How to drop a list of rows from Pandas dataframe?
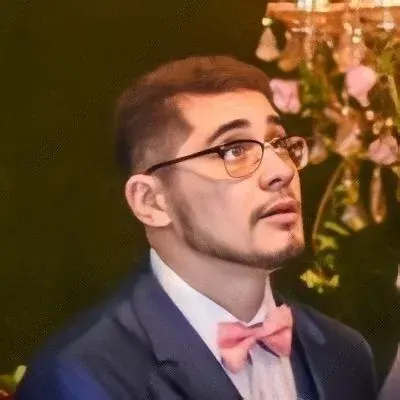
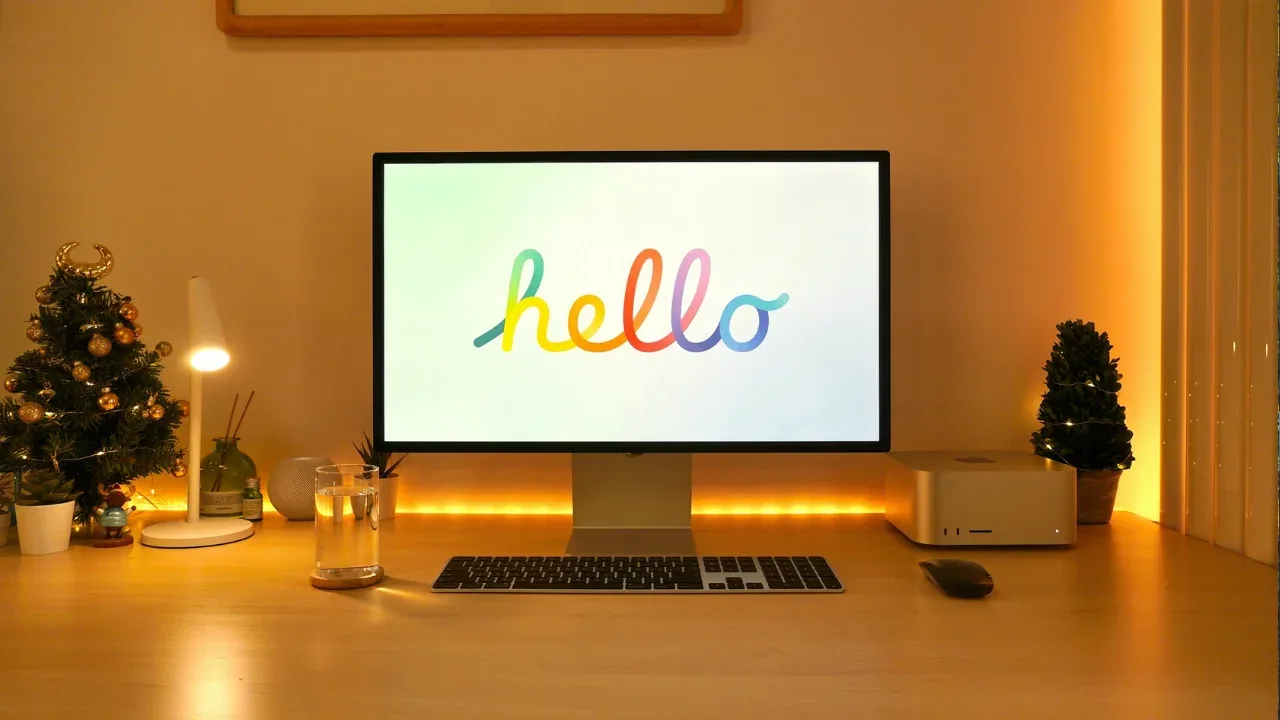
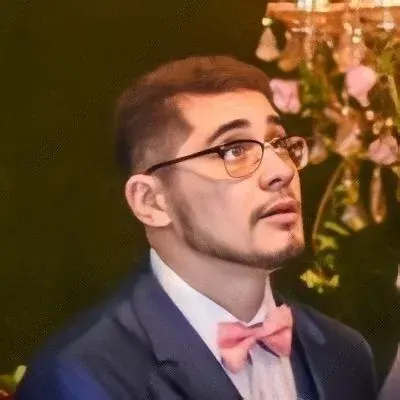
How to Drop a List of Rows from a Pandas DataFrame? πͺπ
If you are working with large datasets in Python using Pandas, you may find yourself needing to drop specific rows from a DataFrame. But what if you have a list of rows that you want to remove? In this blog post, we will explore different methods to drop a list of rows from a Pandas DataFrame in a simple and efficient way. Let's dive in! πββοΈ
The Problem: Dropping Specific Rows from a DataFrame π€
Consider the following DataFrame, called df
:
df = pd.DataFrame(data={'sales': [2.709, 6.59, 10.103, 15.915, 3.196, 7.907],
'discount': [np.nan, np.nan, np.nan, np.nan, np.nan, np.nan],
'net_sales': [2.709, 6.59, 10.103, 15.915, 3.196, 7.907],
'cogs': [2.245, 5.291, 7.981, 12.686, 2.71, 6.459]},
index=pd.MultiIndex.from_tuples([(600141, 20060331),
(600141, 20060630),
(600141, 20060930),
(600141, 20061231),
(600141, 20070331),
(600141, 20070630)],
names=['STK_ID', 'RPT_Date']))
This DataFrame represents sales data for a specific stock (STK_ID
) at different reporting dates (RPT_Date
). Now, you want to drop the rows that correspond to specific sequence numbers in a list, for example, [1, 2, 4]
. Your goal is to remove the second, third, and fifth rows, resulting in the following DataFrame:
df_dropped = pd.DataFrame(data={'sales': [2.709, 15.915, 7.907],
'discount': [np.nan, np.nan, np.nan],
'net_sales': [2.709, 15.915, 7.907],
'cogs': [2.245, 12.686, 6.459]},
index=pd.MultiIndex.from_tuples([(600141, 20060331),
(600141, 20061231),
(600141, 20070630)],
names=['STK_ID', 'RPT_Date']))
Now the question arises: How can we achieve this? Let's explore different solutions! π
Solution 1: Using the drop()
method with level
parameter βοΈ
A simple and elegant way to drop specific rows from a DataFrame is by using the drop()
method along with the level
parameter. The level
parameter allows us to specify the level of the MultiIndex we want to drop rows from. Here's how you can do it:
# Dropping rows using the 'drop()' method
rows_to_drop = [1, 2, 4]
df_dropped = df.drop(rows_to_drop, level=1)
In this example, we specified level=1
because we want to drop rows based on the second level of the MultiIndex, which represents the RPT_Date
. And voilΓ ! The df_dropped
DataFrame will contain only the desired rows. π
Solution 2: Using boolean indexing with isin()
function βοΈ
Another powerful approach to drop specific rows from a DataFrame is by using boolean indexing together with the isin()
function. The isin()
function enables us to check if values are contained in a list. Here's an example to illustrate this technique:
# Dropping rows using boolean indexing
rows_to_drop = [20060630, 20060930, 20070331]
df_dropped = df[~df.index.get_level_values('RPT_Date').isin(rows_to_drop)]
In this case, we used the isin()
function to check if each row's RPT_Date
is contained in the rows_to_drop
list. By negating the result with the ~
operator, we keep only the desired rows in the df_dropped
DataFrame. Cool, right? π
Solution 3: Using reset_index()
and isin()
together π
Alternatively, you can reset the index of your DataFrame using the reset_index()
method and then drop the rows using the isin()
function. Let's see how this can be done:
# Dropping rows using 'reset_index()' and 'isin()'
rows_to_drop = [20060630, 20060930, 20070331]
df_dropped = df[~df.reset_index()['RPT_Date'].isin(rows_to_drop)]
By resetting the index and then accessing the RPT_Date
column with reset_index()['RPT_Date']
, we can apply the isin()
function to check if the values are in the rows_to_drop
list. This method also provides us with the flexibility to combine it with other DataFrame operations if needed. Awesome! π
A Call to Action: Share Your Favorite Approach! π’
Now that you have learned various methods to drop a list of rows from a Pandas DataFrame, why not share your favorite approach with us? Let us know in the comments which solution you found most useful or if you have any other tips or tricks to tackle this problem. We love hearing from our readers! π¬π‘
Remember, manipulating datasets in Python is a superpower. So use these techniques wisely and keep exploring the endless possibilities of Pandas! Happy coding! πΌπ»