How to find which columns contain any NaN value in Pandas dataframe
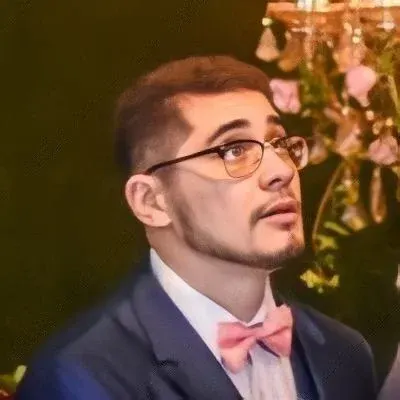
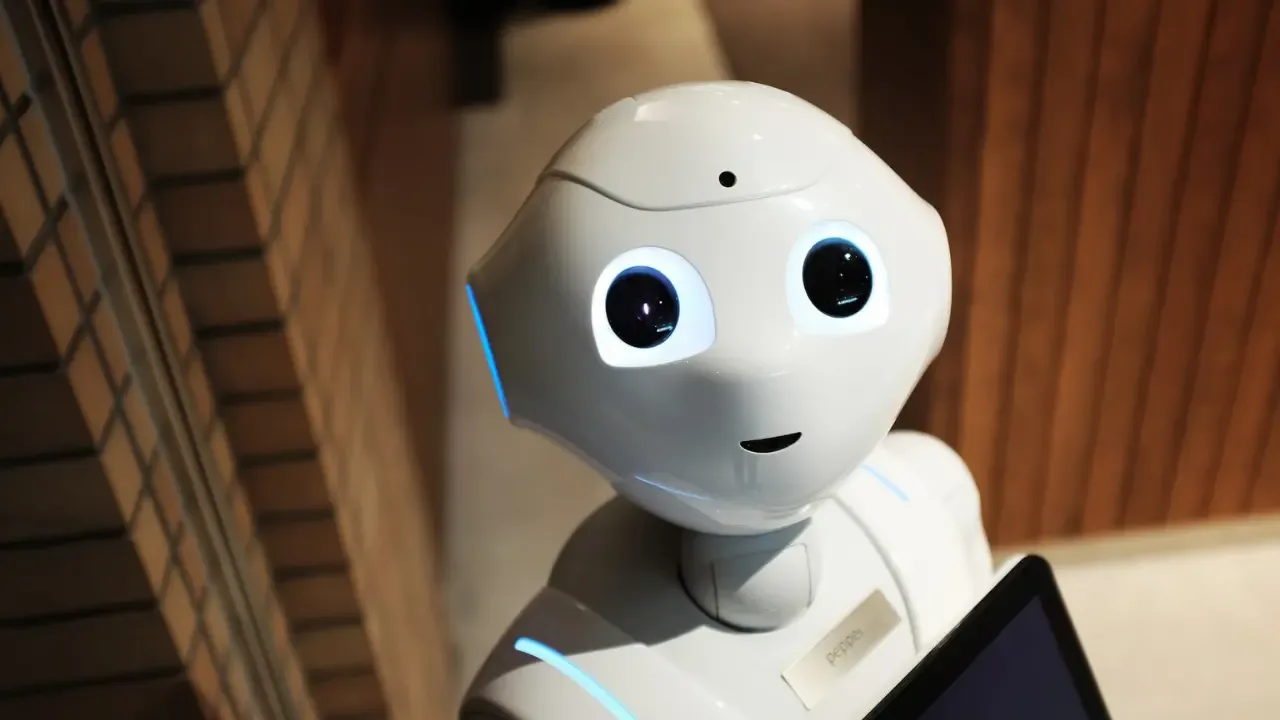
How to Find Which Columns Contain NaN Values in Pandas Dataframe ๐ฎ๐ผ
So, you have a Pandas dataframe with some NaN values scattered here and there, and now you're wondering how to determine which columns contain those pesky NaNs. Fear not, my friend! In this guide, I'll show you how to tackle this common issue and provide you with easy solutions to save the day ๐ฆธโโ๏ธ
The Question: Which Columns Contain NaN Values? ๐ค
Before we dive into the solutions, let's understand the question clearly. We have a dataframe, and we want to find out which columns contain NaN values. Additionally, we want to obtain a list of those column names containing NaNs. Got it! ๐ง ๐ก
Solution #1: Using the isnull() and any() methods ๐ ๏ธ๐ป
The first solution involves using two powerful Pandas methods โ isnull()
and any()
. Here's how you can employ this technique:
# Let's assume your dataframe is named 'df'
columns_with_nans = df.columns[df.isnull().any()].tolist()
Boom! This one-liner will give you a list of column names (columns_with_nans
) that contain NaN values. You can use this list to single out the mischievous columns hiding NaNs ๐ต๏ธโโ๏ธ๐
Solution #2: Leveraging the info() method ๐๐ต๏ธโโ๏ธ
If you want a broader overview of your dataframe, you can take advantage of the info()
method. It provides information about the dataframe, including the number of non-null values in each column. Here's an example:
# Again, 'df' refers to your dataframe
df.info()
Running this code will display a summary of your dataframe, showing you the number of non-null values in each column. By comparing these numbers with the total number of rows in your dataframe, you can identify columns with NaN values.
Solution #3: Utilizing the isnan() and any() functions ๐งช๐๏ธโโ๏ธ
Last but not least, we can rely on the NumPy library to help us tackle this problem. By using the isnan()
function and Pandas' any()
method, we can identify columns with NaN values. Here's how you can implement this solution:
import numpy as np
# Once again, 'df' represents your dataframe
columns_with_nans = df.columns[np.isnan(df).any()].tolist()
Voilร ! This approach will give you a list of column names (columns_with_nans
) that contain NaN values in your dataframe. Time to get rid of those NaNs like a pro! ๐ฉโ๐ฌ๐งช
Get Ready to Tackle Those NaNs! ๐ก๏ธ
By now, you should be well-equipped to handle the task of finding columns with NaN values in your Pandas dataframe. So, grab your coding sword ๐ก๏ธ and bravely face those NaN beasts! ๐ช
If you have any questions or other cool tricks to share, let me know in the comments below! Happy coding! ๐๐ฉโ๐ป
Remember, NaNs won't stand a chance against your Pandas powers! ๐ฆธโโ๏ธ๐ผ
๐ Bonus Tip ๐: If you want to remove NaN values from specific columns, you can use the dropna()
method on those columns directly.
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
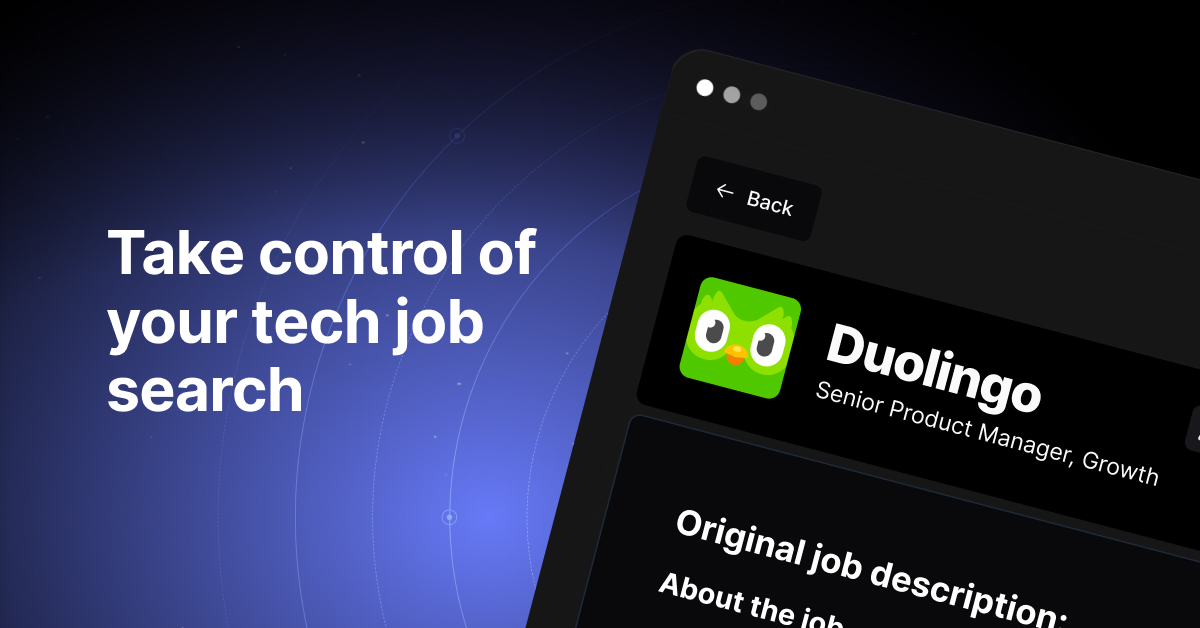