How to check if variable is string with python 2 and 3 compatibility
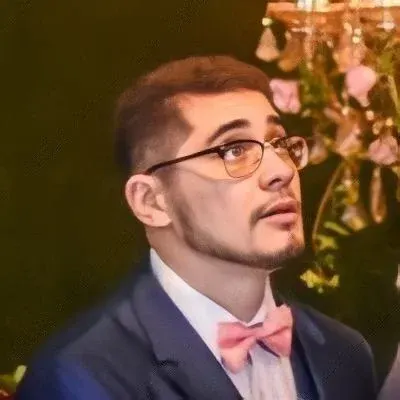
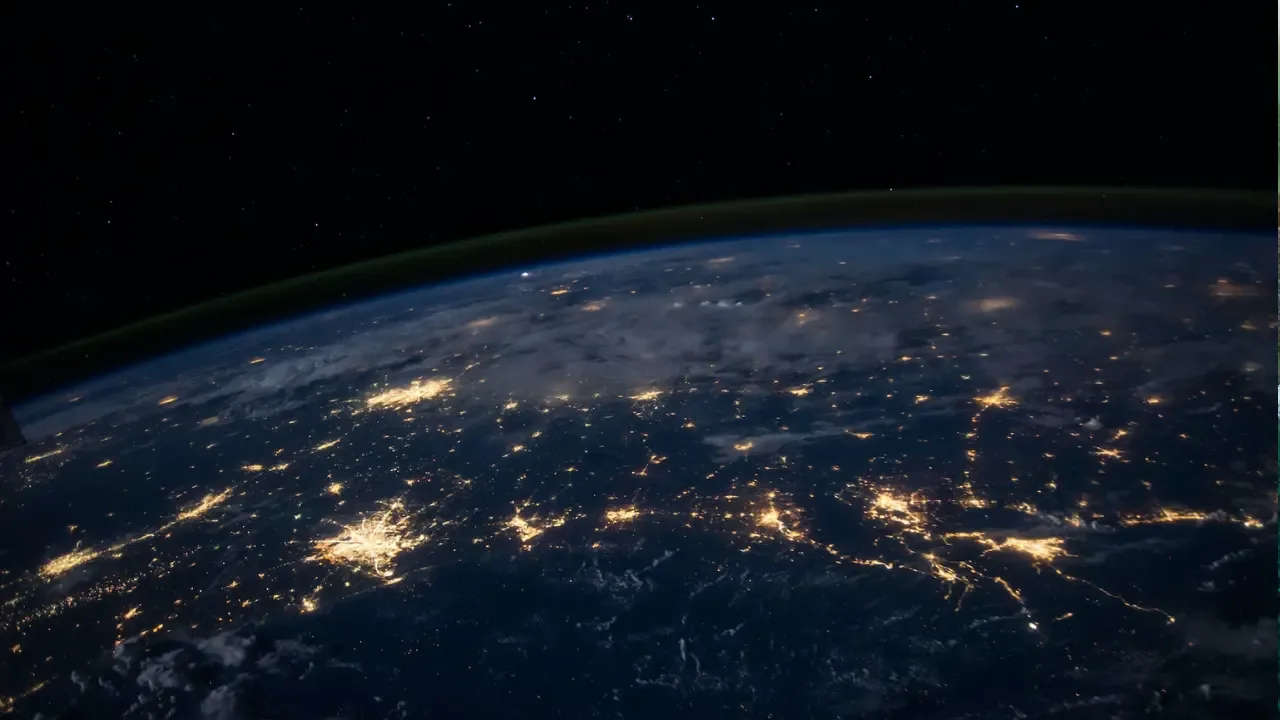
How to Check if a Variable is a String in Python 2 and 3 Compatibility
So you're writing some Python code and you need to check if a variable is a string. Easy, right? Just use the isinstance(x, str)
method in Python 3.x and you're good to go. But what about Python 2.x? Will isinstance(x, str)
work there too? 🤔
The short answer is no, it won't. In Python 2.x, str
and unicode
are distinct types, whereas in Python 3.x, str
is the only type for representing text. This difference can cause some confusion and compatibility issues when writing code that needs to run on both versions.
The Problem
Let's take a closer look at the problem. In Python 2.x, isinstance(u"test", str)
will return False
. This is because the u"test"
string is of type unicode
, not str
. On the other hand, Python 3.x doesn't have the u"foo"
syntax and isinstance("test", str)
will return True
.
The Solution
To write code that works on both Python 2.x and 3.x, we need to use a more elegant solution. One way to do this is by checking the string type using the six
library, which provides compatibility utilities for writing code that works on both versions.
To install the six
library, you can use pip:
pip install six
Here's an example of how you can use the six
library to check if a variable is a string:
import six
def is_string(variable):
if six.PY2:
return isinstance(variable, basestring)
else:
return isinstance(variable, str)
In the above code, we first import the six
module. Then, we define a function called is_string
that takes a variable as an argument. Inside the function, we use the six.PY2
variable to check if we're running Python 2.x. If we are, we use isinstance(variable, basestring)
to check if the variable is a string. If we're running Python 3.x, we use isinstance(variable, str)
.
The Call-to-Action
Now that you know how to check if a variable is a string in Python 2 and 3 compatibility, put your knowledge into practice! Try using the is_string
function in your own code and see how it works. If you have any questions or other Python-related topics you'd like me to cover, leave a comment below! Happy coding! 😄🐍
(If you found this blog post helpful, consider sharing it with your friends and colleagues so they can benefit from it too!)
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
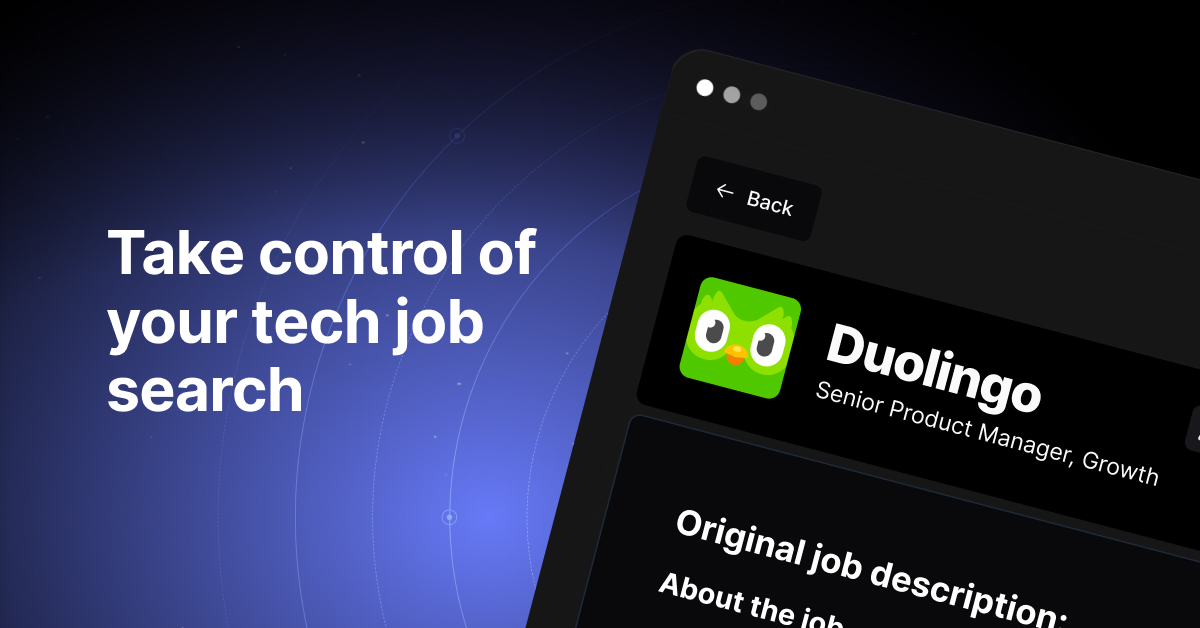