How to filter rows containing a string pattern from a Pandas dataframe
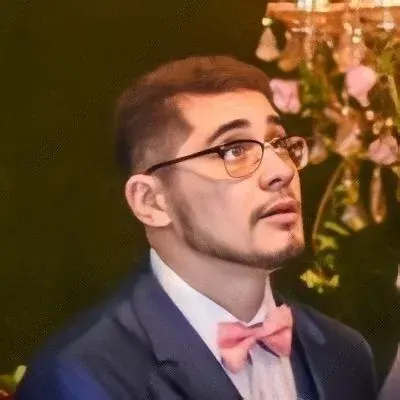
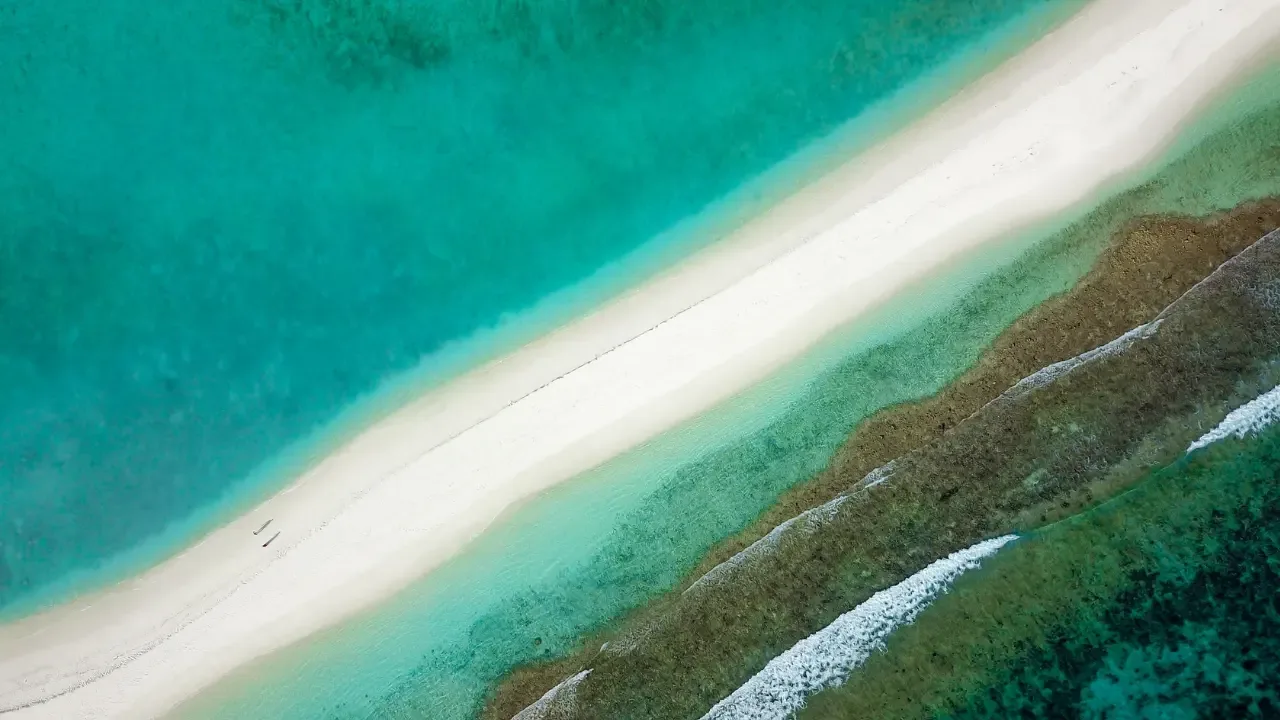
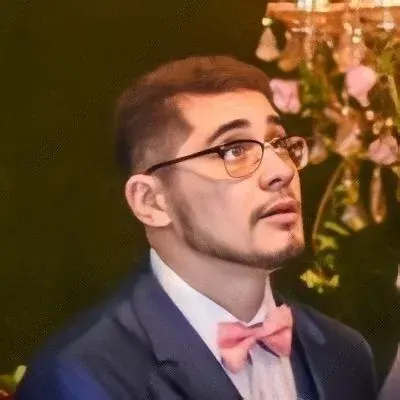
Filtering Rows Containing a String Pattern in Pandas Dataframe 101 🧐🔍
So you have a pandas dataframe and you want to filter the rows that contain a specific string pattern. No worries! In this guide, we'll walk you through the steps to achieve this effortlessly. Let's dive in! 💪
The Scenario 📚🔎
Assume we have the following dataframe in Pandas:
import pandas as pd
df = pd.DataFrame({'vals': [1, 2, 3, 4], 'ids': [u'aball', u'bball', u'cnut', u'fball']})
Which looks like:
ids vals
0 aball 1
1 bball 2
2 cnut 3
3 fball 4
Our goal is to filter the rows that contain the keyword "ball". So, the expected output would be:
ids vals
0 aball 1
1 bball 2
3 fball 4
The Solution 💡🔍
To achieve this, we can use the str.contains
method offered by Pandas. Here's a step-by-step guide on how to do it:
Import the required libraries:
import pandas as pd
Define your dataframe:
df = pd.DataFrame({'vals': [1, 2, 3, 4], 'ids': [u'aball', u'bball', u'cnut', u'fball']})
Use the
str.contains
method with the desired pattern:keyword = 'ball' filtered_df = df[df['ids'].str.contains(keyword)]
Voila! You have your filtered dataframe ready. You can inspect the result using:
print(filtered_df)
And there you have it! You've successfully filtered the rows containing the string pattern "ball" from your dataframe. 🎉
Possible Pitfalls and Tips ⚠️💡
Make sure you correctly pass the name of the column you want to filter to the
str.contains
method. In our case, it's'ids'
.Remember that the
str.contains
method is case-sensitive by default. If you want a case-insensitive match, you can set thecase
parameter toFalse
.Feel free to experiment with different string patterns using regular expressions to perform more advanced filtering.
The Call-to-Action 📢🤝
Now that you know how to filter rows containing a string pattern in a Pandas dataframe, you can unleash your data manipulation powers! Go ahead, give it a try with your own datasets and see how it simplifies your analysis and decision-making processes. If you found this guide helpful, share it with your fellow data enthusiasts! 🚀
Let us know in the comments below if you have any questions or if there are any other data topics you'd like us to cover. Keep exploring and happy coding! 🙌💻