How to create a dictionary of two pandas DataFrame columns
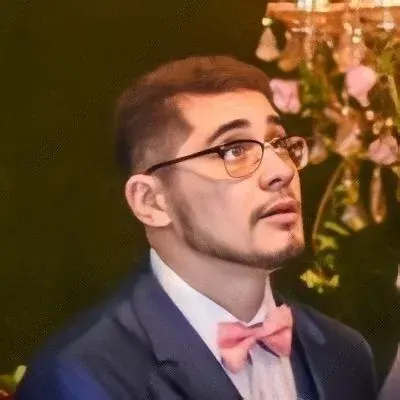
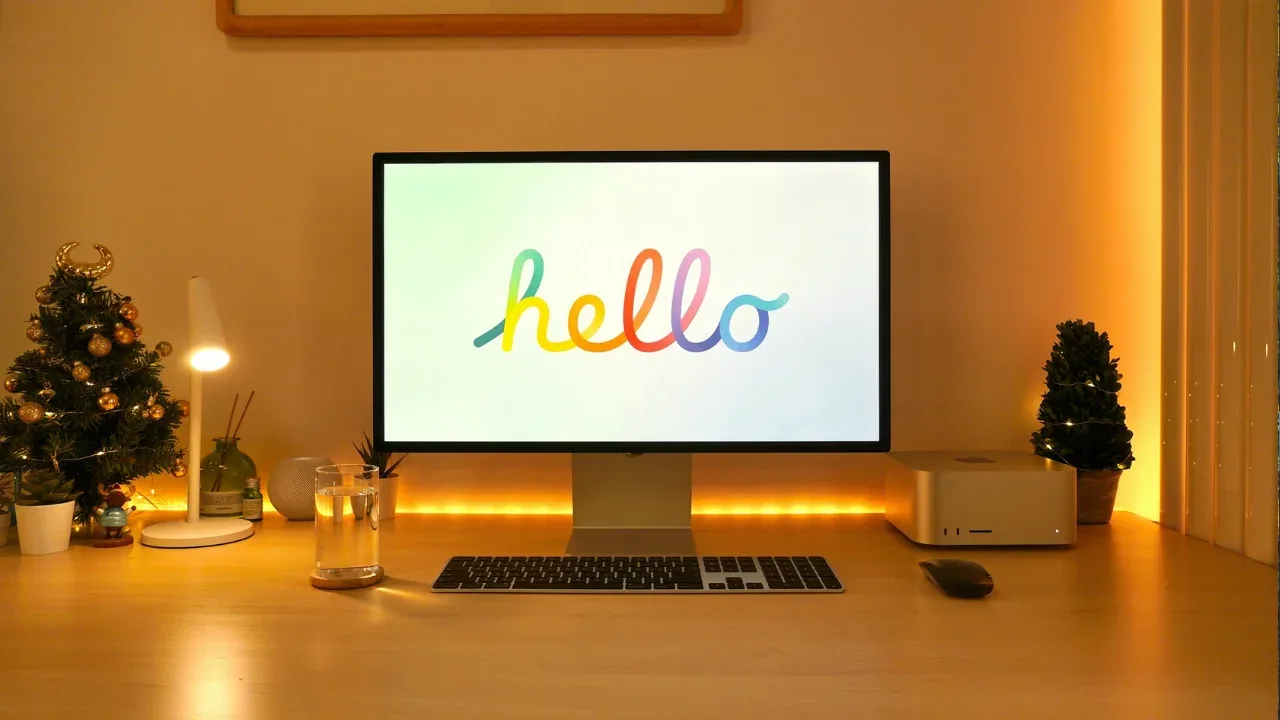
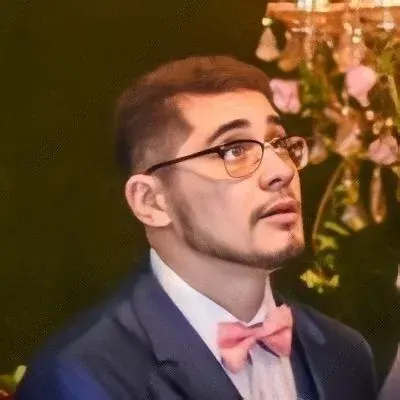
How to Create a Dictionary of Two Pandas DataFrame Columns 😎🐼
Are you ready to level up your data organization game? 😄 In this blog post, we'll dive into the world of pandas DataFrames and explore the most efficient way to create a dictionary from two columns. By the end, you'll have a handy tool to organize and access your data. Let's get started! 💪🚀
The Problem
Imagine you have a pandas DataFrame with two columns, "Position" and "Letter":
import pandas as pd
data = pd.DataFrame({"Position": [1, 2, 3, 4, 5],
"Letter": ['a', 'b', 'c', 'd', 'e']})
You want to create a dictionary where the keys are the values from the "Position" column and the values are the corresponding values from the "Letter" column. The desired output should be:
alphabet = {1: 'a', 2: 'b', 3: 'c', 4: 'd', 5: 'e'}
The Solution
The easiest way to create the desired dictionary is by utilizing the zip()
function in Python. Here's the one-liner that does the magic:
alphabet = dict(zip(data['Position'], data['Letter']))
Let's break it down:
zip(data['Position'], data['Letter'])
combines the two columns and creates pairs of values. This creates an iterable object that can be transformed into a dictionary.dict(...)
takes the iterable object and converts it into a dictionary.
Isn't that neat? 😄
Handling Potential Issues
Issue 1: Non-Unique Keys
If your DataFrame has duplicate values in the "Position" column, using the zip()
function directly would result in losing some values. To avoid that, you can use the groupby()
function to concatenate the values in the "Letter" column. Here's an example:
alphabet = dict(data.groupby('Position')['Letter'].apply(''.join))
Issue 2: Missing Values
In case your DataFrame has missing values in either the "Position" or "Letter" column, the zip()
function will exclude those rows from the final dictionary. So, make sure your data is complete before using this approach.
Try It Yourself! 🚀
Now that you know how to create a dictionary from two pandas DataFrame columns, it's time to put your new skills into practice! Grab your own DataFrame, follow the steps outlined above, and create your personalized dictionary.
If you encounter any issues or have any questions, don't hesitate to reach out in the comments section below. Our community is always here to help you out! 😊
Happy coding! 💻🎉
Did you find this guide helpful? Leave a comment with your experience or any other data organization tips you'd like to share! Let's learn from each other and boost our productivity together! 💪📈
Don't forget to hit the share button and spread the pandas love to your tech-savvy friends! They'll thank you for it! 🙌🎁