How to delete last item in list?
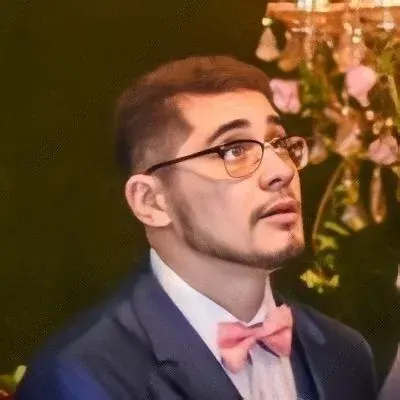
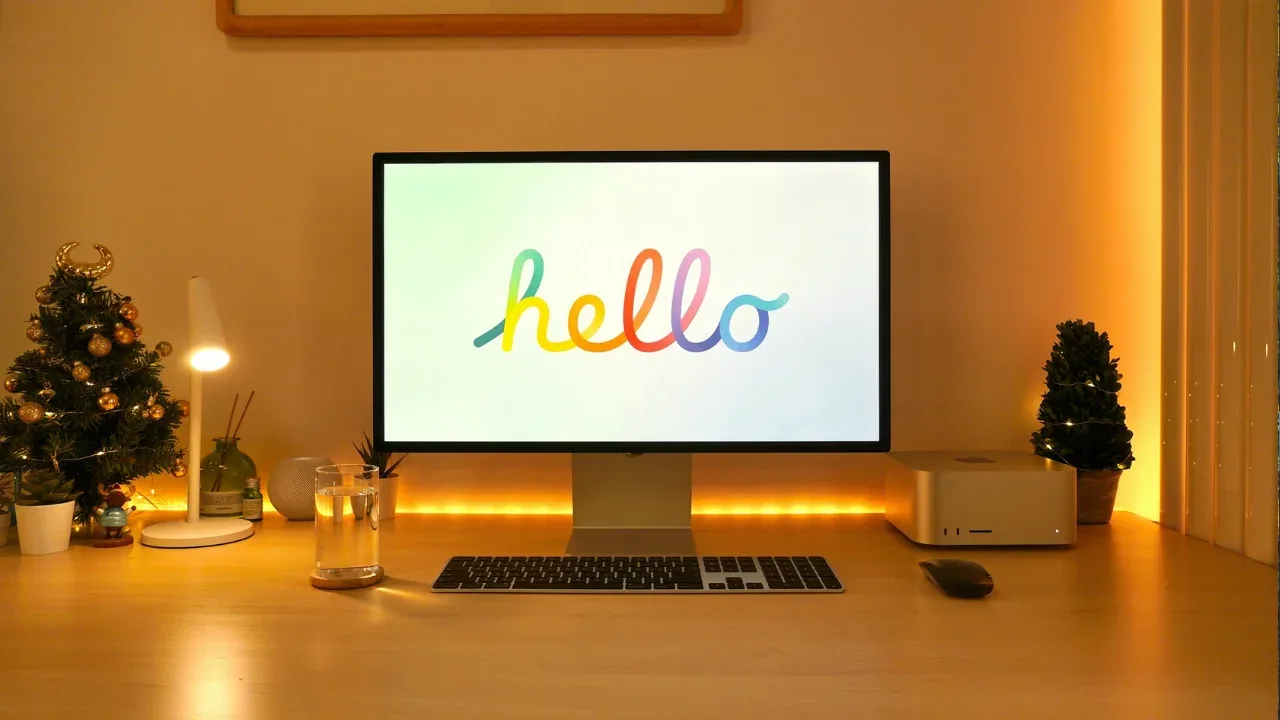
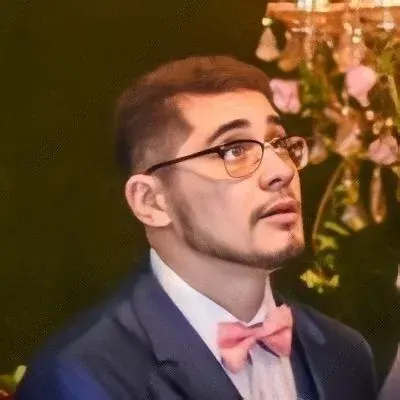
How to Delete the Last Item in a List 💥
Are you struggling to delete the last item in a list in Python? 😩 Don't worry, you're not alone! Many developers face this challenge when working with lists. In this blog post, we're going to tackle this problem head-on and provide you with easy solutions to delete the last item in a list. Let's dive in! 🚀
Understanding the Problem 🤔
To give you some context, let's consider an example. Imagine you have a program that calculates the time it takes for someone to answer a specific question. You want to quit the program's while loop if the answer is incorrect. However, you also need to delete the last calculation from the list so that it doesn't affect the overall result. Sounds tricky, right? 😓
Here's a snippet of the code that you might be working with:
from time import time
q = input('What do you want to type? ')
a = ' '
record = []
while a != '':
start = time()
a = input('Type: ')
end = time()
v = end-start
record.append(v)
if a == q:
print('Time taken to type name: {:.2f}'.format(v))
else:
break
for i in record:
print('{:.2f} seconds.'.format(i))
Solution 1: Using the pop()
Method 🗑️
One simple solution to delete the last item in a list is by using the pop()
method. By default, pop()
removes the last item in the list and returns the value. Here's how you can apply this to your code:
record.pop()
In your case, you can place this line of code after the record.append(v)
line. By doing so, the last item will be removed from the list, and you can call min()
without it affecting the wrong time calculation.
Solution 2: Slicing the List 🍰
Another approach to deleting the last item in a list is by utilizing slicing. With slicing, you can extract a portion of a list, excluding the last item. Here's how to implement this solution:
record = record[:-1]
This line of code will create a new list record
that contains all the elements except for the last one. By assigning this new list to the variable record
, you effectively remove the last item from the original list.
Solution 3: Using the del
Statement 🗑️
If you prefer a bit more simplicity, you can use the del
statement to delete the last item in a list. Here's an example:
del record[-1]
The del
statement allows you to remove an item at a specific index in the list. In this case, -1
refers to the last item in the list.
Conclusion and Getting Engaged ✨
Deleting the last item in a list can be a little tricky, but with these solutions, you should be able to master it in no time! 💪
Now it's your turn to take action! Try implementing one of these solutions in your code and see the magic happen. Don't worry if you encounter any issues – we're here to help! If you have any questions or need further assistance, feel free to leave a comment below. Let's tackle this challenge together! 🤝
Happy coding! 😄👩💻👨💻