How to display pandas DataFrame of floats using a format string for columns?
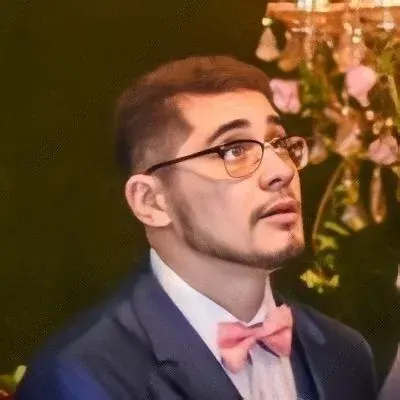
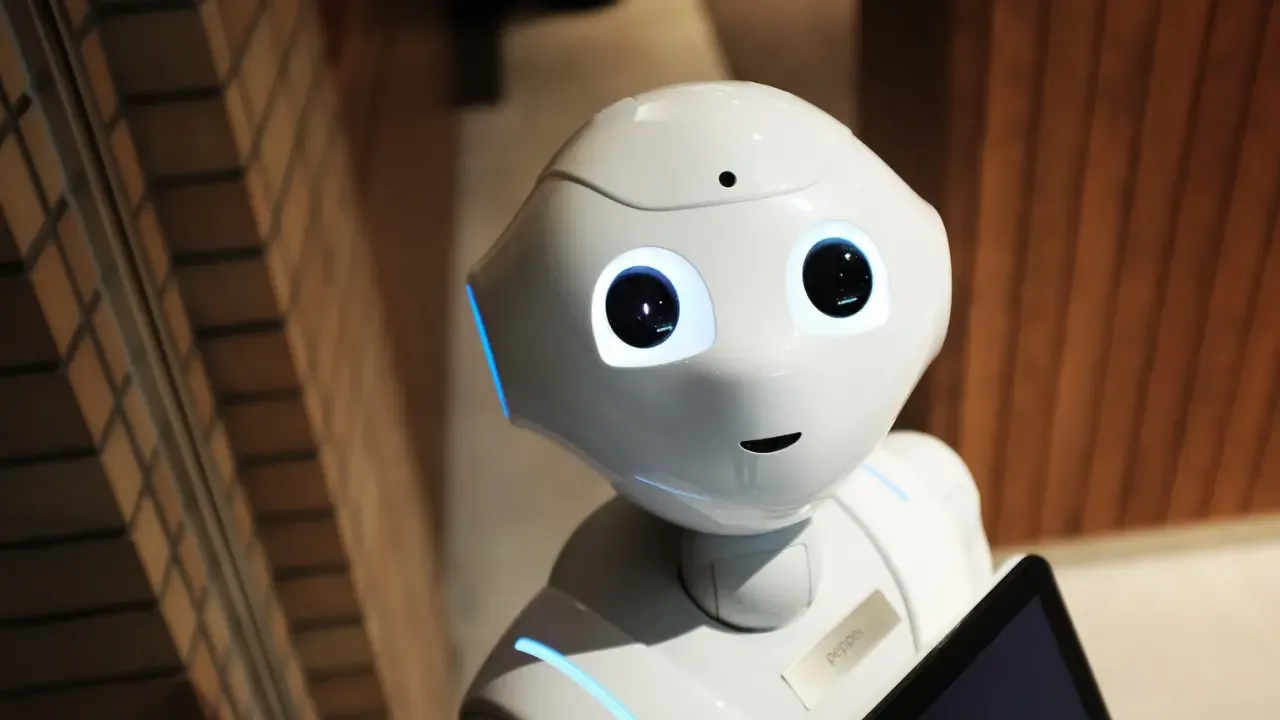
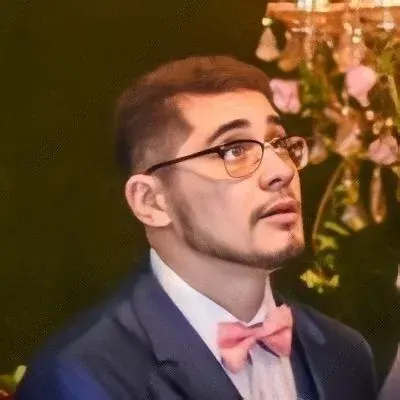
How to Display Pandas DataFrame of Floats Using a Format String for Columns? πΈ
So you want to display your pandas DataFrame with a specific format for the float columns? You're not alone! Many data analysts and scientists face this challenge when working with financial or numerical data. But fear not, because in this guide, I'll show you how to easily accomplish this task without modifying the data itself or creating a copy.
Let's start with the given example DataFrame:
import pandas as pd
df = pd.DataFrame([123.4567, 234.5678, 345.6789, 456.7890],
index=['foo', 'bar', 'baz', 'quux'],
columns=['cost'])
print(df)
This will display the DataFrame as follows:
cost
foo 123.4567
bar 234.5678
baz 345.6789
quux 456.7890
But what if you want to add a currency symbol and round the values to 2 decimal places π°? Let's dive into the solution!
Solution 1: Using DataFrame Styler π§ͺ
Pandas provides an amazing tool called DataFrame Styler, which allows you to apply various formatting options to your DataFrame for display purposes. Here's how you can use it:
styled_df = df.style.format({'cost': '${:.2f}'})
display(styled_df)
By applying the style.format()
method, we can specify a format string for the 'cost' column. In this case, '{:.2f}'
represents floating-point values rounded to 2 decimal places. The currency symbol '$' is added as well.
The display()
function from IPython is used to render the styled DataFrame in Jupyter notebooks or IPython environments. If you're running this code in a regular Python environment or script, you can use print()
to achieve a similar result.
Solution 2: Using Pandas Option π οΈ
Another approach is to modify the default options of pandas to format all float columns in a specific way. Here's how you can do it:
pd.options.display.float_format = '${:.2f}'.format
print(df)
By setting the pd.options.display.float_format
option, we can specify a format string that pandas will use to display float columns. In this case, '{:.2f}'
is used to round the values to 2 decimal places and add the currency symbol '$' as a prefix.
Keep in mind that modifying the default option affects all subsequent float values in all DataFrames. If you want to revert to the default format, you can reset it using the following code:
pd.options.display.float_format = None
Conclusion and Your Turn β¨
Congratulations! You've learned two easy ways to display pandas DataFrame of floats using a format string for columns. Whether you prefer using DataFrame Styler for more specific formatting or changing the default pandas option, both solutions allow you to achieve the desired result without altering the underlying data.
Now it's your turn to try it out! Apply these techniques to your own pandas DataFrames and impress your colleagues with beautifully formatted numerical results πͺ.
If you have any questions or run into any issues, feel free to ask in the comments below. Happy pandas styling! π©βπ»πΌπ₯