How to filter rows in pandas by regex
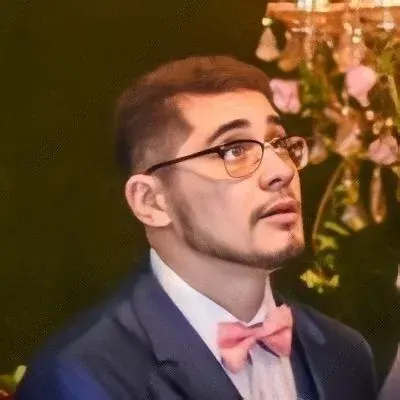
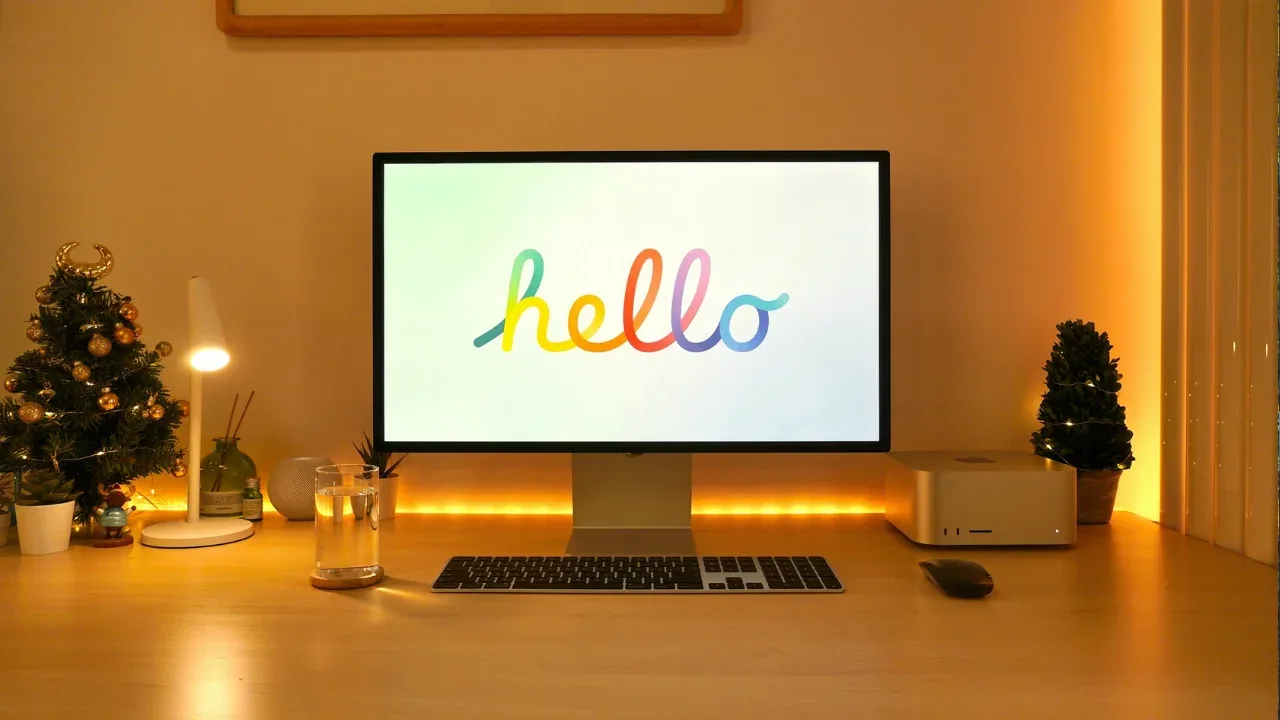
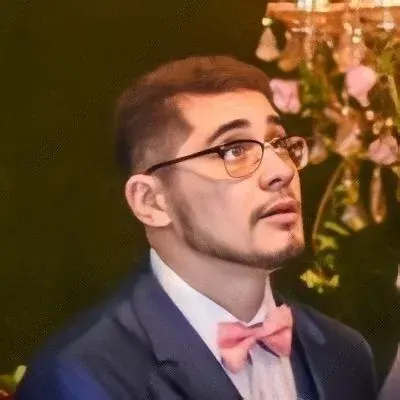
Filtering Rows in Pandas by Regex: A Handy Guide ๐งฉ
Are you tired of manually filtering rows in your Pandas DataFrame using complex regular expressions? Look no further! In this guide, we'll explore an easy and clean way to filter rows based on regular expressions. Say goodbye to convoluted code and hello to efficiency! ๐
The Problem ๐ค
Let's start with the problem at hand. We have a DataFrame called foo
, containing two columns: 'a' and 'b'. We want to filter the rows that start with the letter 'f' using a regex.
Here's an example of the initial DataFrame:
foo = pd.DataFrame({'a': [1, 2, 3, 4], 'b': ['hi', 'foo', 'fat', 'cat']})
Starting with a Regex Matcher ๐ช
Our initial instinct might be to use the str.match()
function from Pandas, which matches the beginning of a string with a regex pattern. We can start by running the following code:
foo.b.str.match('f.*')
Unfortunately, the result is not quite what we expected. Instead, we get an array of empty tuples, like this:
0 []
1 ()
2 ()
3 []
Name: b
Obtaining a Boolean Index โ๏ธ
To get a more useful result, we need to dig a bit deeper. Instead of using str.match()
directly, we can tweak our approach by using the str.len()
function to calculate the length of each matched result. By comparing the length to zero, we can derive a boolean index.
This is how you can achieve it:
foo.b.str.match('(f.*)').str.len() > 0
The output would be:
0 False
1 True
2 True
3 False
Name: b
Filtering Rows Based on the Boolean Index ๐
Now that we have our boolean index, we can finally filter the rows based on the condition. We can achieve this using the boolean index inside square brackets, as shown below:
foo[foo.b.str.match('(f.*)').str.len() > 0]
The resulting DataFrame will contain only the rows where the 'b' column starts with 'f':
a b
1 2 foo
2 3 fat
A Cleaner Approach? ๐งน
The above solution works perfectly fine, but if you're like us, you might think of ways to make it even cleaner. Thankfully, there is!
Instead of artificially wrapping our regex pattern in a group, we can use the str.contains()
function directly. This function checks if a string matches a regex pattern anywhere within it.
Here's the cleaner approach:
foo[foo.b.str.contains('^f')]
In this updated solution, we use the caret symbol (^) before the 'f' character to match the start of the string. The result is the same as before, but with a more elegant solution.
Conclusion and Your Turn! ๐
Filtering rows in Pandas by regex doesn't have to be a daunting task anymore. With our handy guide, you can confidently filter rows based on regex patterns in a clean and efficient way. Say goodbye to messy code!
Now it's your turn to try it out. Experiment with your own DataFrames and unleash the power of regex filtering in Pandas! Don't forget to share your insights and experiences in the comments section below. Happy coding! ๐ปโจ