How to dump a dict to a JSON file?
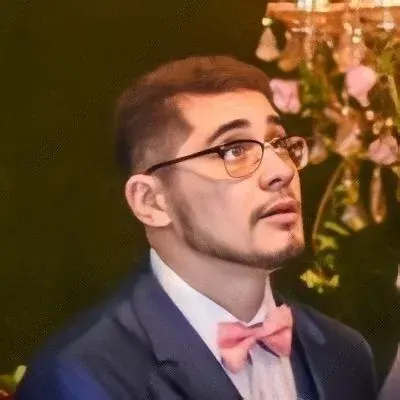
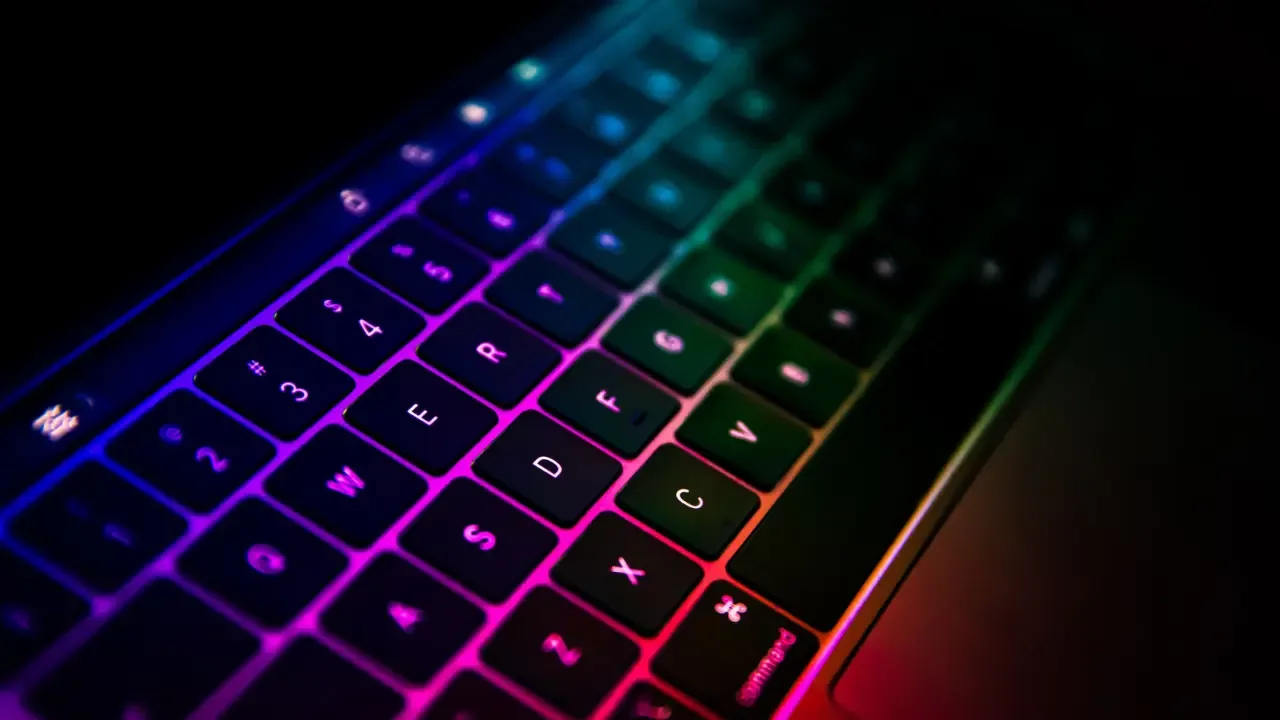
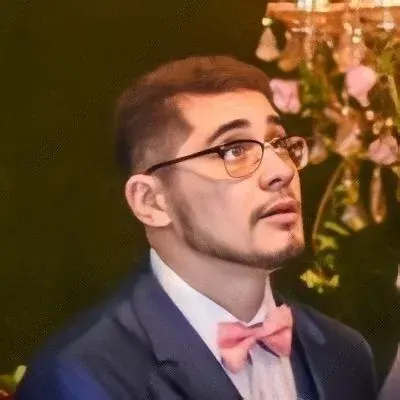
How to Dump a Dict to a JSON File: A Pythonic Way 🐍
So, you've got a dictionary that you want to dump into a JSON file in a specific format. Perhaps you want to generate a d3
treemap, and you need the data to be structured a certain way. Don't worry, we've got you covered! In this blog post, we'll walk you through the process of dumping your dictionary to a JSON file in a pythonic way. 🚀
Let's consider the following dictionary as an example:
sample = {'ObjectInterpolator': 1629, 'PointInterpolator': 1675, 'RectangleInterpolator': 2042}
And here's how you want it to look in the JSON file:
{
"name": "interpolator",
"children": [
{"name": "ObjectInterpolator", "size": 1629},
{"name": "PointInterpolator", "size": 1675},
{"name": "RectangleInterpolator", "size": 2042}
]
}
Sounds simple enough, right? Here's how you can achieve it: 😎
Step 1: Import the Required Libraries
To start, you'll need to import the json
library, which provides functions for working with JSON data:
import json
Step 2: Restructure the Dictionary
Next, we'll restructure the dictionary to match the desired JSON structure. In this case, we want to create a new dictionary with the keys "name"
and "children"
, and assign the appropriate values.
restructured_dict = {
"name": "interpolator",
"children": [
{"name": key, "size": value} for key, value in sample.items()
]
}
Here, we're using a list comprehension to iterate over the items in the sample
dictionary and create a list of dictionaries with the keys "name"
and "size"
.
Step 3: Dump the Dictionary to a JSON File
Finally, we'll dump the restructured_dict
to a JSON file using the json.dump()
function. This function takes two parameters: the dictionary to be dumped, and the file object to which the JSON data will be written.
with open("output.json", "w") as file:
json.dump(restructured_dict, file)
Here, we're using a context manager (with open(...) as file
) to automatically close the file after writing.
Step 4: Celebrate Your Success! 🥳
Congratulations! You've successfully dumped your dictionary to a JSON file in the desired format. Now you can use the generated JSON file for your d3
treemap or any other purpose you had in mind. 👏
Conclusion
Dumping a dictionary to a JSON file in a specific format is a common task, and luckily, Python makes it easy. By following the steps outlined in this guide, you can quickly restructure your dictionary and generate the desired JSON file with just a few lines of code. 🎉
If you found this guide helpful, make sure to share it with your friends and colleagues. And don't forget to let us know if you have any questions or suggestions for future blog posts. Happy coding! 😄💻
Related Links: