How to extract numbers from a string in Python?
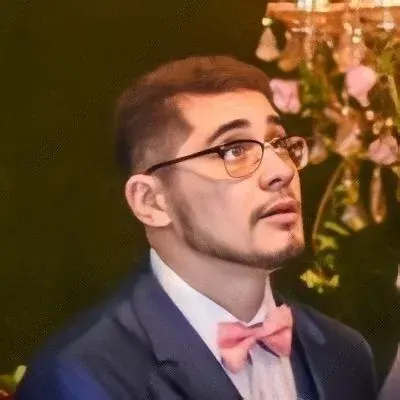
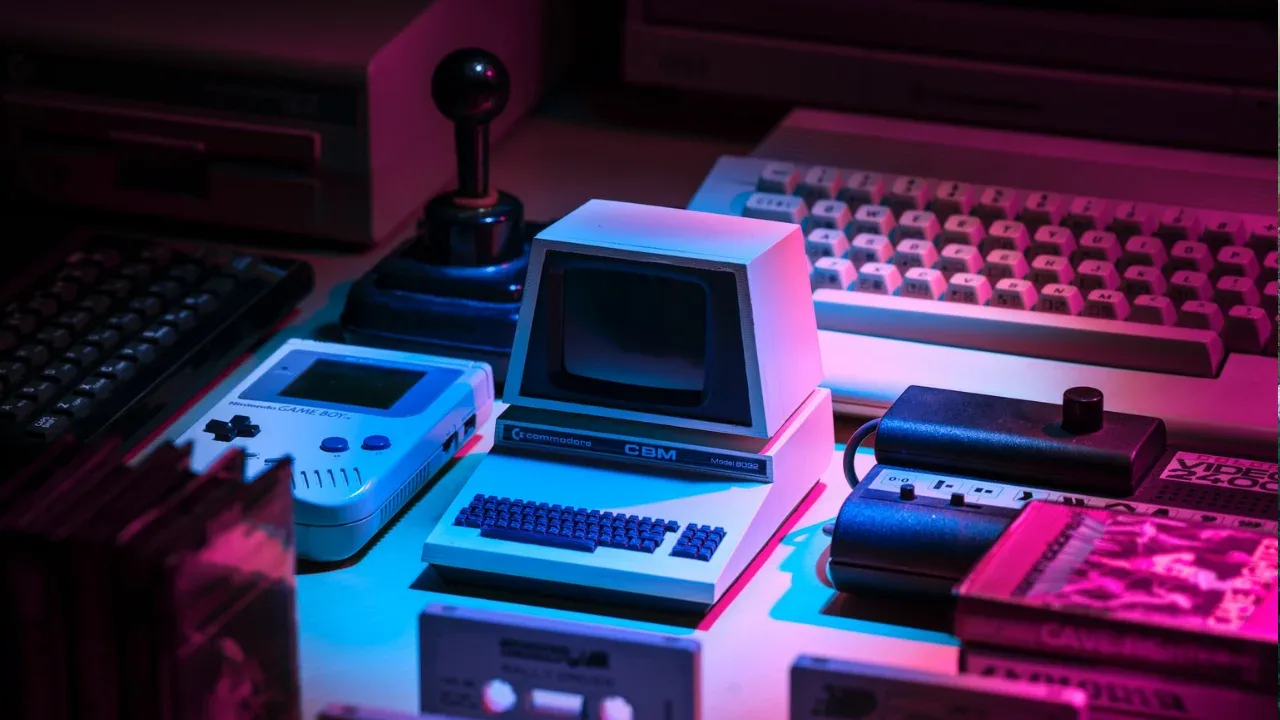
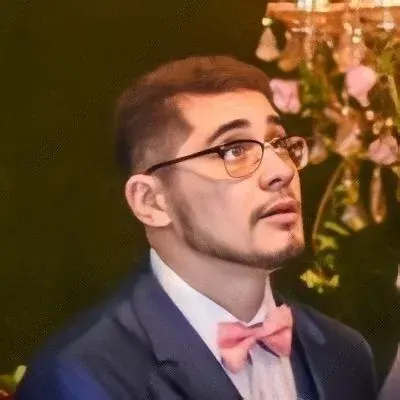
How to extract numbers from a string in Python? ππ’π»
Have you ever encountered a situation where you needed to extract all the numbers contained within a string in Python? π€ Whether you're dealing with user input, parsing text files, or just trying to extract numerical data from a string, this common problem has a couple of straightforward solutions. In this blog post, we'll explore two popular methods: using regular expressions and the isdigit()
method. Let's dive in! πͺ
Using Regular Expressions π₯π
Regular expressions (or regex) are powerful tools used for pattern matching in strings. They are especially handy when dealing with complex text patterns and extracting specific data. To extract numbers from a string using regex, we'll use the re
library available in Python. πͺπ§΅
Here's how you can do it:
import re
text = "hello 12 hi 89"
numbers = re.findall(r'\d+', text)
print(numbers)
Output:
['12', '89']
In the example above, re.findall(r'\d+', text)
searches for all occurrences of one or more digits (\d+
) within the text
string. The findall
function returns a list of all matching numbers.
Regular expressions provide flexibility in handling more complex cases. For instance, if you wanted to extract decimal numbers or negative numbers, you can modify the regex pattern accordingly. It's a powerful technique worth exploring further if your needs go beyond simple number extraction. πβ¨
Using the isdigit()
Method π‘π€
If the string you're working with only contains numeric digits, you can use the isdigit()
method available for strings. This method returns True
if all characters in the string are digits, and False
otherwise. However, it won't work if the string contains non-digit characters, such as spaces or punctuation marks. π«π’
To extract numbers using the isdigit()
method, you can split the string into individual words and check if each word is a number. Here's an example:
text = "hello 12 hi 89"
numbers = [int(word) for word in text.split() if word.isdigit()]
print(numbers)
Output:
[12, 89]
In this example, we split the text
string into individual words using the split()
method. Then, we iterate over these words and use the isdigit()
method to check if each word is a number. If it is, we convert it to an integer using int(word)
and add it to the numbers
list.
This method can be useful when you have control over the input string and are confident that it will only contain numeric values or words separated by spaces.
Choosing the Right Approach π€π
Now that you've seen both methods in action, you might be wondering which one is better suited for your needs. The choice between regex and the isdigit()
method depends on the complexity of the string you're working with:
If your string can contain non-digit characters and you need more precise control over the number extraction process (e.g., handling decimal places or negative numbers), regex provides a more powerful and flexible solution.
If your string only contains digits or words separated by spaces, and you prefer a simpler approach, the
isdigit()
method can be a convenient choice.
Consider the context and requirements of your specific use case to make an informed decision. π§
Wrapping Up and Taking Action ππ
Extracting numbers from a string in Python doesn't have to be a daunting task. By using either regular expressions or the isdigit()
method, you can easily extract numerical data without breaking a sweat. Choose the approach that best suits your needs, and remember to experiment and explore further depending on the complexity of your string patterns. π‘π’
Now itβs your turn! Have you encountered any challenges when trying to extract numbers from a string? What approach did you use, and how did it work for your specific use case? Share your thoughts, experiences, and questions in the comments below. Let's learn from each other! ππ¬
Happy coding! π»π