How to deal with SettingWithCopyWarning in Pandas
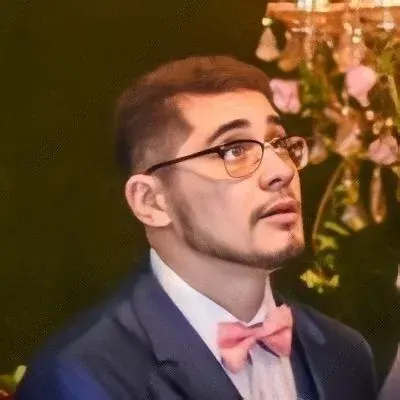
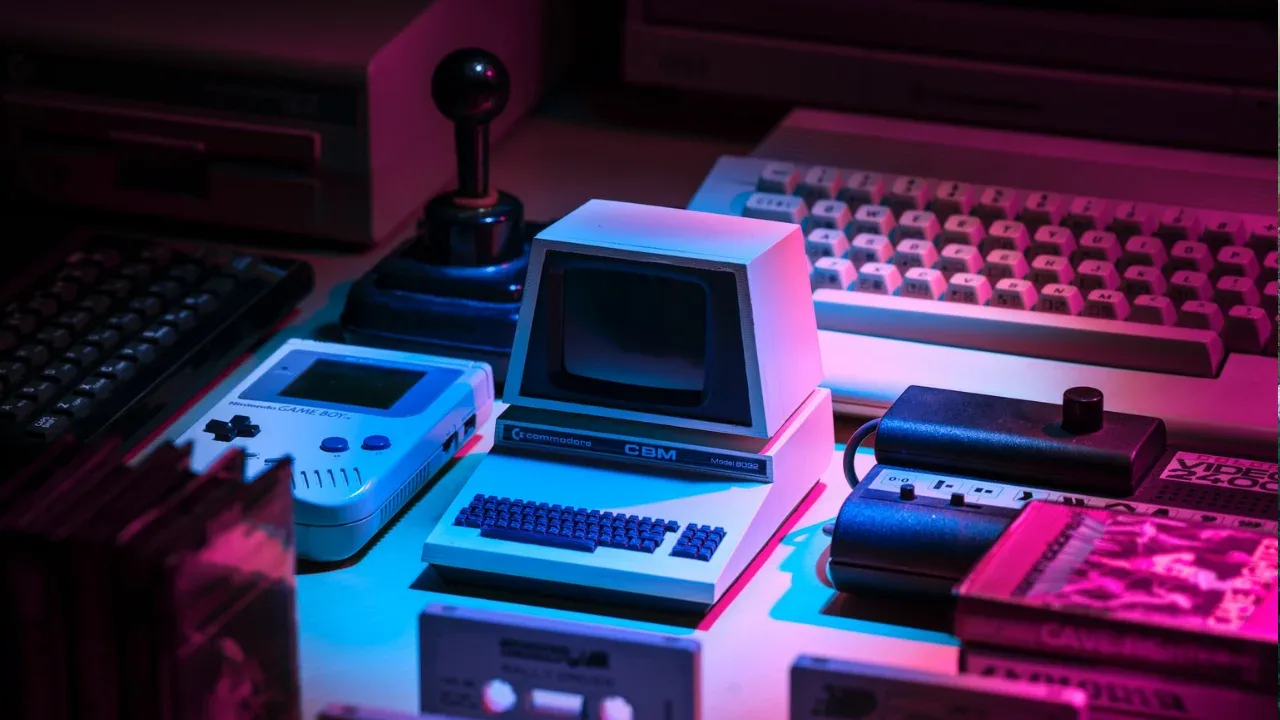
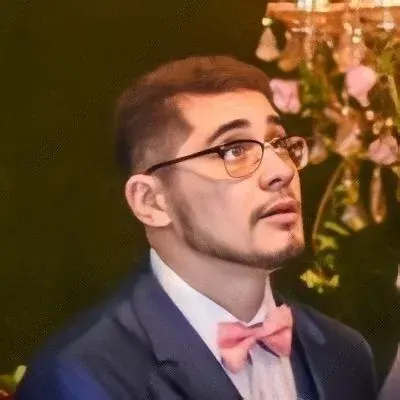
How to Deal with SettingWithCopyWarning in Pandas 👀💥
So, you've just upgraded your Pandas library and now your application is throwing out a bunch of new warnings. One of them is the dreaded SettingWithCopyWarning
. What does it mean? Do you need to make changes to your code? And how can you suspend the warning if you still want to use the offending code?
In this blog post, we'll dive into the world of SettingWithCopyWarning
in Pandas and provide you with easy solutions to deal with it. We'll also address common issues and give you a compelling call-to-action that encourages reader engagement.
What is SettingWithCopyWarning? 🚧
The SettingWithCopyWarning
is a warning message that Pandas throws when you try to set a value on a copy of a slice from a DataFrame, instead of using the .loc[row_index, col_indexer] = value
syntax.
In simple terms, it means you are trying to modify a DataFrame that was created as a slice of another DataFrame, and Pandas is warning you that your changes may not propagate as you expect.
Understanding the Context 🖊️
Let's take a look at the function that is giving us these warnings:
def _decode_stock_quote(list_of_150_stk_str):
"""decode the webpage and return dataframe"""
from cStringIO import StringIO
str_of_all = "".join(list_of_150_stk_str)
quote_df = pd.read_csv(StringIO(str_of_all), sep=',', names=list('ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefg'))
quote_df.rename(columns={'A':'STK', 'B':'TOpen', 'C':'TPCLOSE', 'D':'TPrice', 'E':'THigh', 'F':'TLow', 'I':'TVol', 'J':'TAmt', 'e':'TDate', 'f':'TTime'}, inplace=True)
quote_df = quote_df.ix[:,[0,3,2,1,4,5,8,9,30,31]]
quote_df['TClose'] = quote_df['TPrice']
quote_df['RT'] = 100 * (quote_df['TPrice']/quote_df['TPCLOSE'] - 1)
quote_df['TVol'] = quote_df['TVol']/TVOL_SCALE
quote_df['TAmt'] = quote_df['TAmt']/TAMT_SCALE
quote_df['STK_ID'] = quote_df['STK'].str.slice(13,19)
quote_df['STK_Name'] = quote_df['STK'].str.slice(21,30)
quote_df['TDate'] = quote_df.TDate.map(lambda x: x[0:4]+x[5:7]+x[8:10])
return quote_df
This function decodes a webpage and returns a DataFrame. However, it seems that some of the lines of code in this function are causing the SettingWithCopyWarning
to be triggered.
Easy Solutions to Deal with the Warning 🛠️
To address the SettingWithCopyWarning
, you have two easy solutions:
Solution 1: Use .loc[row_index, col_indexer] = value
Instead of directly assigning a value to a DataFrame slice like quote_df['TVol'] = quote_df['TVol']/TVOL_SCALE
, use the .loc
indexer to set the value:
quote_df.loc[:, 'TVol'] = quote_df['TVol']/TVOL_SCALE
This syntax ensures that the change is made on the original DataFrame and not on a copy.
Solution 2: Make a Copy Explicitly
If you want to keep the original code and still avoid the warning, you can make a copy explicitly using the .copy()
method:
quote_df = quote_df.copy()
quote_df['TVol'] = quote_df['TVol']/TVOL_SCALE
By making a copy, you ensure that any subsequent modifications are made on the new DataFrame and not on the original slice.
Suspending the Warning ⚠️
If you insist on using the code that triggers the warning and want to suspend it temporarily, you can use the warnings
module in Python:
import warnings
warnings.filterwarnings('ignore', category=pd.core.common.SettingWithCopyWarning)
By filtering out the SettingWithCopyWarning
, you can continue using the code without seeing the warning messages. However, proceed with caution and ensure that the changes you make to the DataFrame are indeed propagating as expected.
Conclusion and Call-to-Action 🏁🔥
Now that you understand what the SettingWithCopyWarning
means and how to deal with it, you can confidently handle this common issue in Pandas. Don't let those warning messages stop you from writing awesome data manipulation code!
If you found this blog post helpful, share it with your friends and colleagues who work with Pandas. And remember, keep coding smart and stay curious! 😎💪