How to check if any value is NaN in a Pandas DataFrame
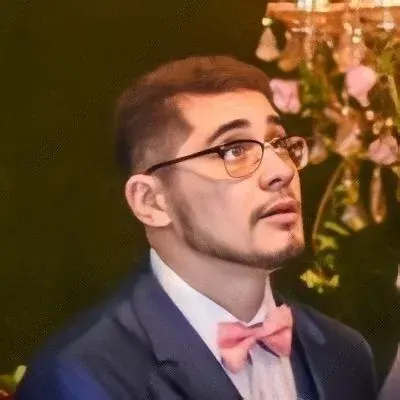
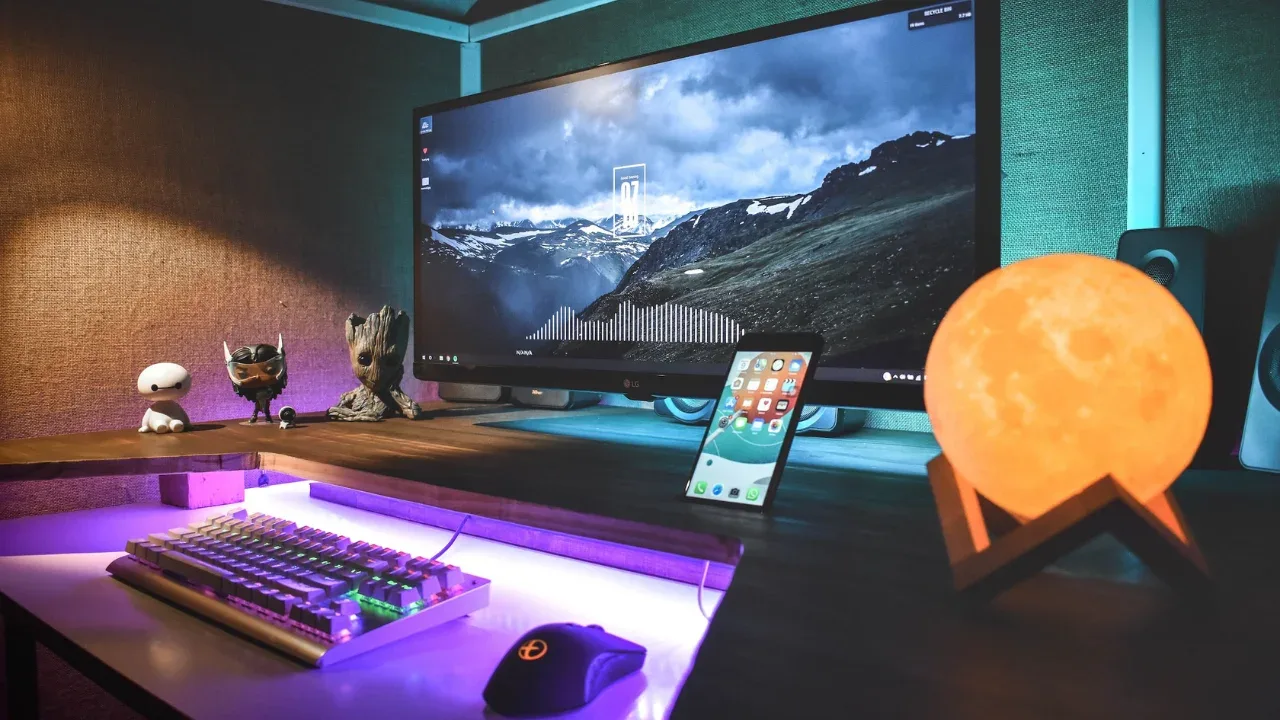
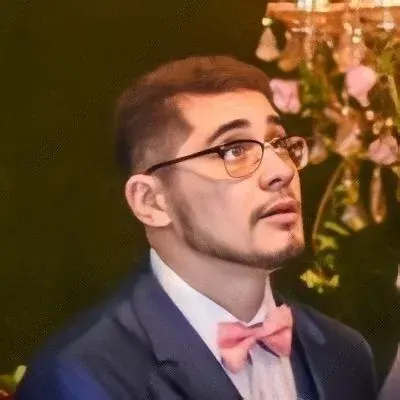
📢📝 Blog Post: How to Check if Any Value is NaN in a Pandas DataFrame
Hey there tech enthusiasts! 👋 Are you struggling with checking for NaN values in a Pandas DataFrame? Don't worry, you're not alone! 🤔 In this blog post, we'll explore common issues and provide easy solutions to help you tackle this problem like a pro. Let's get started! 🚀
Understanding the Problem
So, you have a DataFrame in Python Pandas and you want to check if it contains any NaN values. 💡 This can be useful in various scenarios, like data cleaning or ensuring data integrity. You might have come across the pd.isnan()
function, but it returns a DataFrame of booleans for each element, which doesn't directly solve your problem. 😞
Easy Solutions
Fear not! 🙌 We have a few simple solutions that will make your life easier. Let's dive into each one:
Solution 1: df.isnull()
Method
The isnull()
method is your go-to solution! 🙌 This method returns a boolean DataFrame highlighting the NaN values in your DataFrame. Here's an example:
import pandas as pd
# Create a sample DataFrame
data = {'A': [1, 2, np.nan], 'B': [4, np.nan, 6], 'C': [np.nan, 8, 9]}
df = pd.DataFrame(data)
# Check if any value is NaN
is_nan = df.isnull().values.any()
print(is_nan)
In this example, is_null
will be True
if there's at least one NaN value in the DataFrame, and False
otherwise. 🎉
Solution 2: df.isnull().sum().sum()
Function
An alternative solution is to use the sum()
function twice to count the total number of NaN values in your DataFrame. Like this:
import pandas as pd
# Create a sample DataFrame
data = {'A': [1, 2, np.nan], 'B': [4, np.nan, 6], 'C': [np.nan, 8, 9]}
df = pd.DataFrame(data)
# Check if any value is NaN
nan_count = df.isnull().sum().sum()
is_nan = True if nan_count > 0 else False
print(is_nan)
By summing all the NaN values with .isnull().sum().sum()
, you'll get the total count. If it's greater than 0, you'll know there are NaN values present. 🎯
Get Engaged!
Congratulations on mastering the art of checking NaN values in a Pandas DataFrame! 🎉 Now, it's time for you to put your newfound knowledge into action. Why not try it out in your own projects or share this post with your fellow tech enthusiasts? Let's spread the word and make their lives easier too! 💪
If you have any questions or want to share your own creative solutions, drop a comment below. We'd love to hear from you! 📩
Keep coding and stay tuned for more amazing content! Happy geeking! 😄✨