How to check whether a pandas DataFrame is empty?
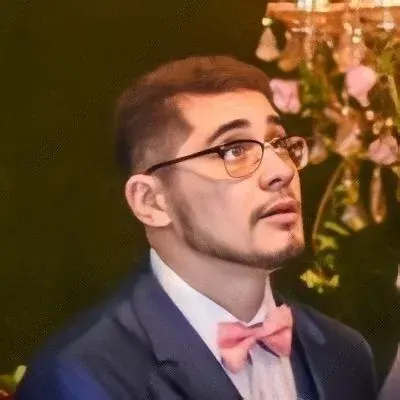
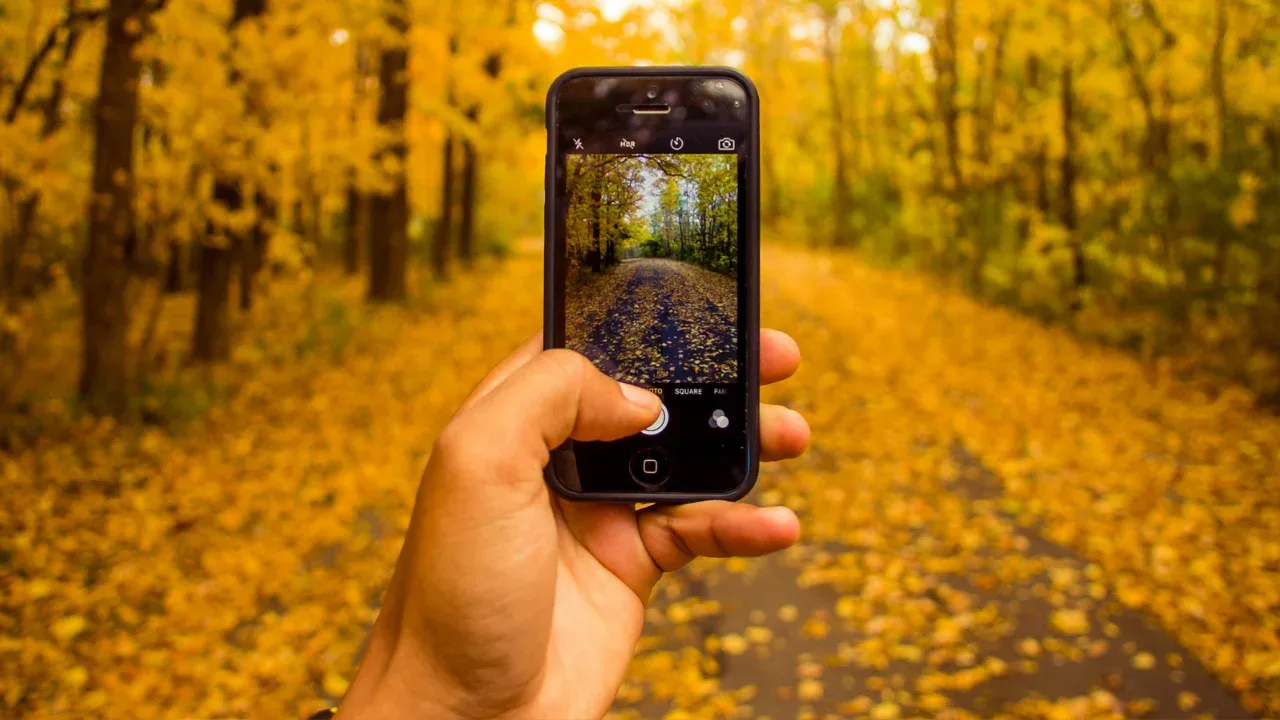
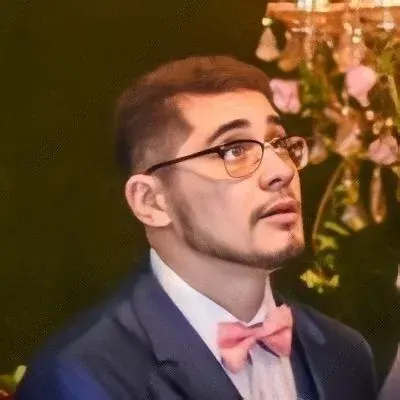
Is Your Pandas DataFrame Empty? Here's How to Check!
So, you've got a pandas DataFrame and you want to know if it's empty, huh? 🧐 Don't worry, we've got you covered! In this blog post, we'll walk you through the simple steps to determine whether your DataFrame is empty or not. Let's dive in! 💪
The Scenario
Before we begin, let's set the stage. You want to print a message in the terminal if your DataFrame is empty. Sounds like a common task, right? 😄
The Solution
To check whether your DataFrame is empty, one easy method is to use the empty
attribute. Let's see how it works:
import pandas as pd
# Create an empty DataFrame
df = pd.DataFrame()
# Check if the DataFrame is empty
if df.empty:
print("Oops! The DataFrame is empty.")
In the example above, we import the pandas library and create an empty DataFrame called df
. Next, we use the .empty
attribute to check if the DataFrame is empty. If it is, we print a helpful message in the terminal.
Going Beyond the Obvious
But hey, we've got more tricks up our sleeves! What if your DataFrame has some columns with data but others are empty? You might still want to consider it empty overall. Here's how you can do that:
import pandas as pd
import numpy as np
# Create a DataFrame with empty columns
df = pd.DataFrame({'Column1': [1, 2, 3], 'Column2': np.nan})
# Check if the DataFrame is empty overall
if df.dropna().empty:
print("Whoops! The DataFrame is empty overall.")
In this example, we use the pandas library again and create a DataFrame called df
with two columns. The first column contains some values, while the second column is intentionally left empty using the np.nan
value. We then use a combination of .dropna()
and .empty
to check if the DataFrame is empty overall. If it is, we print another helpful message in the terminal.
Your Turn to Explore
Now that you know how to check whether your pandas DataFrame is empty or not, it's time to put your newfound knowledge into action! Go ahead and try it out in your own projects. If you encounter any issues or have further questions, don't hesitate to reach out. We're always here to help! 😊
Wrapping Up
Checking if your pandas DataFrame is empty is a breeze when you know the right tools. In this blog post, we learned how to use the .empty
attribute to check for emptiness. We even went a step further to handle scenarios where only some columns are empty. Now it's your turn to apply these techniques and make your projects more robust. Happy coding! 👩💻👨💻
If you found this guide helpful, don't forget to share it with your tech-savvy friends. We love to spread the knowledge! 🌟💡
Now go out there and conquer those empty DataFrames like the coding champ you are! 💪✨