How to drop rows of Pandas DataFrame whose value in a certain column is NaN
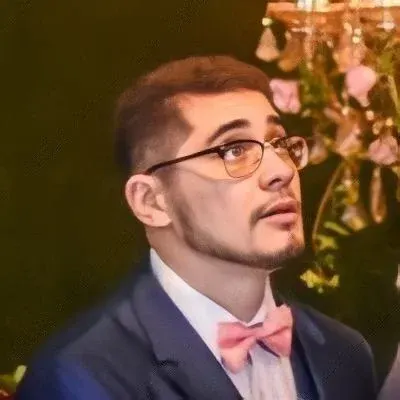
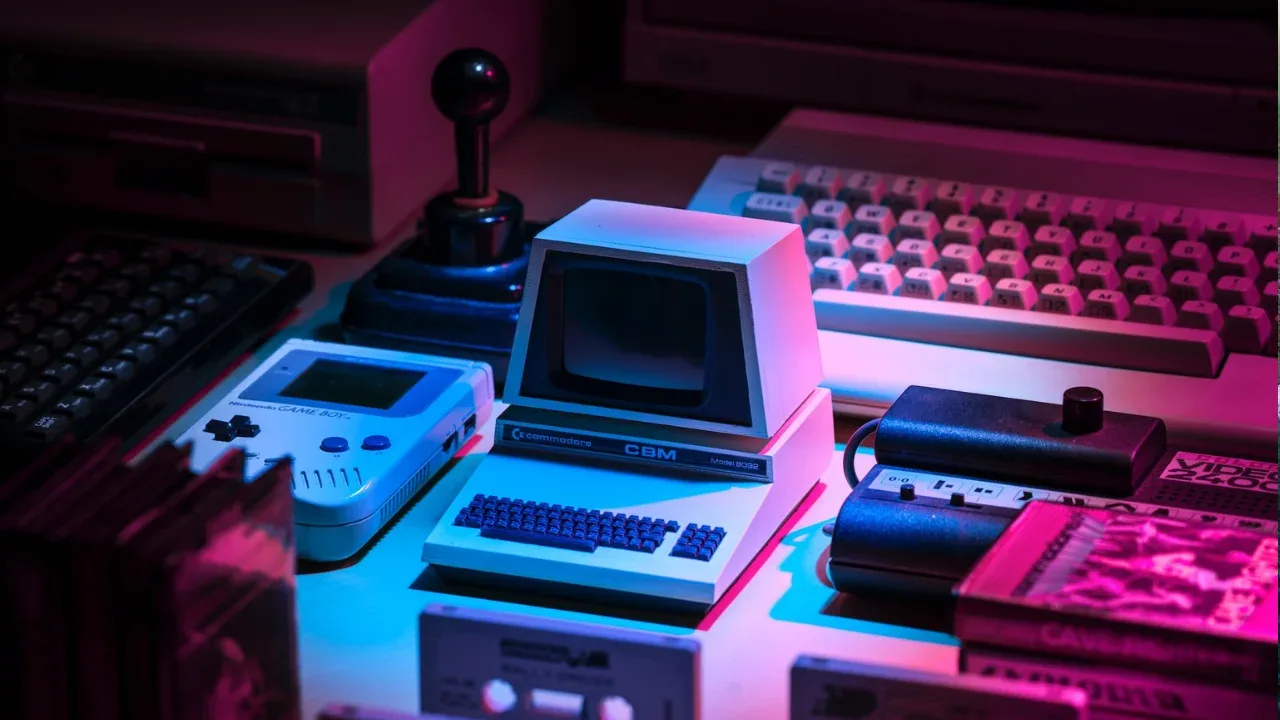
How to Drop Rows of Pandas DataFrame with NaN Values in a Certain Column
Are you struggling to drop rows from your Pandas DataFrame that have NaN values in a specific column? Don't worry, you're not alone! Many data analysts and scientists face this issue when working with messy data. In this guide, we'll walk you through some easy solutions to remove those pesky NaN rows and help you clean up your DataFrame.
Understanding the Problem
Let's begin by understanding the problem at hand. You have a DataFrame that looks something like this:
STK_ID EPS cash
STK_ID RPT_Date
601166 20111231 601166 NaN NaN
600036 20111231 600036 NaN 12
600016 20111231 600016 4.3 NaN
601009 20111231 601009 NaN NaN
601939 20111231 601939 2.5 NaN
000001 20111231 000001 NaN NaN
And you want to drop rows where the value in the EPS
column is NaN, resulting in the following DataFrame:
STK_ID EPS cash
STK_ID RPT_Date
600016 20111231 600016 4.3 NaN
601939 20111231 601939 2.5 NaN
Solution 1: Using the Pandas dropna
Method
Pandas provides a convenient method called dropna
that can be used to drop rows with NaN values. To achieve the desired result, you can use the following code:
df.dropna(subset=['EPS'], inplace=True)
Let's break down this code. The dropna
method is called on your DataFrame df
. The subset
parameter specifies the column(s) from which you want to drop the rows. In this case, we want to drop rows based on the EPS
column. The inplace=True
ensures that the changes are made directly on the original DataFrame, rather than creating a new one.
Solution 2: Using Boolean Indexing
Another approach to drop rows with NaN values in the EPS
column is by using boolean indexing. Here's an example of how you can achieve this:
df = df[df['EPS'].notna()]
In this code snippet, we're using the notna()
method to create a boolean mask. The mask checks each value in the EPS
column and returns True
for non-NaN values. By applying this mask to the DataFrame, we can filter out the rows with NaN values in the EPS
column.
Solution 3: Using the drop
Method
If you prefer using the drop
method, you can accomplish the same result by specifying the indices of rows where EPS
is NaN. Here's how you can do it:
df.drop(df[df['EPS'].isna()].index, inplace=True)
This code snippet finds the indices of rows where EPS
is NaN using the isna()
method, and then drops those rows using the drop
method with the inplace=True
parameter.
Conclusion and Call-to-Action
Congratulations! You now have three different ways to drop rows from a Pandas DataFrame based on NaN values in a specific column. Whether you choose to use the dropna
method, boolean indexing, or the drop
method, the decision depends on your personal preferences and coding style.
Take some time to experiment with these approaches and see which one works best for you. Remember to always take into account the size and complexity of your DataFrame when choosing the most appropriate solution.
If you found this guide helpful, be sure to share it with others who might be struggling with the same problem. And don't forget to subscribe to our newsletter for more tips and tricks on data analysis with Python! 📊🐼
Keep on coding! 💻🚀
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
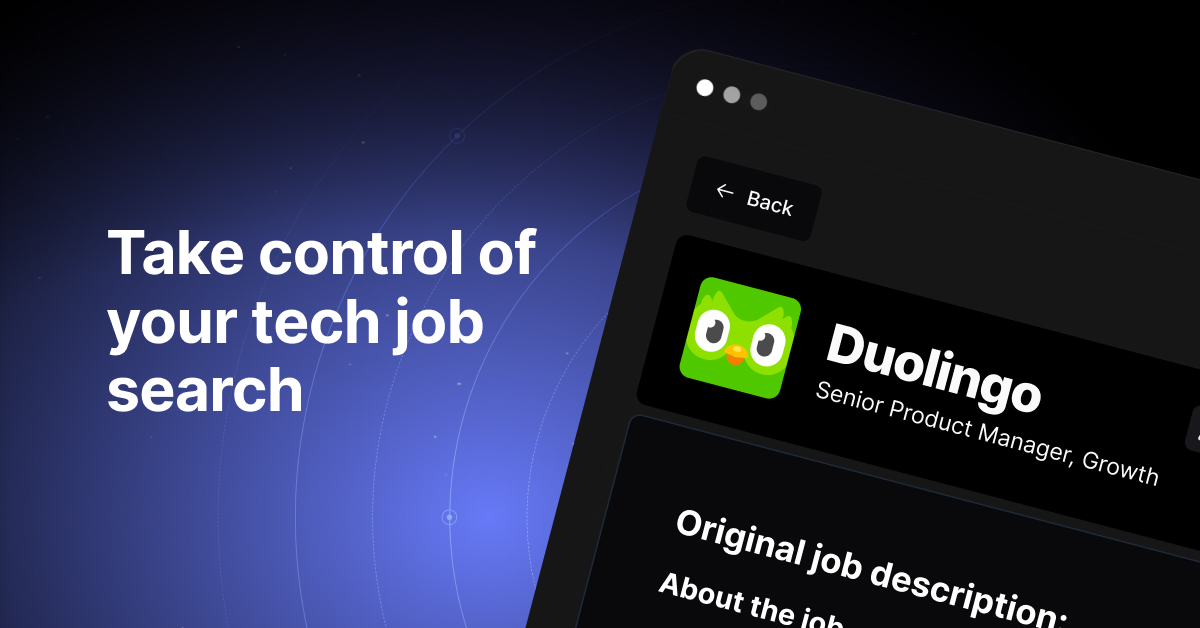