How to correct TypeError: Unicode-objects must be encoded before hashing?
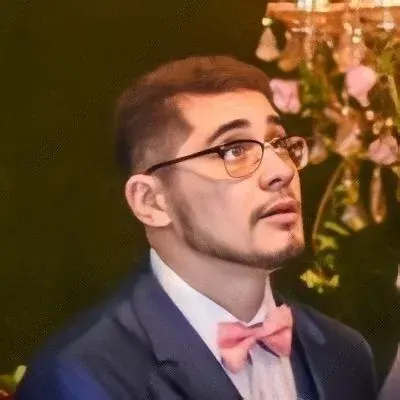
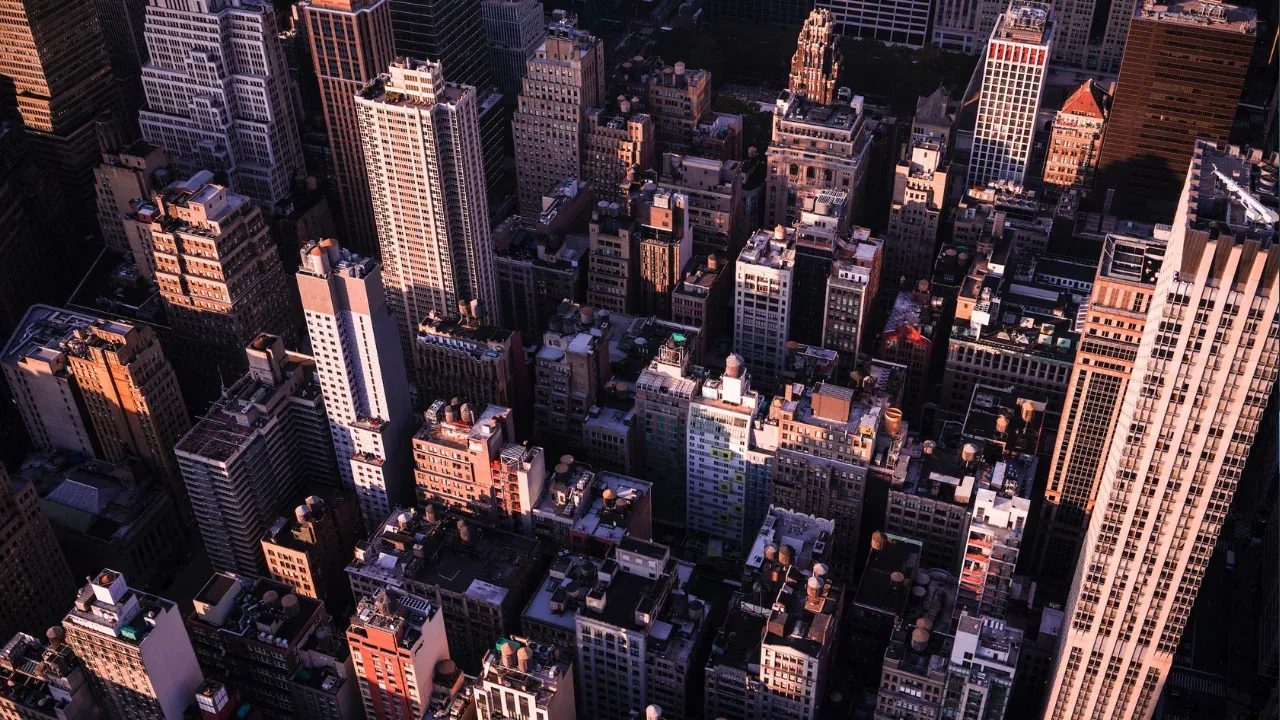
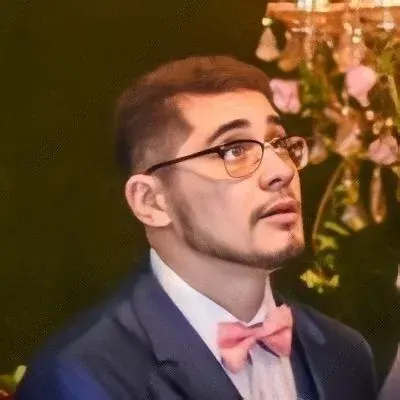
How to correct TypeError: Unicode-objects must be encoded before hashing? 😕
So, you're trying to execute some code in Python 3.2.2, but you ran into this error:
Traceback (most recent call last):
File "python_md5_cracker.py", line 27, in <module>
m.update(line)
TypeError: Unicode-objects must be encoded before hashing
Don't worry, this error is fixable! Let's dive in and solve this problem together. 💪
Understanding the Error
First, let's understand what this error message is trying to tell us. The error is raised when trying to use a Unicode object as input for a hashing function without encoding it beforehand. In the specific code snippet you provided, the error is occurring on line 27 where m.update(line)
is called.
The Fix: Encoding Unicode Objects
To resolve this error, we need to encode the Unicode object before passing it to the m.update()
function. The most common encoding to use is UTF-8, but you can also use other encodings depending on your specific requirements.
Here's how you can modify your code to fix the error:
m.update(line.encode('utf-8'))
With this change, you're encoding the line
variable as UTF-8 before updating the hash object with it. This should prevent the TypeError
from occurring.
Updated Code:
import hashlib, sys
m = hashlib.md5()
hash = ""
hash_file = input("What is the file name in which the hash resides? ")
wordlist = input("What is your wordlist? (Enter the file name) ")
try:
hashdocument = open(hash_file, "r")
except IOError:
print("Invalid file.")
input()
sys.exit()
else:
hash = hashdocument.readline()
hash = hash.replace("\n", "")
try:
wordlistfile = open(wordlist, "r")
except IOError:
print("Invalid file.")
input()
sys.exit()
else:
pass
for line in wordlistfile:
m = hashlib.md5()
line = line.replace("\n", "")
m.update(line.encode('utf-8')) # Fix: Encode the Unicode string
word_hash = m.hexdigest()
if word_hash == hash:
print("Collision! The word corresponding to the given hash is", line)
input()
sys.exit()
print("The hash given does not correspond to any supplied word in the wordlist.")
input()
sys.exit()
🎉 Problem Solved!
Congratulations! You've successfully fixed the TypeError
issue. Now you can run your code without running into that specific error.
Keep in mind that encoding Unicode objects is important when working with hashing functions or any other operations that require byte input. So, make sure to encode your Unicode objects appropriately to avoid similar errors in the future.
📣 Share Your Experience
Did this solution help you? We'd love to hear about your experience! Share your thoughts, feedback, or any other issues you're facing in the comments below. Let's learn and grow together! 🌟