How to delete a record in Django models?
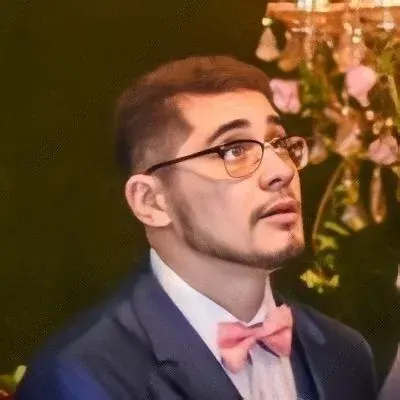
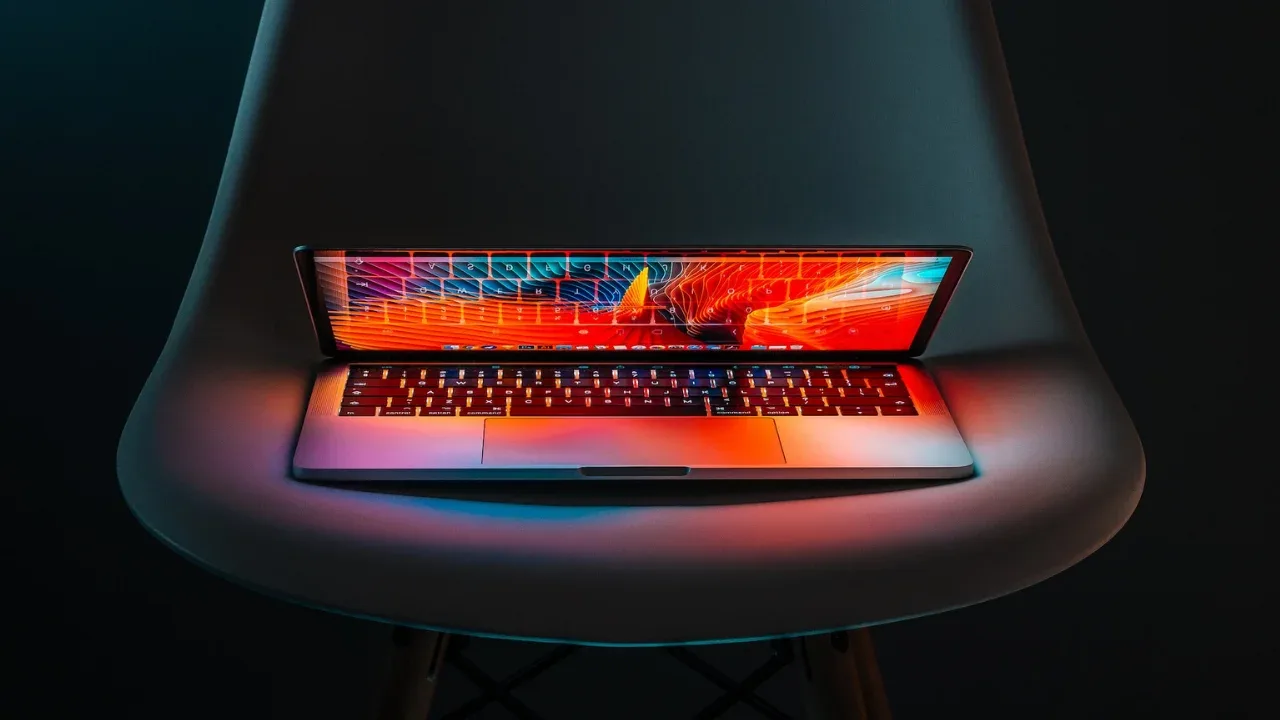
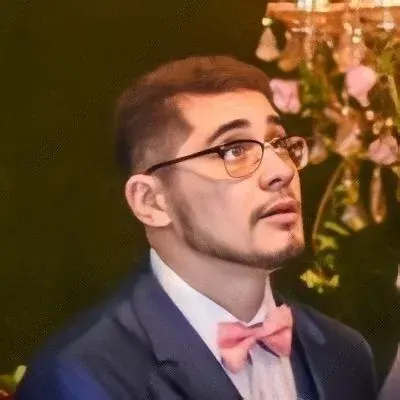
🚀 How to Delete a Record in Django Models?
Deleting a record in Django models might seem like a daunting task, but fear not! In this guide, we will walk you through the process step by step and provide easy solutions to common issues. By the end, you'll be able to confidently delete records in Django models like a pro!
📝 Understanding the Problem
To delete a record in Django models, we need to emulate the SQL query DELETE FROM table_name WHERE id = 1;
. In Django, we interact with the database using objects called models. Each model represents a database table, and each instance of a model represents a specific row in that table.
Given the context you provided, we assume you have a table named table_name
and you want to delete the record with id = 1
. Let's dive into the solutions!
🛠️ The Solutions
Solution 1: Using the .delete()
method
The simplest and most straightforward way to delete a record in Django models is by using the .delete()
method. Here's how you do it:
Record.objects.get(id=1).delete()
In this example, Record
is the Django model representing the table_name
table. We use the .get(id=1)
method to retrieve the record with id = 1
, and then we call .delete()
on it to remove it from the database.
If the record with the specified id
does not exist, this solution will raise a Record.DoesNotExist
exception. To handle this, you can wrap the delete operation in a try-except
block.
Solution 2: Using the .filter()
method
Another approach is to use the .filter()
method to find the record based on a specific condition and then delete it. Here's an example:
Record.objects.filter(id=1).delete()
In this case, the .filter(id=1)
method returns a queryset that matches the condition id = 1
. We then call the .delete()
method on the queryset to delete all matching records.
If no records match the condition, this solution won't raise any exceptions and will simply return without deleting anything.
Solution 3: Deleting multiple records
To delete multiple records at once, you can use the .filter()
method with additional conditions. For example, to delete all records with id
less than 5:
Record.objects.filter(id__lt=5).delete()
In this case, the id__lt=5
condition selects all records with an id
less than 5. By calling .delete()
on the queryset, you delete all those matching records in one go!
📣 Time to Take Action!
Now that you know how to delete records in Django models, it's time to put this knowledge into action! Experiment with different models, conditions, and deletion scenarios to strengthen your understanding.
If you have any questions or run into any issues, feel free to reach out to us in the comments section below. We're here to help! 😊
Happy deleting! 💥