How to check if the string is empty in Python?
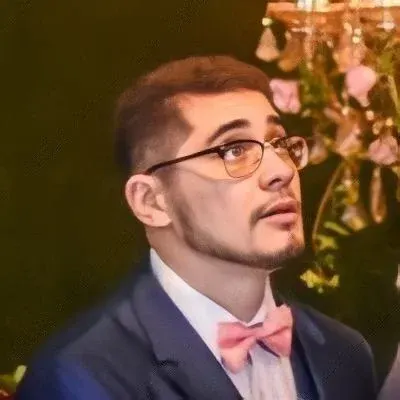
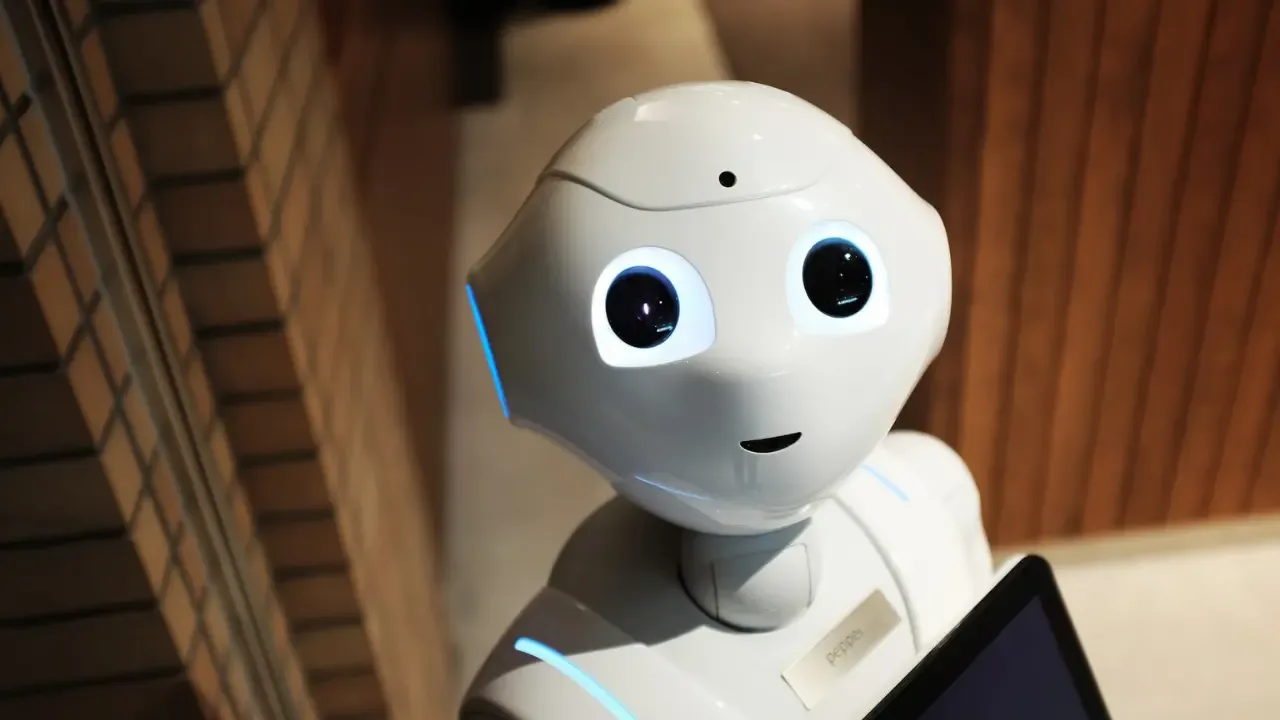
How to Check if the String is Empty in Python
Hey there, Pythonistas! 😎
Have you ever found yourself wondering how to check if a string is empty in Python? 🤔 Well, worry no more! In this blog post, we will explore easy solutions to this common problem. We'll also discuss the best practices for checking empty strings with elegance and style. 💪
The Empty String Conundrum
Let's start by addressing the question raised in the context above. Unfortunately, Python doesn't have a specific string.empty
variable like in some other languages. But fear not! There are other ways to tackle this challenge. 🚀
Solution 1: Using Conditional Statements
One simple approach is to use conditional statements to determine if a string is empty. You can do this by checking if the length of the string is equal to zero. Here's an example:
my_string = "Hello, World!"
if len(my_string) == 0:
print("The string is empty!")
else:
print("The string is not empty!")
In this case, if the length of my_string
is zero, it means the string is empty. Otherwise, it contains some characters.
Solution 2: Leveraging Truthiness
Python treats empty strings as "falsy" values. This means that if you use a string in a boolean context, an empty string will be evaluated as False
. On the other hand, a non-empty string will be evaluated as True
. Check out this snippet:
my_string = ""
if not my_string:
print("The string is empty!")
else:
print("The string is not empty!")
In this example, we use the not
operator to check if the string is falsy, indicating that it's empty.
Solution 3: Using the str.strip
Method
The str.strip
method is handy for removing leading and trailing whitespace from a string. By applying it to an empty string, you can determine if the string contains only whitespace characters or is truly empty. Take a look:
my_string = " "
if not my_string.strip():
print("The string is empty or contains only whitespace!")
else:
print("The string is not empty!")
This solution helps detect strings with whitespace that may look empty but aren't.
The Elegant Path
Now, let's address the concern raised in the context about hard coding ""
every time we need to check for an empty string. While it's a valid concern, sometimes it's necessary to be explicit for clarity purposes. However, if you frequently encounter this scenario, you can define a helper variable like EMPTY_STRING = ""
to improve code readability. 📝
Wrapping Up
You've learned various ways to check if a string is empty in Python. Whether you prefer using conditional statements, leveraging truthiness, or using the str.strip
method, you now have the tools to tackle this common challenge with grace and ease. 😃
So, what's your favorite way to check for empty strings? Let us know in the comments below! And don't forget to share this article with your fellow Python enthusiasts. Happy coding! 🐍✨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
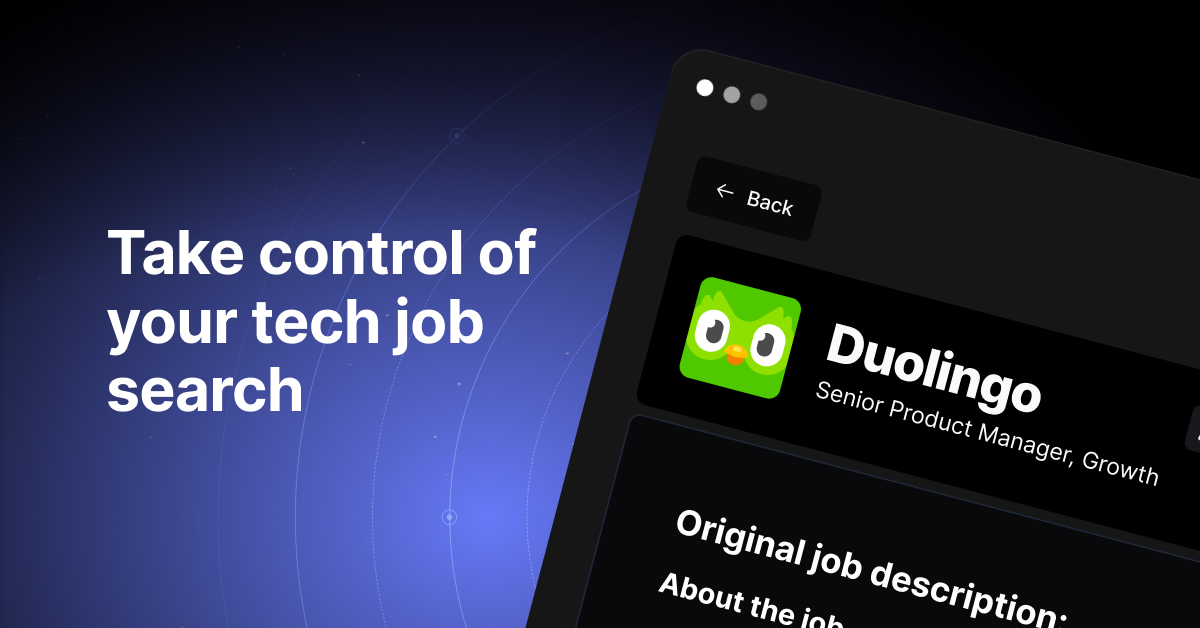