How to declare and add items to an array in Python
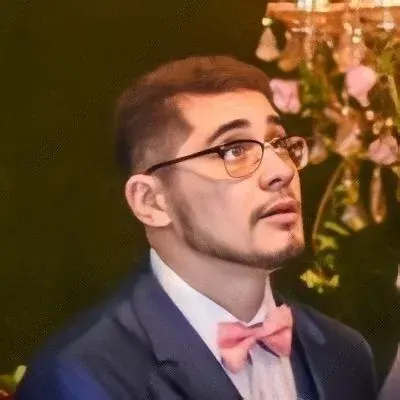
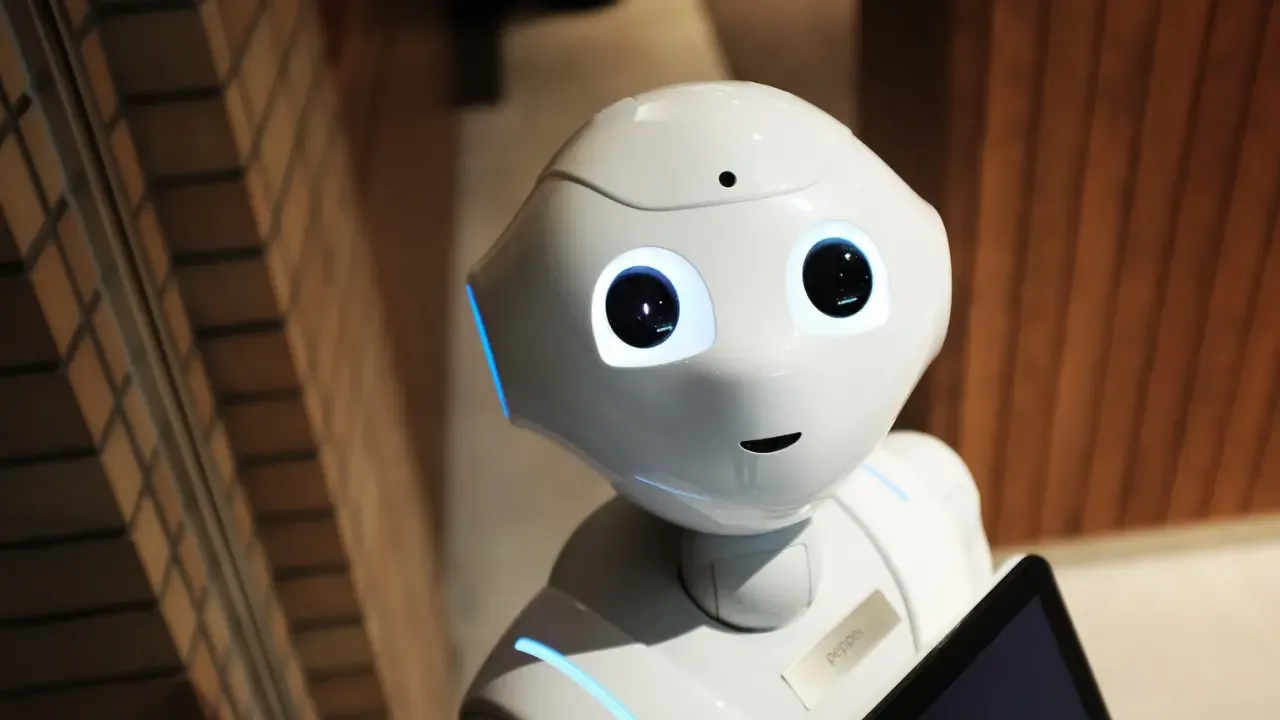
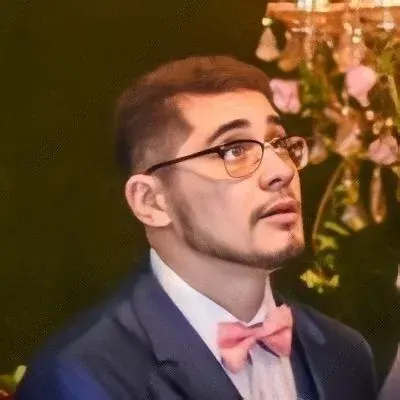
How to Declare and Add Items to an Array in Python
So, you want to add items to an array in Python? 🐍 Great! Arrays are a fundamental data structure that allow you to store multiple values in a single variable. However, the syntax for declaring and adding items to an array in Python may differ slightly from other languages you may be familiar with.
The Problem
Let's start by addressing the specific problem mentioned in the context. The code provided suggests that the user is trying to declare an empty array and then add items to it using the append()
method. However, they encounter an error because dictionaries, denoted by {}
, are being used instead of arrays.
The Solution
To solve this problem, you need to declare an array, not a dictionary. In Python, arrays are typically created using the list
keyword or by enclosing the values in square brackets []
. Here's an example of how you can declare an empty array and add items to it:
my_array = [] # Declare an empty array
my_array.append("value1") # Add items to the array
my_array.append("value2")
my_array.append("value3")
In the code snippet above, we first declare an empty array called my_array
using the square bracket notation []
. Then, the append()
method is used to add items to the array by specifying the values in double quotes within the parentheses. Each call to append()
adds a new item to the end of the array.
Alternatively, you can also declare an array with initial values using the following syntax:
my_array = ["value1", "value2", "value3"]
This creates an array my_array
with three initial values: "value1"
, "value2"
, and "value3"
. Adding new items to this array would follow the same append()
method as shown before.
Common Issues
1️⃣ Forgetting to Declare an Array
One common issue is forgetting to declare an array before trying to add items to it. Remember to use either the list
keyword or the square bracket notation to declare your array.
2️⃣ Using an Incorrect Method
As seen in the initial problem, using an incorrect method like append()
on a dictionary instead of an array can result in an error. Make sure to use the correct method for arrays.
3️⃣ Overwriting the Array
Another mistake is overwriting the array with a new value instead of adding items to it. If you reassign a new value to an existing array variable, its previous contents will be lost.
Take It Further!
Now that you know how to declare and add items to an array in Python, the possibilities are endless! You can access and manipulate array elements using their index values, use loops to iterate through arrays, or even combine arrays to perform more complex calculations. Keep exploring and experimenting with arrays to unleash the full power of Python!
If you have any questions or run into any issues, feel free to comment below. Happy coding! 😊