How to check if type of a variable is string?
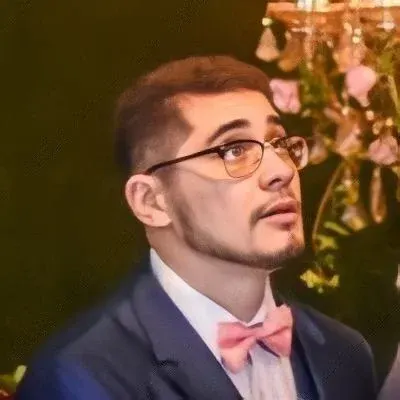
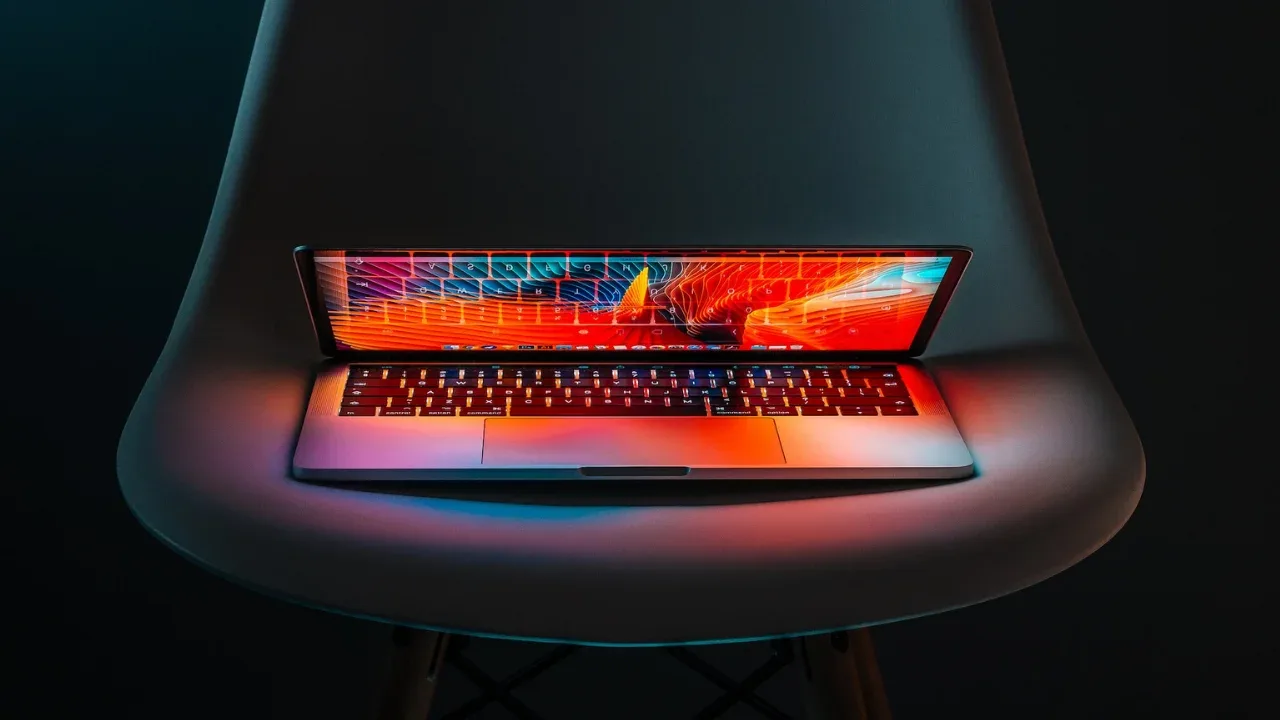
🖊️ Blog Post: How to Check if the Type of a Variable is a String?
Introduction
Have you ever found yourself wondering if a variable you're working with in Python is of type "string"? If so, you're not alone! Many developers encounter this question when working with different data types in their code. In this blog post, we'll explore this common issue and provide you with easy solutions to confidently determine the type of a variable. So let's dive in! 💻🔍
Understanding the Challenge
Before we jump into the solutions, let's understand the challenge at hand. The question posed is whether there is a way to check if the type of a variable in Python is a string, similar to how we can use the isinstance()
function to check if a variable is an integer.
Solution 1: Using the type()
Function
The type()
function in Python allows us to determine the type of a variable. This function returns the type of an object, which could be a string, integer, boolean, or any other data type.
To check if a variable is of type string, we can simply compare the result of type(variable)
with the str
type. Here's an example:
x = "Hello, World!"
if type(x) == str:
print("The variable is of type string.")
else:
print("The variable is not of type string.")
In the code snippet above, we compare the result of type(x)
with the str
type. If they match, we print a confirmation message that the variable is indeed a string. Otherwise, we print a message indicating that it is not.
Solution 2: Using the isinstance()
Function
While the type()
function is useful, Python provides us with an even more convenient method to check the type of a variable: the isinstance()
function.
The isinstance()
function allows us to check if a variable is an instance of a specific class or type. In the case of checking if a variable is a string, we can use the isinstance()
function as follows:
x = "Hello, World!"
if isinstance(x, str):
print("The variable is of type string.")
else:
print("The variable is not of type string.")
Here, we pass the variable x
as the first argument, followed by the str
type as the second argument to the isinstance()
function. If the variable is of type string, we print the corresponding message; otherwise, we print a message stating otherwise.
Conclusion
Checking the type of a variable in Python can be done using either the type()
function or the isinstance()
function. Both approaches provide simple and reliable solutions to determine if a variable is of type string.
By understanding and implementing these solutions, you can confidently check for string types within your code and ensure proper handling of different data types. So go ahead and give it a try! 🚀
Call to Action
Now that you have learned efficient ways to check if a variable is of type string, why not put your new knowledge into practice? Open your favorite Python IDE and try checking the types of different variables in your own code! Share your findings in the comments below and let's keep the discussion going. Happy coding! 💡💬
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
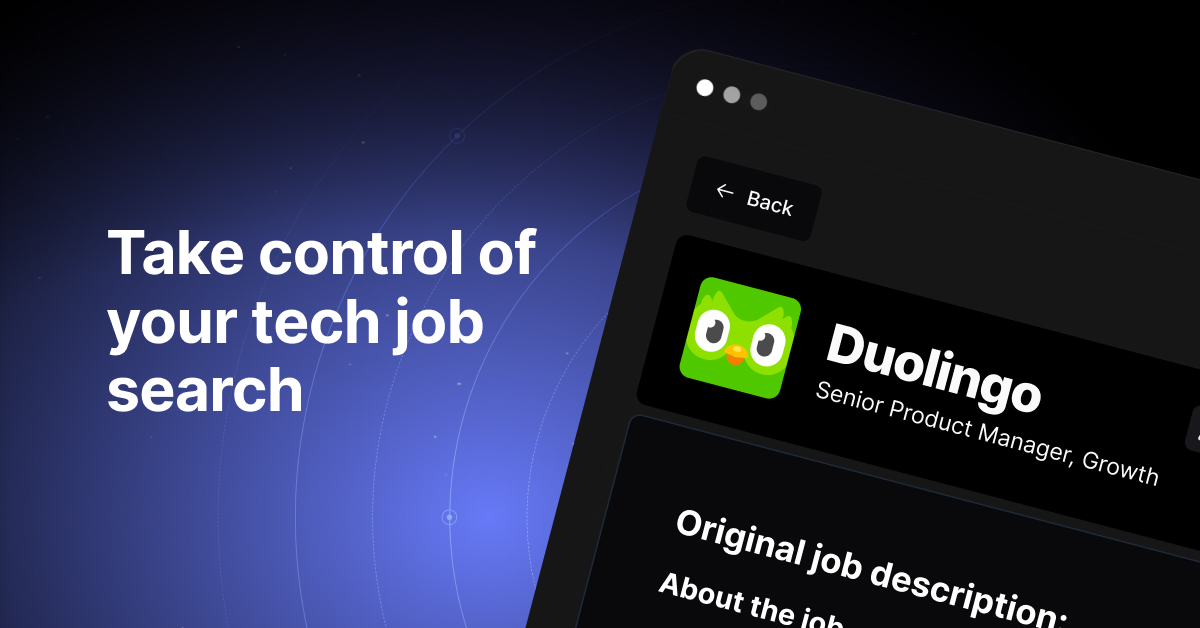