How to debug in Django, the good way?
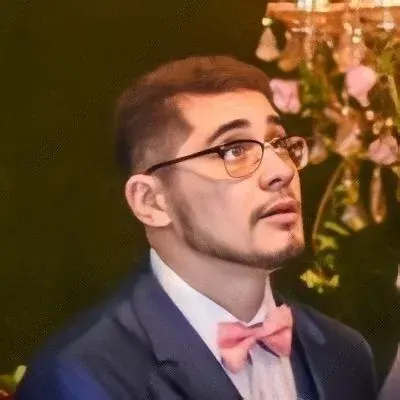
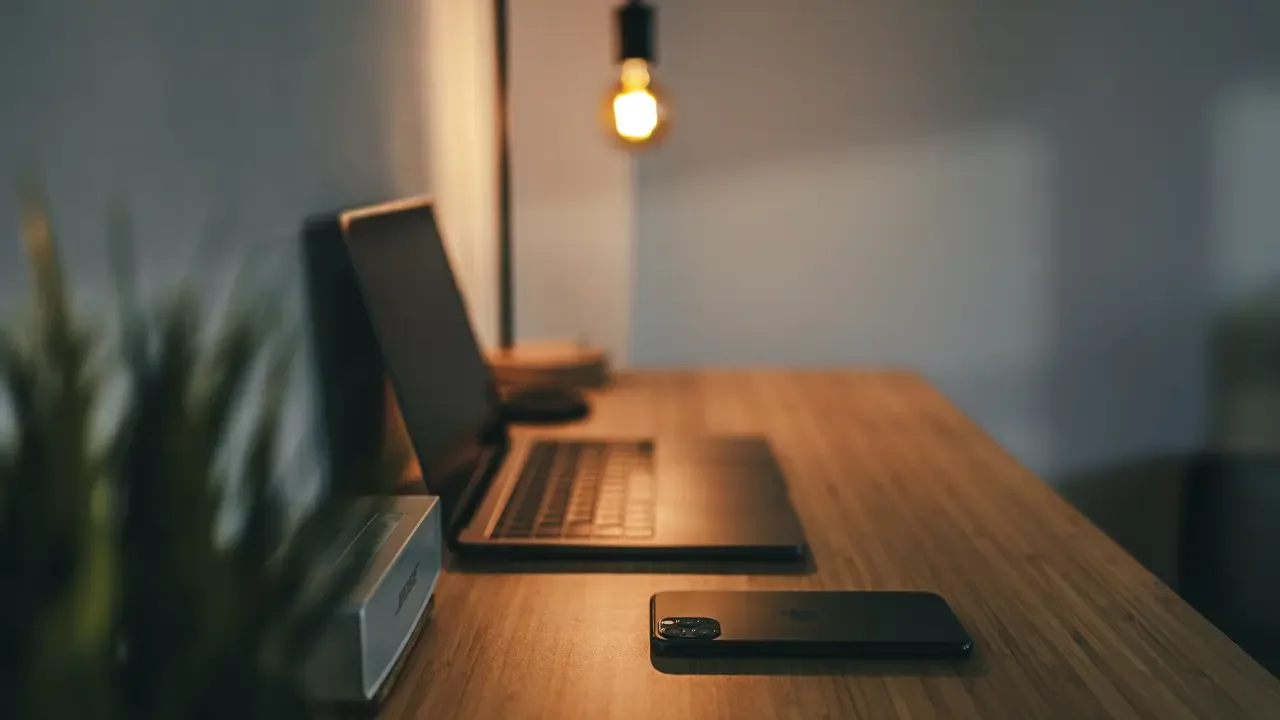
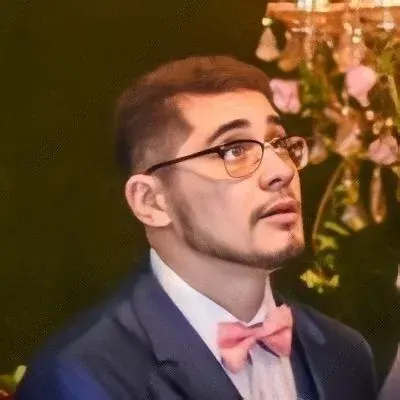
🔎 How to Debug in Django, the Good Way? 🔍
If you're new to Python and Django, you've probably encountered the daunting task of debugging your code. It's easy to get lost in the sea of tracebacks and syntax errors, but fear not! I've got some tips and tricks to help you debug like a pro. Let's dive in! 💻
Understanding the Debugging Process
First things first, let's understand the basic debugging process. When you encounter an error in your Django code, Django's debug mode will provide you with a detailed traceback. This traceback contains valuable information about the error, including the line number and file where it occurred.
To get started with debugging, you can simply examine the traceback and identify the line where the error occurred. However, there are better ways to streamline this process and make it more efficient.
1. Make Use of the print()
Statement 🖨️
One of the simplest yet effective ways to debug your Django code is by strategically placing print()
statements in your code. By printing important variables and values, you can gain insights into how your code is executing and identify any unexpected behaviors.
For example, if you suspect that a certain variable is not getting the value you expect, you can print its value at specific points in your code. This will help you pinpoint where the issue lies and compare the actual value to your expected one.
def my_view(request):
my_variable = get_value()
print(my_variable) # Debugging statement
# Rest of the code
2. Take Advantage of the Django Debug Toolbar 🛠️
The Django Debug Toolbar is a fantastic tool that provides a comprehensive debugging interface for your Django projects. It gives you detailed information about the queries executed, cache usage, and much more.
To install the Django Debug Toolbar, simply add it to your project's settings.py
file:
INSTALLED_APPS = [
...
'debug_toolbar',
...
]
Once installed, you can access the debug toolbar by adding ?debug_toolbar
to the end of your URL. This will open a dedicated panel that displays various debugging information, making it easier to identify and resolve issues.
3. Utilize Breakpoints with Django's Built-in Debugger 🕵️♀️
Django comes with a built-in debugger called pdb (Python Debugger), which allows you to set breakpoints in your code. Breakpoints pause the execution of your code at specific points, allowing you to inspect variables and step through your code line by line.
To use breakpoints, you need to import the pdb
module and call the set_trace()
function at the desired location:
import pdb
def my_view(request):
my_variable = get_value()
pdb.set_trace() # Breakpoint
# Rest of the code
When you run your Django application and reach the breakpoint, the execution will pause, and you can interactively debug your code. You can print variables, step through the code, and explore the state of your application.
4. Leverage Logging for Debugging Information 📝
Logging is another powerful tool that can assist you during the debugging process. By strategically placing log statements in your code, you can output valuable information about the flow of your application.
To use logging in Django, you need to import the logging
module and configure it in your project's settings.py
file:
import logging
logging.basicConfig(level=logging.DEBUG)
Once configured, you can use various logging levels (e.g., DEBUG, INFO, WARNING) to output different levels of information. For example:
import logging
logger = logging.getLogger(__name__)
def my_view(request):
my_variable = get_value()
logger.debug(f"Value of my_variable: {my_variable}")
# Rest of the code
By exploring the log output, you can gain helpful insights into the execution flow and identify any anomalies or unexpected behavior.
Conclusion and Call-to-Action
Debugging in Django doesn't have to be a frustrating experience. By following these tips and utilizing various debugging techniques, you can streamline the process and uncover hidden bugs with ease.
So, the next time you find yourself scratching your head over a Django error, remember to:
🖨️ Use the
print()
statement strategically🛠️ Take advantage of the Django Debug Toolbar
🕵️♀️ Utilize breakpoints with Django's built-in debugger
📝 Leverage logging for debugging information
Start debugging like a pro today and conquer those bugs! 🚀
Have you encountered any interesting debugging scenarios in Django? Share your experiences and tips in the comments below. Let's debug together and make coding easier for everyone! 💪