How to convert string to binary?
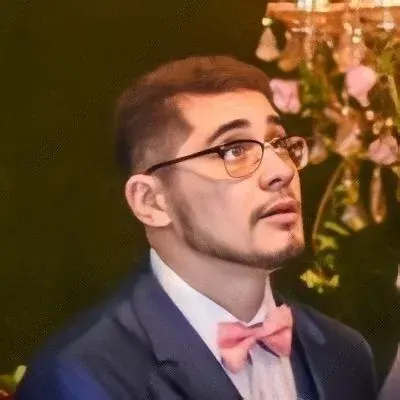
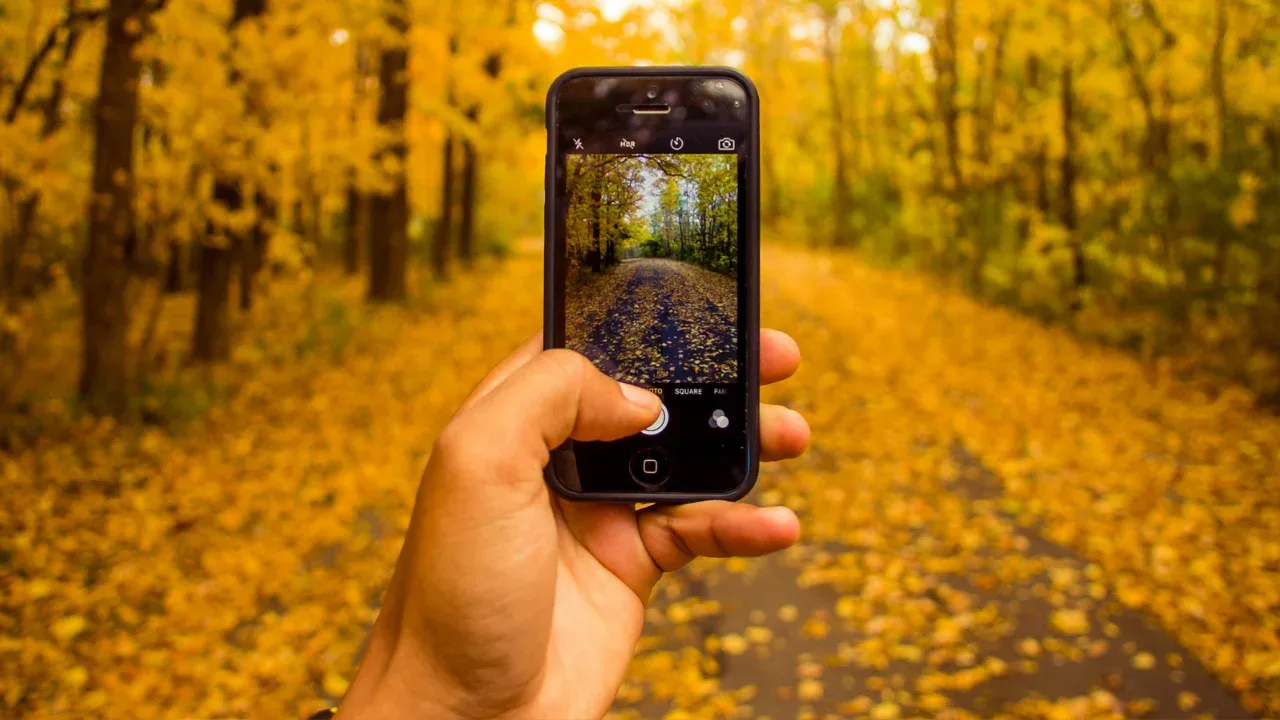
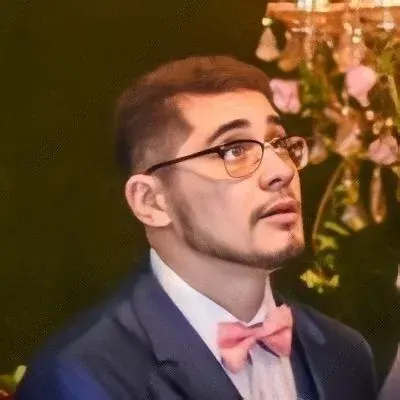
💬 Easy Guide: How to Convert a String to Binary in Python
Are you looking for a way to convert a string into its binary representation? Look no further! In this blog post, we will explore a simple and neat solution to achieve this in Python.
🤔 The Challenge
Let's start with the challenge at hand. We have a string, for example, "hello world"
, and we want to get the binary representation of each character in the string. We're not sure whether there is a built-in module or a convenient method to accomplish this task.
⚡️ The Solution
Fortunately, Python provides a straightforward way to convert a string into binary. We can achieve this by using the built-in ord()
function along with a list comprehension. Here's the code snippet that does the trick:
def toBinary(string):
binary = [format(ord(char), '08b') for char in string]
return binary
st = "hello world"
binary_representation = toBinary(st)
print(binary_representation)
By iterating over each character in the string and applying the ord()
function, we convert each character into its corresponding Unicode value. Then, we use the format()
function with the '08b'
format specifier to format each Unicode value as an 8-bit binary representation. Finally, we collect all the binary representations in a list.
When you run this code, it will output:
['01101000', '01100101', '01101100', '01101100', '01101111', '00100000', '01110111', '01101111', '01110010', '01101100', '01100100']
Voila! We have successfully converted the string "hello world"
into its binary representation ✨
✨ Let's Break It Down
Now, let's break down the line of code that does the actual conversion:
binary = [format(ord(char), '08b') for char in string]
ord(char)
: Theord()
function returns an integer representing the Unicode character.format(value, '08b')
: Theformat()
function is used to format the value as a binary representation. The08b
format specifier ensures that the result is formatted as an 8-bit binary value.for char in string
: The list comprehension iterates over each character in the string.
📚 Going Further
You have successfully learned how to convert a string to binary in Python. But there's even more you can do with this newfound knowledge! Here are a few ideas to explore:
Reverse the process: Convert binary back into a string using the
chr()
function.Create a binary converter program that can handle multiple inputs from the user.
Feel free to experiment and explore the possibilities!
🚀 Get Coding!
Now that you have the knowledge and code at your fingertips, it's time to give it a try yourself. Open up your Python environment, copy the code snippet, and start converting strings to binary with ease!
If you have any questions or suggestions, feel free to leave a comment below. Happy coding! 🎉