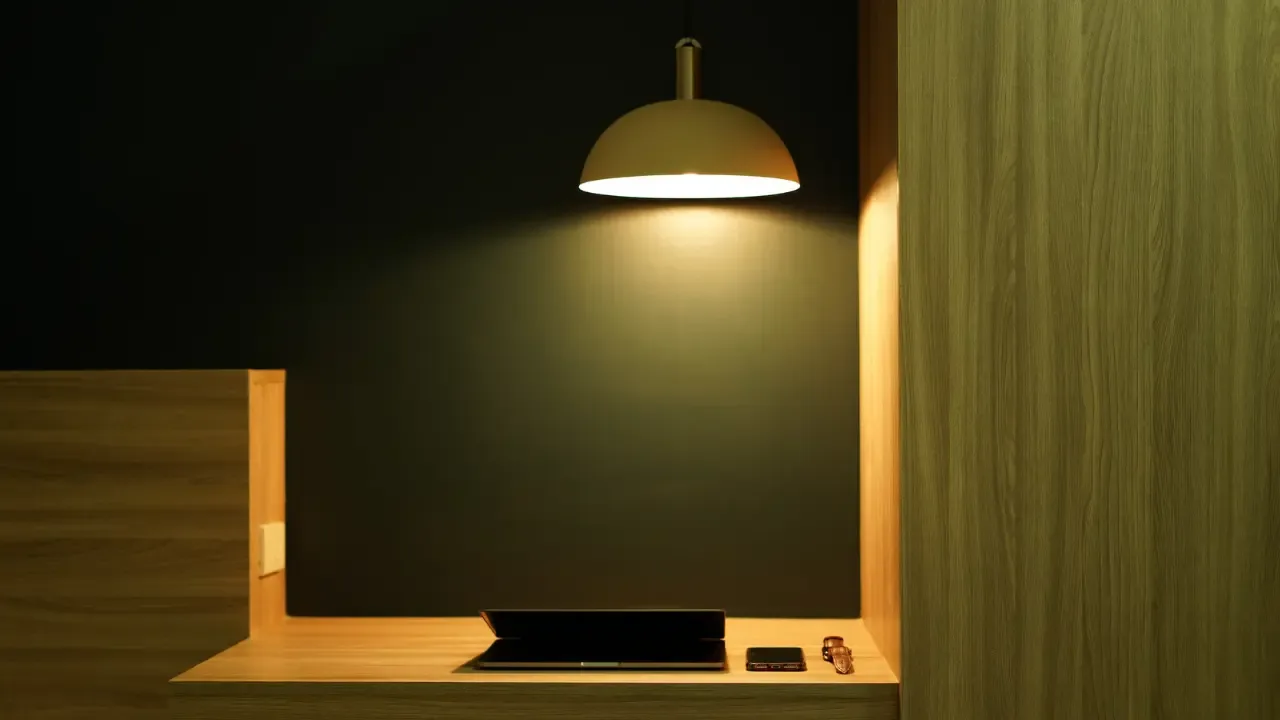
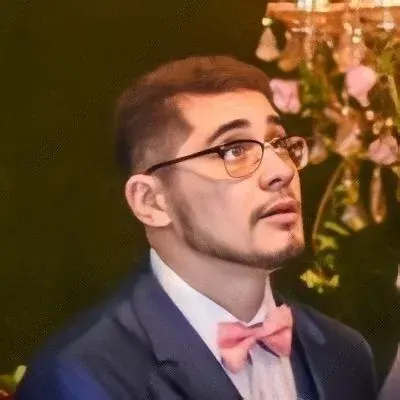
How to Get a Function Name as a String?
So, you want to know how to get a function's name as a string? Maybe you're working on some fancy Python code and you need to dynamically retrieve the name of a function. Or perhaps you're just curious and want to know if it's even possible. Well, you're in luck! In this blog post, we'll address this common issue and provide easy solutions for you.
The Problem 🤔
Let's take a closer look at the example you provided:
def foo():
pass
>>> name_of(foo)
"foo"
In this scenario, name_of
is a hypothetical function that we wish existed to give us the name of foo
. Unfortunately, Python doesn't have a built-in function to directly get the name of a function. But fear not! We have a few workarounds that can help us achieve this.
Solution 1: __name__
Attribute 😎
One way to get the name of a function is by using the __name__
attribute. This attribute holds the name of the function as a string. Here's how you can use it:
def foo():
pass
name = foo.__name__
print(name) # Output: "foo"
By accessing the __name__
attribute of the function, we can obtain its name. It's simple, straightforward, and doesn't require any external libraries.
Solution 2: inspect
Module 🕵️♀️
If you're looking for a more versatile solution, you can make use of the inspect
module. This module provides several functions and classes that help you gather information about live objects, including functions.
First, we need to import the inspect
module:
import inspect
Now, let's see how the inspect
module can help us:
def foo():
pass
name = inspect.getframeinfo(foo.__code__).function
print(name) # Output: "foo"
By using the getframeinfo
function from the inspect
module, we can retrieve information about the code frame where our function is defined. From this information, we can extract the function's name.
Call-to-Action: Your Turn! 🚀
That's it! Now you know two simple ways to get a function's name as a string in Python. Whether you prefer using the __name__
attribute or the inspect
module, you have the tools to tackle this problem.
So, why not try it out yourself? Write a little snippet of code using one of the methods we discussed and see if you can successfully extract a function's name as a string. Don't forget to share your experience in the comments below! We'd love to hear from you.
Remember, learning and sharing knowledge is what makes the tech community thrive. So spread the word and share this blog post with your fellow developers. Until next time, happy coding! 💻🔥
Note for Markdown Language: Make sure to remove the HTML tags around the provided code example in the question before using it in the blog post.