How to convert "binary string" to normal string in Python3?
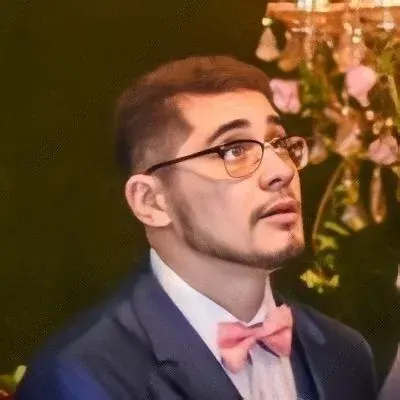
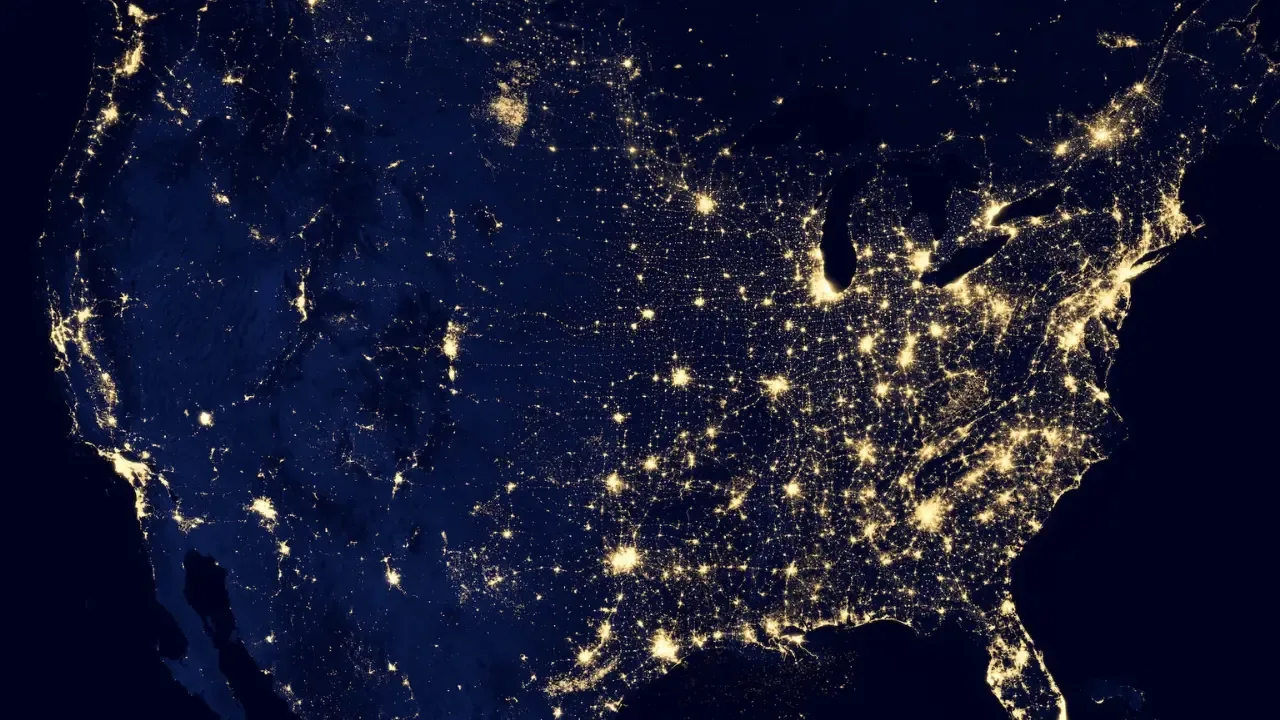
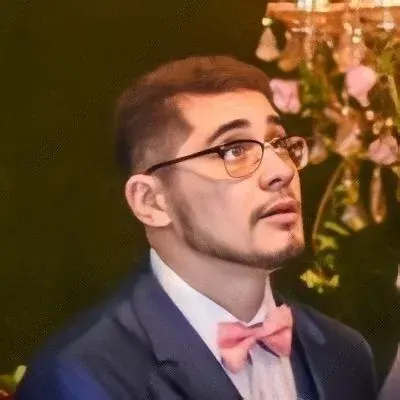
How to Convert 'Binary String' to Normal String in Python3?
Have you ever come across a situation where you have a binary string in Python and you want to convert it into a regular string? 🤔
Well, worry no more! In this guide, I'm going to walk you through a simple solution to this common problem. But before we dive into the solution, let's understand the problem a bit better.
Understanding the Problem
When you have a binary string in Python, it is often represented with a prefix b'
, which can be quite annoying, especially when you want to print or use it as a normal string.
Here's an example to give you a clearer picture:
b'a string'
As you can see, the binary string is enclosed within b''
. So, any attempt to print or convert it using str()
won't give you the desired result:
print(b'a string') # Output: b'a string'
print(str(b'a string')) # Output: b'a string'
Now that we understand the issue, let's move on to the solution.
The Solution
To convert a binary string into a regular string, you can use the decode()
method. The decode()
method is available in Python's bytes
object and allows you to specify the encoding to be used.
Here's an example of how you can use the decode()
method to solve our problem:
binary_string = b'a string'
normal_string = binary_string.decode('utf-8')
print(normal_string) # Output: a string
In the example above, the binary string is decoded using the UTF-8 encoding, which is the most common encoding used for text in Python. The result is stored in the normal_string
variable and can be printed or used just like any other regular string.
It's important to note that the encoding used to decode the binary string should match the encoding of the original text. In most cases, UTF-8 should work fine, but you may need to choose a different encoding based on your specific requirements.
Call-to-Action
Now that you know how to convert a binary string to a normal string in Python, give it a try in your own code! If you have any questions or face any challenges while implementing this solution, feel free to leave a comment below. I'll be more than happy to help you out! 😊
Remember, sharing is caring! If you found this guide helpful, don't forget to share it with your fellow Pythonistas. Happy coding! 👩💻🐍👨💻
Juan John 🚀