How to clear the interpreter console?
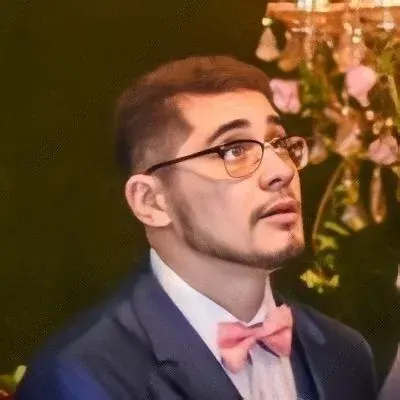
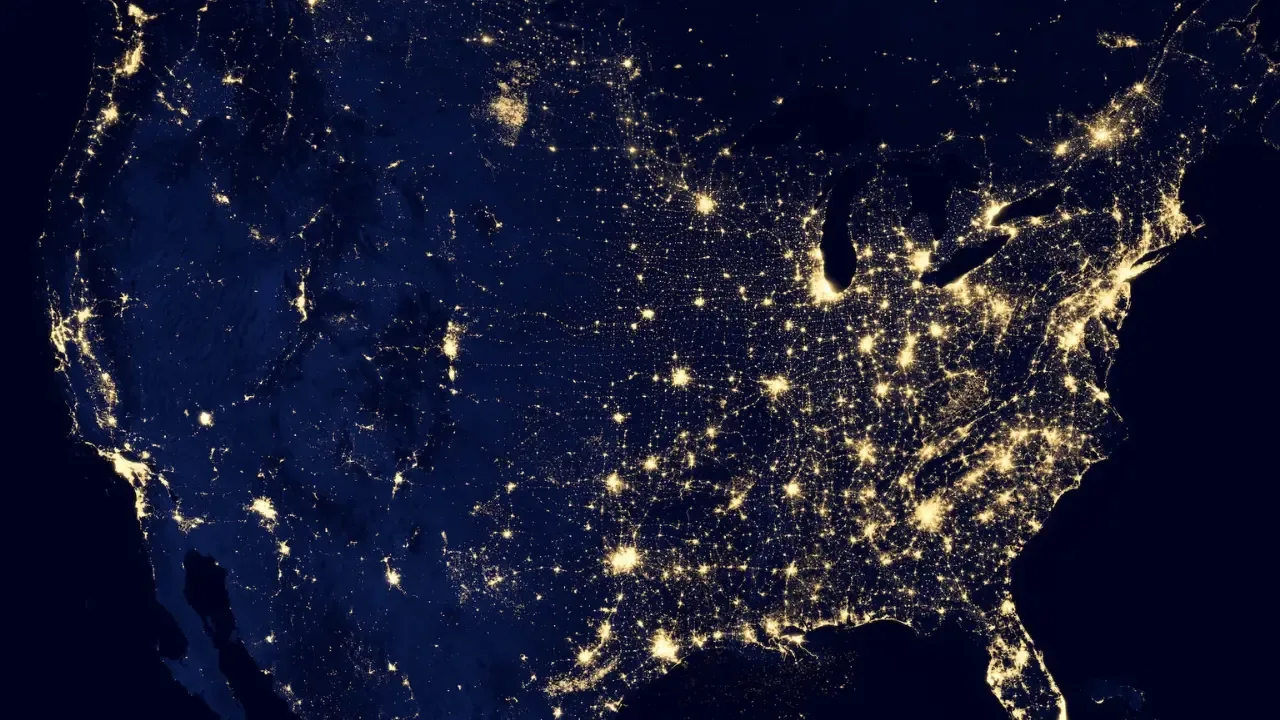
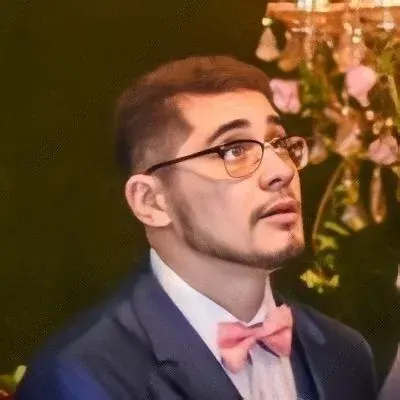
How to Clear the Interpreter Console: A Simple Guide for Python Developers 👨💻
So, you've been using the Python interpreter 🐍 to test your commands, check out the dir()
and help()
functions, and generally explore the Python universe. But now, the console is starting to get cluttered with past commands and prints, making it confusing to re-run commands multiple times. Don't worry, we've got you covered! In this guide, we'll show you a couple of easy solutions to clear the interpreter console and keep things clean. Let's get started! 🚀
The System Call Approach
First, let's address the system call approach you've mentioned. While it's true that you can use system calls like cls
on Windows or clear
on Linux to clear the console, it might not be the most convenient option. Luckily, there's a simpler way to achieve the same result within the Python interpreter itself. 💡
Solution 1: Using os
and sys
Modules
To clear the interpreter console programmatically, you can utilize the os
and sys
modules in Python. Here's a step-by-step explanation on how to do it:
Import the necessary modules:
import os import sys
Define a function that clears the console:
def clear_console(): if sys.platform.startswith('win'): # Windows os.system('cls') else: # Linux or macOS os.system('clear')
Now, whenever you want to clear the console, simply call the
clear_console()
function:clear_console()
By leveraging this approach, you can easily clear the interpreter console without the need for system-specific commands. 🎉
Solution 2: Utilizing the IPython Package
If you are using the IPython interpreter, there's an even easier way to clear the console. In IPython, you can use the magic command %clear
to clear the console. Here's how:
Install the IPython package if you haven't already:
pip install ipython
Launch the IPython interpreter by typing
ipython
in your console.To clear the console, simply type
%clear
and hit enter:%clear
And voilà! The console is now sparkling clean and ready for new adventures in the Python world. 🌟
A Request for Readers
We hope you found these solutions helpful for clearing the interpreter console. If you have any other tips or tricks, or if you've encountered any other issues related to the Python interpreter console, we would love to hear from you! Share your thoughts and experiences in the comments below, and let's build a vibrant community of Pythonistas! 🎉
Thanks for reading, and happy coding! 💻✨