How to dynamically build a JSON object?
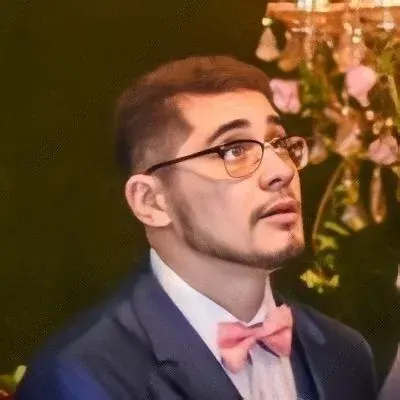
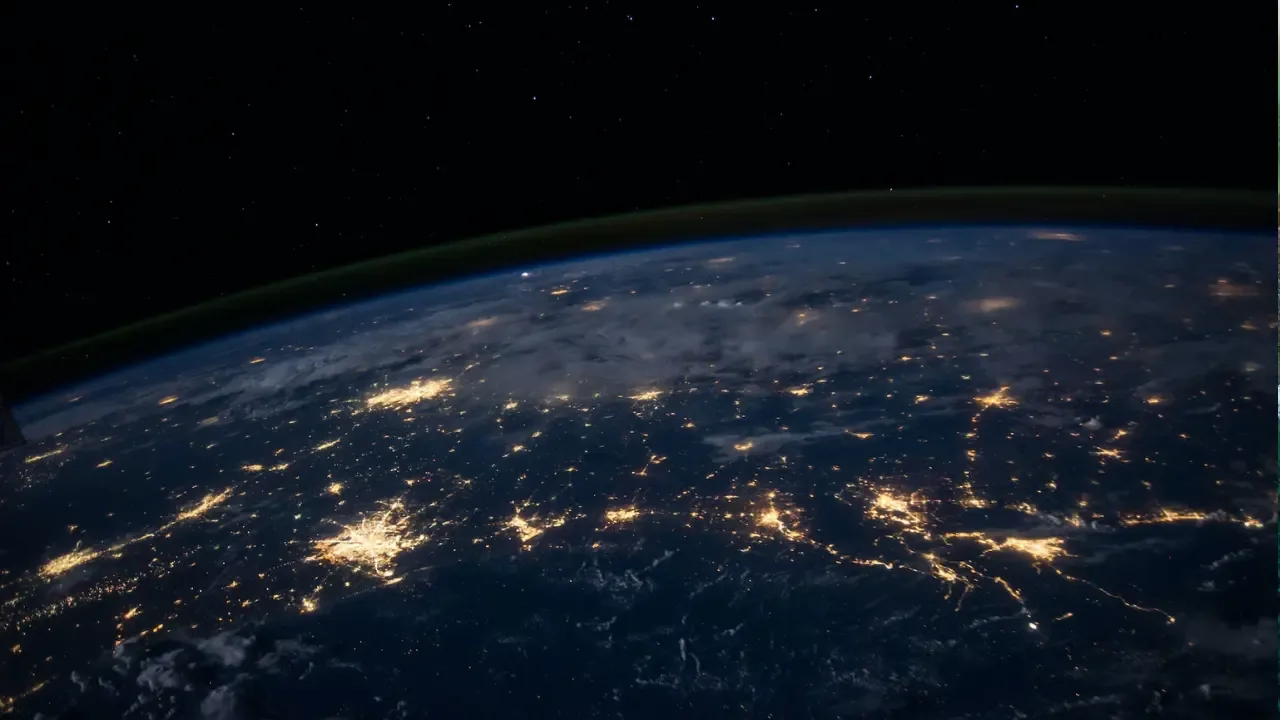
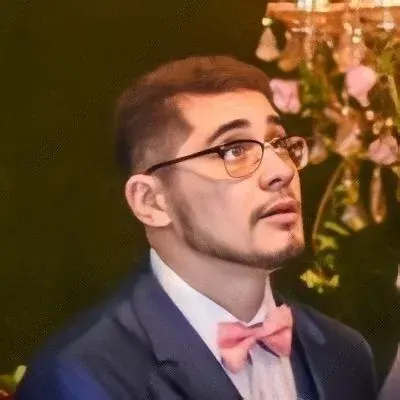
How to Dynamically Build a JSON Object in Python
So, you're new to Python and playing around with JSON data. You want to dynamically build a JSON object by adding key-value pairs to an existing object. Don't worry, we've got you covered!
Understanding the Problem
In the provided code snippet, you encountered a TypeError
saying "'str' object does not support item assignment". 🤔 This error occurs because the json_data
variable is a string, and strings in Python are immutable, meaning you can't change them once they are created.
Simple Solution - Use Python Dictionaries
To dynamically build a JSON object, you should use Python dictionaries. JSON objects and dictionaries have a similar structure, making it easy to convert between them using the json
library. Here's how you can modify your code:
import json
json_data = {} # Create an empty dictionary instead of a string
json_data["key"] = "value" # Add key-value pairs using dictionary notation
json_string = json.dumps(json_data) # Convert the dictionary to a JSON string
print('JSON: ', json_string)
✨ Voila! Now you have a dynamically built JSON object without any errors.
Explanation
Let's go step by step to understand what's happening in the corrected code:
We create an empty dictionary
json_data
instead of an empty string.We add key-value pairs using the dictionary notation
json_data["key"] = "value"
. This is possible because dictionaries in Python allow mutable item assignment.We convert the dictionary to a JSON string using
json.dumps(json_data)
. This function from thejson
library converts Python objects to JSON strings.Finally, we print the JSON string.
Wrapping Up
Now you know how to dynamically build a JSON object in Python using dictionaries instead of strings. 🎉
Remember, the key to solving this problem was understanding the difference between immutable strings and mutable dictionaries in Python.
Feel free to explore more complex scenarios where you can build nested JSON objects by adding dictionaries as values to your main dictionary. 🚀
Do you have any other questions or topics you'd like us to cover? Leave a comment below and let's continue the conversation! 👇