How to combine multiple QuerySets in Django?
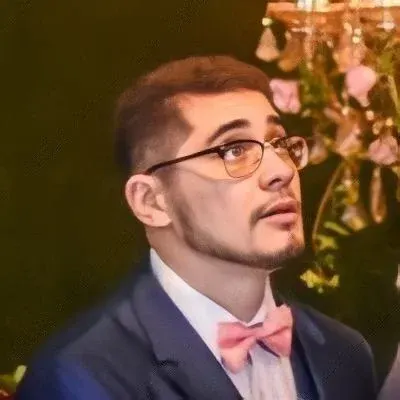
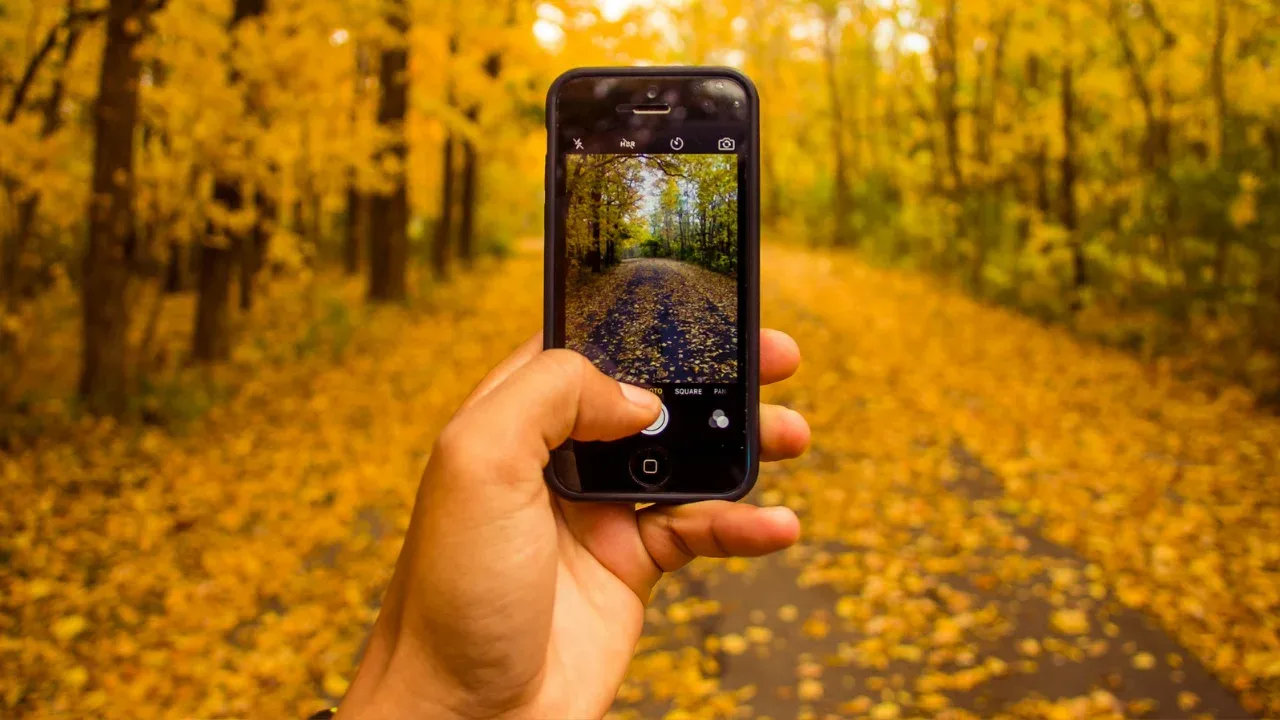
Combine Multiple QuerySets in Django: A Complete Guide 😎
So you're building a Django site and trying to implement a powerful search functionality across three different models. You want to use the generic object_list
view to display the search results with pagination. But here's the kicker - you need to merge three QuerySets into one to make it work seamlessly. Don't worry, I've got you covered! 😁
The Problem 🤔
You've tried combining the QuerySets using a simple approach like the one below but hit a roadblock:
result_list = []
page_list = Page.objects.filter(
Q(title__icontains=cleaned_search_term) |
Q(body__icontains=cleaned_search_term))
article_list = Article.objects.filter(
Q(title__icontains=cleaned_search_term) |
Q(body__icontains=cleaned_search_term) |
Q(tags__icontains=cleaned_search_term))
post_list = Post.objects.filter(
Q(title__icontains=cleaned_search_term) |
Q(body__icontains=cleaned_search_term) |
Q(tags__icontains=cleaned_search_term))
for x in page_list:
result_list.append(x)
for x in article_list:
result_list.append(x)
for x in post_list:
result_list.append(x)
return object_list(
request,
queryset=result_list,
template_object_name='result',
paginate_by=10,
extra_context={
'search_term': search_term},
template_name="search/result_list.html")
Unfortunately, the above approach doesn't work as expected. It throws an error because the list you're using lacks the clone
attribute.
The Solution 💡
To successfully merge multiple QuerySets in Django, we can leverage the power of the chain
function from the itertools
module. Here's how you can do it:
from itertools import chain
result_list = list(chain(page_list, article_list, post_list))
return object_list(
request,
queryset=result_list,
template_object_name='result',
paginate_by=10,
extra_context={
'search_term': search_term},
template_name="search/result_list.html")
By using itertools.chain
, we concatenate the QuerySets page_list
, article_list
, and post_list
into a single iterable. Then, by converting it to a list, we create the merged QuerySet result_list
that can be passed to the object_list
view without the clone
attribute error.
Conclusion and Call-to-Action 👏
Voila! You now have a foolproof way to combine multiple QuerySets in Django using the itertools.chain
function. Say goodbye to that pesky clone
attribute error and enjoy the seamless merging of your search results across multiple models.
If you found this guide useful, don't forget to share it with your Django developer friends. And if you have any further questions or suggestions, I'd love to hear from you in the comments below. Happy coding! 🚀🔥
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
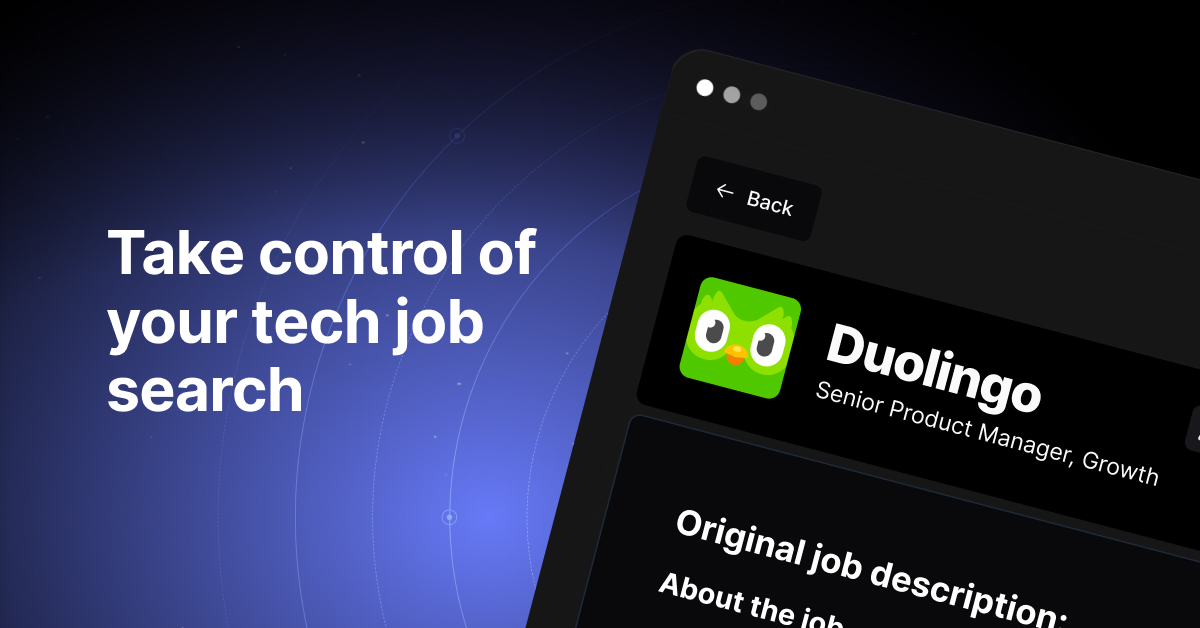