How to determine a Python variable"s type?
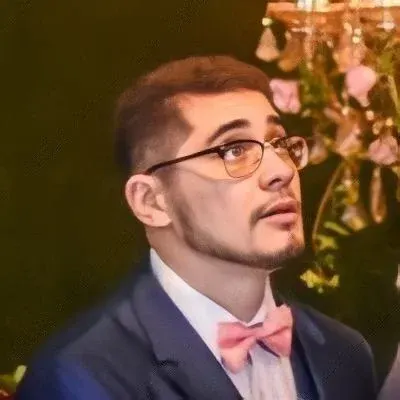
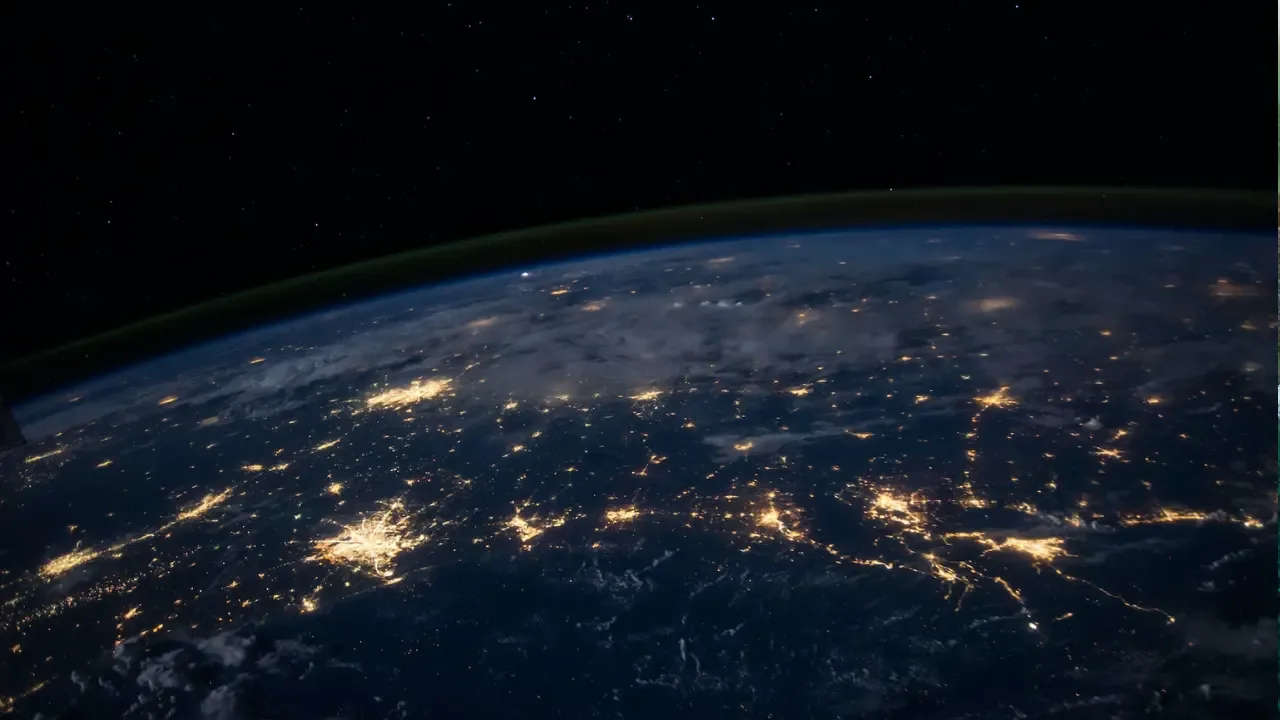
🐍 The Secret Life of Python Variables: Unraveling Their Mysteries 🕵️♀️
So, you want to know the true nature of Python variables, huh? You've come to the right place! 🎉
Picture this: You're immersed in your Python code, working your magic, when suddenly you find yourself at a crossroads. You stumble upon a variable, but have no clue what type it really is! 😱 Don't worry, my friend, we're about to shed some light on this matter.
🔍 Examining the True Identity of Python Variables
To determine the type of a variable, Python offers us a handy built-in function called type()
. Using this function is as easy as pie! 🍰 Just pass your variable as an argument, like so:
my_variable = 42
print(type(my_variable))
This will output the true identity of my_variable
, and you'll finally gain the insights you seek. 💡
🚀 Common Issues: Unsigned 32-Bit Variables
Ah, the fabled unsigned 32-bit variable! This is a common source of confusion for many Pythonistas. 🤔 But worry not, my friend, for I shall guide you through this.
Python doesn't have a built-in unsigned 32-bit integer data type. Instead, it provides a struct
module that you can use to represent unsigned integers of different sizes. Let's see how it works:
import struct
# Let's say you have an unsigned 32-bit integer represented as a byte string
byte_string = b'\x01\x00\x00\x00'
# Unpack the byte string using the 'I' format specifier
unsigned_int = struct.unpack('I', byte_string)[0]
print(unsigned_int)
And BOOM! 💥 There you have it! By using the struct
module, you can easily handle unsigned 32-bit integers in Python.
📣 Let's Engage!
Congratulations, my friend! You've reached the end of this exciting journey into the realm of Python variable types. 🎉 But wait, there's more! I encourage you to experiment with different variable types and share your discoveries in the comments below. 🗣️
Do you have any other Python conundrums or mind-boggling tech puzzles that need solving? Let us know, and we'll be sure to dive right in and provide you with the answers you seek. 🙌
Remember, in the world of programming, curiosity is key! So go forth, explore, and may the Pythonic forces be with you! 🐍💪
Happy coding! 😄✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
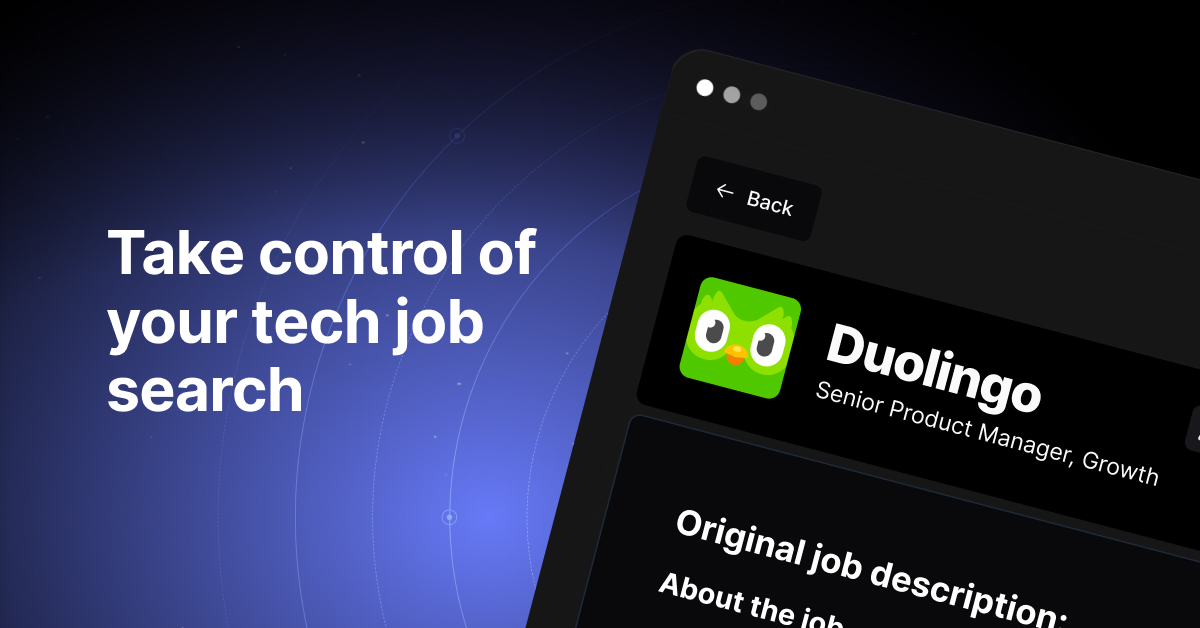