How to convert JSON data into a Python object?
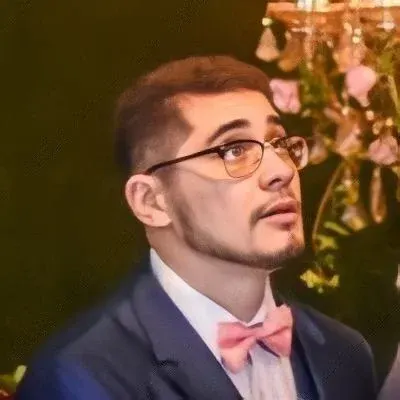
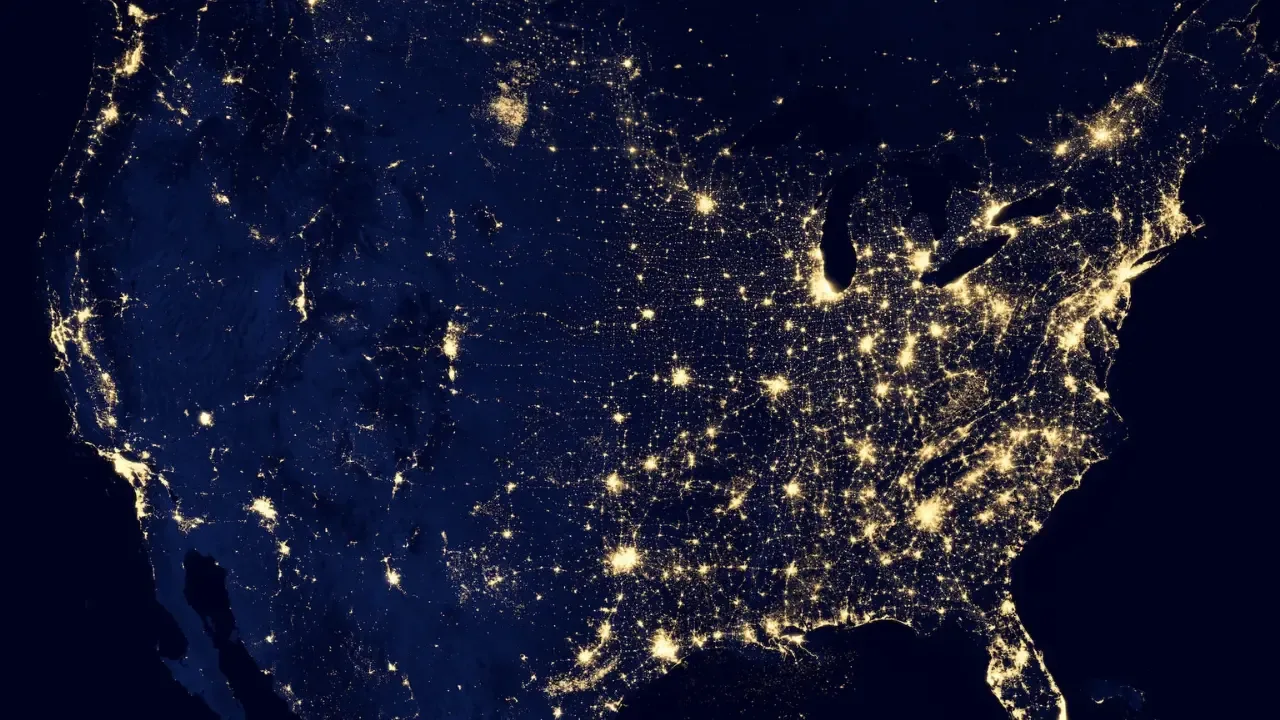
Converting JSON data into a Python object: A quick and easy guide! 📚
So, you want to convert JSON data into a Python object, huh? 🤔 Well, you're in the right place! In this guide, we'll take a deep dive into this topic, addressing common issues and providing easy solutions to help you conquer this challenge. Let's get started! 💪
Understanding the problem 🧐
Imagine you receive JSON data objects from the Facebook API and you want to store them in your database. The example code you provided works fine for simple JSON data. However, things can get a bit tricky when dealing with complex JSON structures. 😫
Introducing the solution: json
module 🚀
To convert JSON data into a Python object, we'll leverage the power of the json
module. This module provides functions that allow us to handle JSON data effortlessly.
Here's how you can do it: ✨
import json
# Assuming you have your JSON data in a variable named `json_data`
python_object = json.loads(json_data)
By using the loads()
function, we can convert JSON data into a Python object. This object can be a list
, dict
, int
, str
, float
, or even bool
, depending on the structure of the JSON data.
Handling complex JSON data objects 🌟
Now, let's address the issue you brought up about handling complex JSON data objects. 🤝
If your JSON data consists of nested objects or arrays, fear not! The json
module has got your back. It automatically converts JSON nested structures into corresponding Python objects. 🙌
For example, consider the following JSON data:
{
"name": "John Doe",
"age": 30,
"address": {
"street": "123 Main St",
"city": "New York",
"state": "NY"
},
"hobbies": ["reading", "coding", "swimming"]
}
After converting this JSON data into a Python object, you can access the values and nestings like this:
name = python_object['name'] # John Doe
age = python_object['age'] # 30
street = python_object['address']['street'] # 123 Main St
city = python_object['address']['city'] # New York
hobbies = python_object['hobbies'] # ['reading', 'coding', 'swimming']
You see, even with complex JSON data structures, the json
module simplifies the conversion process. 🎉
Your turn to shine: Start converting JSON to Python! ✨
Now that you know how to convert JSON data into a Python object, it's time to put your skills to the test! Start leveraging this technique in your code to extract meaningful data from JSON and utilize it in your project. 💡
Remember, the json
module in Python is your ally when it comes to working with JSON data. Explore its documentation to explore other powerful capabilities it offers.
Share your success! 🎉
We hope this guide has helped you understand the process of converting JSON data into a Python object. Now, it's your turn to engage with us! Drop a comment or reach out on social media to let us know how this technique benefited you or share any cool tips you may have. 🙌
Happy coding! 💻✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
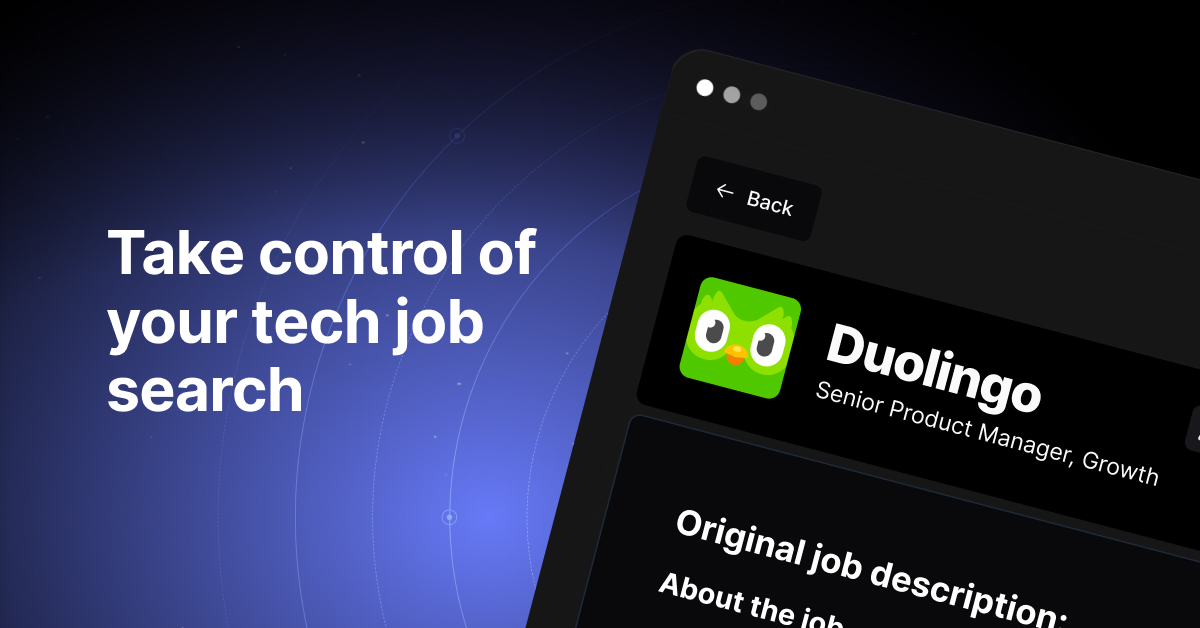