How to convert string representation of list to a list
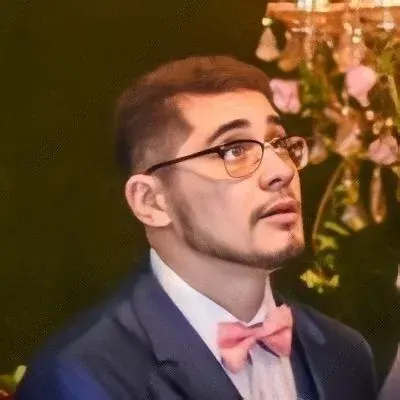
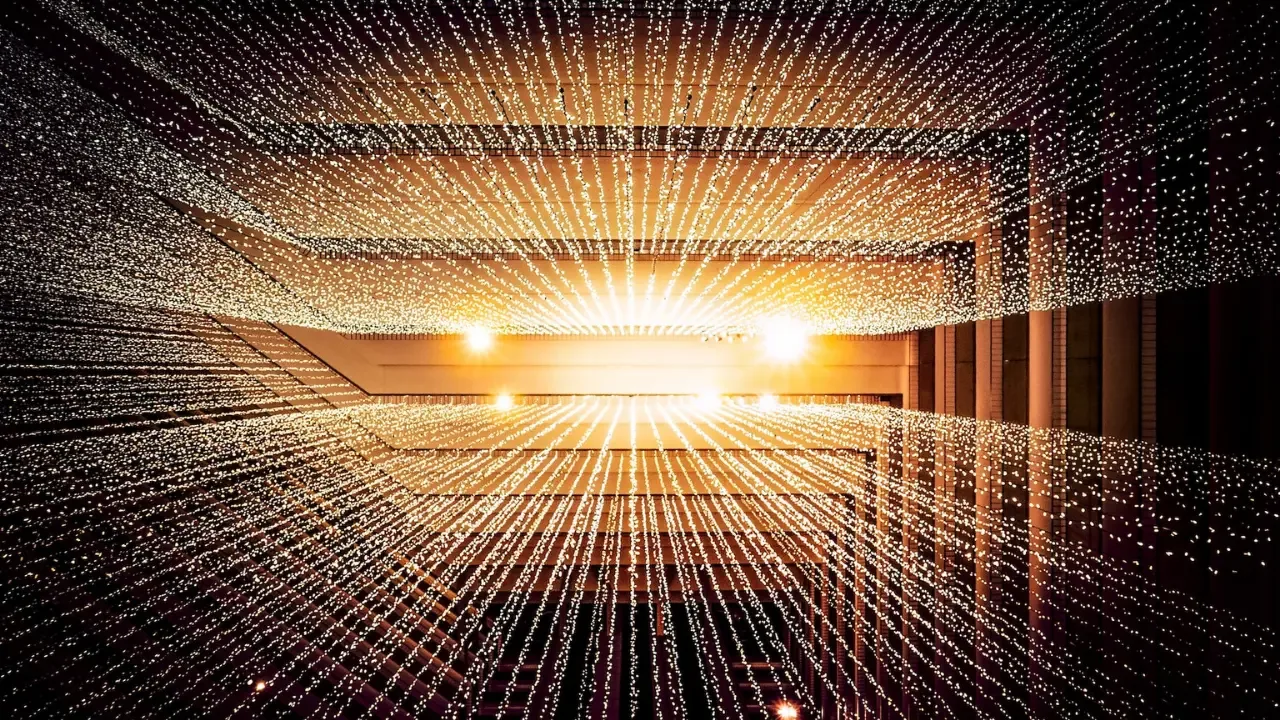
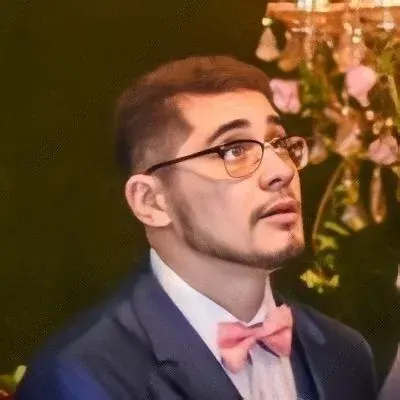
How to Convert a String Representation of a List to a List
Have you ever come across a situation where you need to convert a string representation of a list into an actual list in your code? 🤔 Don't worry, you're not alone! This is a common problem that many developers face, and fortunately, there are simple solutions available. In this blog post, we will explore different methods to convert a string representation of a list to a list, even when dealing with spaces and non-letter characters.
The Problem
Let's start by understanding the problem at hand. You have a string variable x
that represents a list:
x = '[ "A","B","C" , " D"]'
Your goal is to convert this string representation into a Python list:
x = ["A", "B", "C", "D"]
However, there may be additional challenges to overcome. The string could contain spaces between the commas and within the quotes, making the conversion process trickier.
The Kludgy Approach
Before we dive into the quick and easy solutions, let's discuss the kludgy approach the original author mentioned. The approach involved using strip()
and split()
functions, along with manual checking for non-letter characters. While this approach may work, it can quickly become messy and convoluted, especially as the complexity of the string representation increases.
The Quick and Easy Solutions
Fortunately, Python provides powerful built-in functions and libraries that simplify this task. Here are a few quick and easy solutions to convert a string representation of a list to a list:
Solution 1: Using the eval()
function
The eval()
function in Python can evaluate and execute any valid Python expression, including a list. It takes a string as input and returns the corresponding Python object. In our case, we can use eval()
to convert our string representation of the list to an actual list:
x = '[ "A","B","C" , " D"]'
x = eval(x)
Although eval()
is a powerful tool, it's important to be cautious when using it. Since it can execute any valid Python expression, it may also execute malicious code if the input comes from an untrusted source. Use it only when you have control over the input or trust the source.
Solution 2: Using the json
module
Another quick and safe solution involves using the json
module, which provides functionality for working with JSON data. Although our string representation is not in strict JSON format, we can make a few adjustments and utilize json.loads()
to convert it to a list:
import json
x = '[ "A","B","C" , " D"]'
x = json.loads(x.replace("'", '"'))
Here, we use replace()
to convert single quotes to double quotes, making our string representation valid JSON. Then, json.loads()
safely converts it to a list.
Solution 3: Using a List Comprehension
If you prefer a more hands-on approach without relying on external libraries, you can use a list comprehension to tackle this problem. Here's how:
x = '[ "A","B","C" , " D"]'
x = x[1:-1].replace('"', '').split(',')
x = [item.strip() for item in x]
In this solution, we first remove the square brackets using string slicing (x[1:-1]
). Then, we remove the quotes using replace('"', '')
and split the string into a list using split(',')
. Finally, we use a list comprehension and strip()
to remove any additional spaces.
Choose the solution that suits your needs and coding style the best!
Call-to-Action: Engage with the Community
We hope these solutions help you convert string representations of lists into actual lists effortlessly. If you found this blog post helpful or have any questions, share your thoughts in the comments section below. Let's learn from each other and help the community grow together! 🌱💪
Now go forth and conquer the world of list conversions! Happy coding! 🚀✨