How to determine whether a Pandas Column contains a particular value
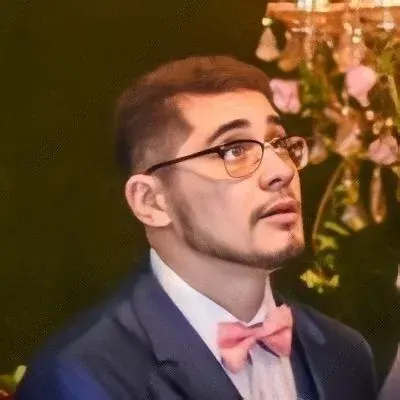
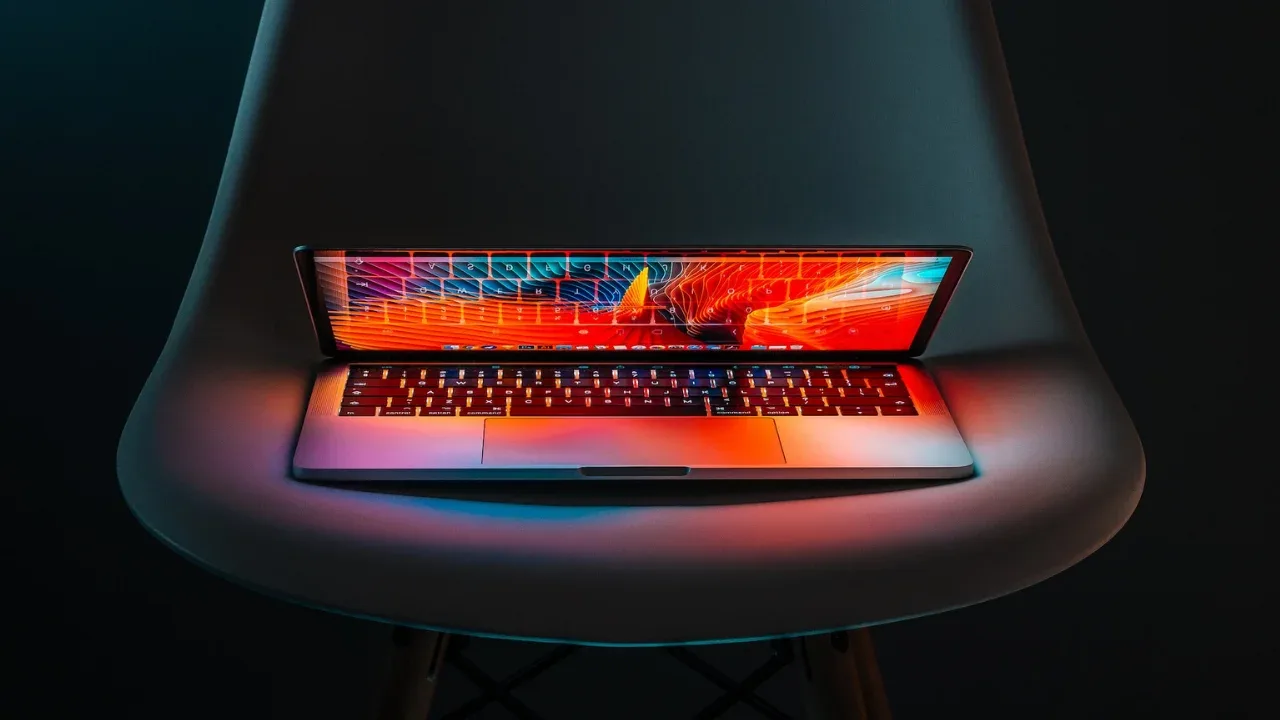
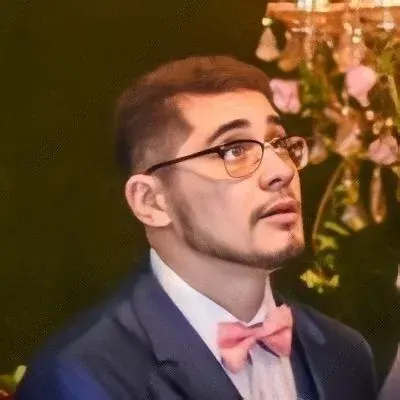
How to Determine Whether a Pandas Column Contains a Particular Value 😮🔍
Are you struggling to determine whether a value exists in a Pandas column? 😕 Don't worry, you're not alone! Many users face similar challenges when working with Pandas data frames. In this guide, we will address this common issue and provide easy solutions to help you find the answers you need. Let's dive right in! 💪💡
The Problem: 🤔
A user encountered an unexpected behavior when trying to determine if a Pandas column contained a particular value. They attempted to use the following code:
if x in df['id']:
# Some code logic...
However, even when they provided a value that they knew did not exist in the column (e.g., 43 in df['id']
), it still returned True
. This behavior raised questions, as they expected it to return False
since there were no matching entries when selecting a subset of the data frame using df[df['id'] == 43]
.
The Solution: ✨
To solve this problem, we need to understand how Pandas handles these operations. When you use if x in df['id']
, it checks if x
is present in the entire column. However, the returned result is a Boolean series with True
or False
values for each row, indicating whether a match was found.
To obtain a single Boolean value indicating whether a particular value exists in the column, we can leverage the .any()
method. Here's how you can modify the code to achieve the desired outcome:
if (df['id'] == x).any():
# Some code logic...
By using (df['id'] == x)
within parentheses, we create a Boolean series that compares each value in the column with x
. The .any()
method then checks if any of these comparisons result in True
, indicating that a match was found.
Example Usage: 💻🔬
Let's illustrate this with an example. Suppose we have a Pandas data frame called df
with the following 'id' column:
id
--
10
20
30
To check if x = 30
exists in the 'id' column, we can use the modified code:
if (df['id'] == 30).any():
print("Match found!")
else:
print("No match found.")
In this case, the code will output: "Match found!"
Why Doesn't the Original Method Work? 🤷♂️
The original approach using if x in df['id']
returns a Boolean series rather than a single Boolean value. This series compares x
with each row in the column, resulting in a series of True
and False
values. Therefore, by checking if this series exists, the condition if x in df['id']
will always return True
. It's important to note that this behavior is consistent with Pandas' design and allows for more flexible operations on data frames.
Conclusion: 🎉📝
Determining whether a particular value exists in a Pandas column is now a piece of cake for you! By using (df['id'] == x).any()
instead of if x in df['id']
, you can obtain accurate results and make informed decisions in your data analysis endeavors.
We hope this guide has shed light on this common problem and provided you with an accessible solution. Give it a try, and let us know your thoughts! If you have any other questions or face any challenges, feel free to reach out in the comments below. Happy Pandas coding! 😄🐼
** 🚀 Remember, with Pandas data frames, there's always a way to get the answers you seek! 🚀**