How to convert an XML string to a dictionary?
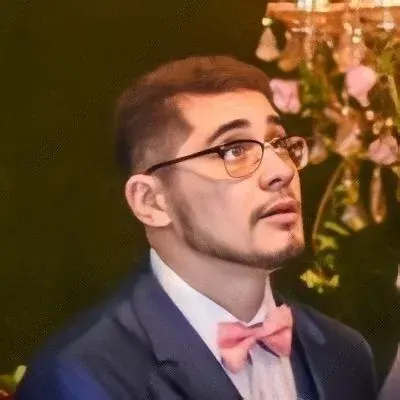
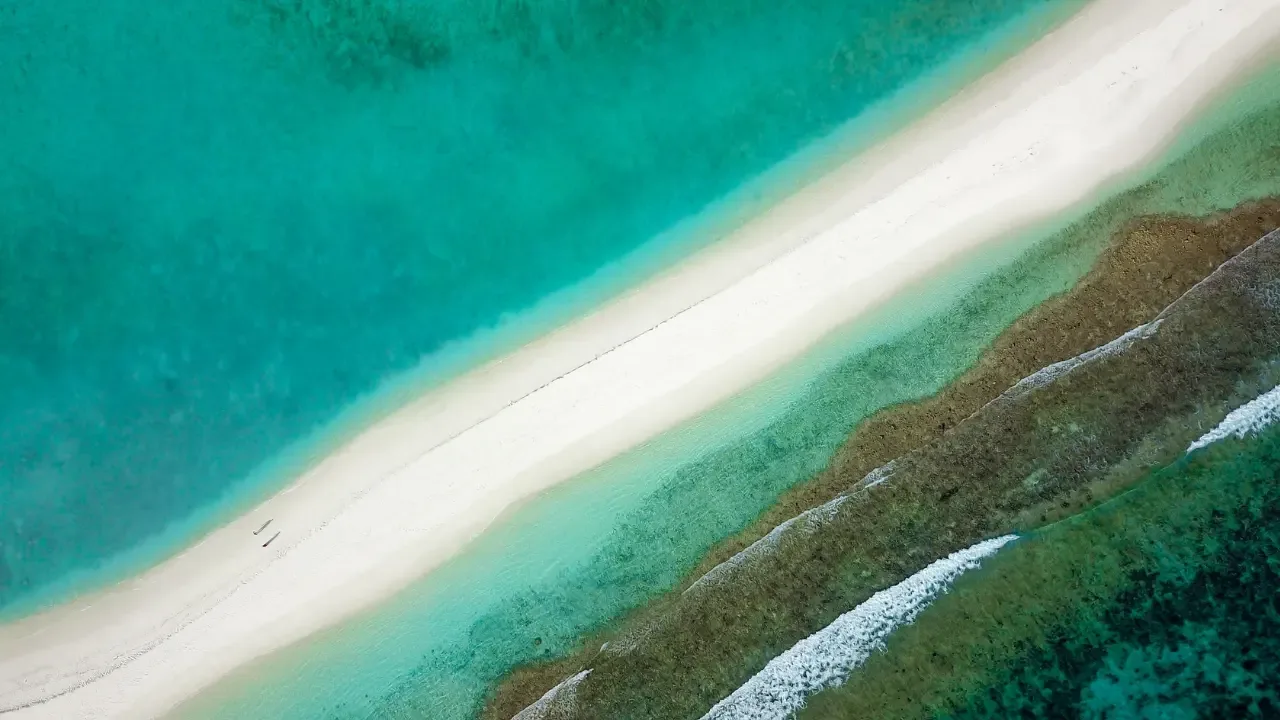
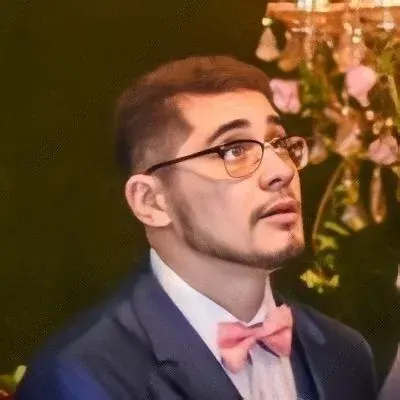
š Hey there, tech enthusiasts! Today, we're diving into the realm of XML and dictionaries. š We'll explore the question of how to convert an XML string to a dictionary in a Python program. So, let's get started! š
š The Problem:
Imagine you have a program that receives an XML document from a socket. š” You have stored the XML document as a string and now want to convert it into a Python dictionary. š¤ This seamless conversion is similar to what Django's simplejson
library offers.
šÆ The Desired Outcome: Given an XML string, the goal is to convert it into a dictionary. For example, if we have the following XML string:
str = "<?xml version="1.0" ?><person><name>john</name><age>20</age></person>"
We aim to transform this XML string, str
, into the corresponding dictionary:
dic_xml = {'person': {'name': 'john', 'age': 20}}
Now that we know what we want to achieve, let's explore some solutions! š”
š§ Solution 1 - Using the xmltodict
Library:
One way to convert an XML string to a dictionary is by using the xmltodict
library in Python. This library simplifies the process by providing a convenient parse()
function. Here's how you can use it:
import xmltodict
str = "<?xml version="1.0" ?><person><name>john</name><age>20</age></person>"
# Convert XML string to dictionary
dic_xml = xmltodict.parse(str)
print(dic_xml)
š§ Solution 2 - Using ElementTree
:
Another approach is to use Python's built-in ElementTree
module. This module provides easy-to-use functionality for parsing XML documents. Here's an example:
import xml.etree.ElementTree as ET
str = "<?xml version="1.0" ?><person><name>john</name><age>20</age></person>"
# Create ElementTree object from XML string
tree = ET.ElementTree(ET.fromstring(str))
# Get the root element
root = tree.getroot()
# Convert XML element to dictionary
dic_xml = {}
for child in root:
dic_xml[child.tag] = child.text
print(dic_xml)
š¼ Reader Engagement Time! Now that you have easy solutions to convert XML strings to dictionaries, what other challenges do you face when dealing with XML data? Share your experiences and thoughts in the comments below! Let's learn from each other and build a stronger tech community! šš¤
That's it for today's blog post! We explored how to convert XML strings to dictionaries using two different libraries. Whether you choose xmltodict
or ElementTree
, you'll be able to handle XML data like a pro! š
If you found this blog post helpful, be sure to share it with your fellow tech enthusiasts! Let's spread the knowledge and make coding easier for everyone! šš»
Stay tuned for more exciting tech tips and tricks. Until next time, happy coding! šāØ