How to check if an object has an attribute?
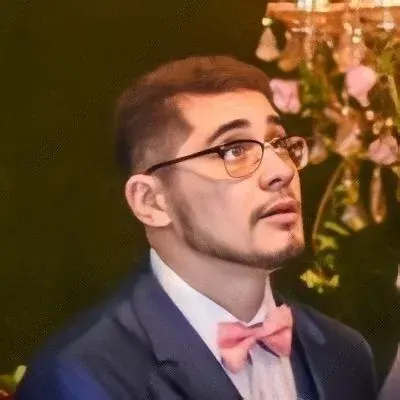
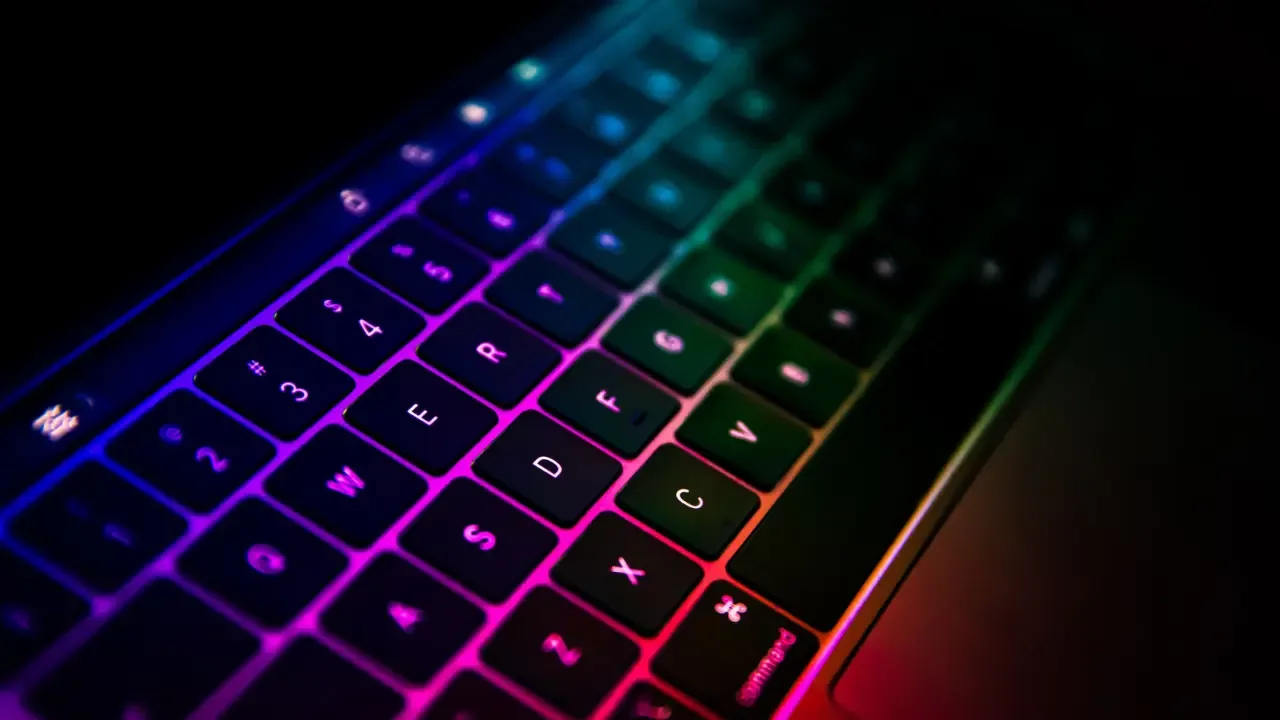
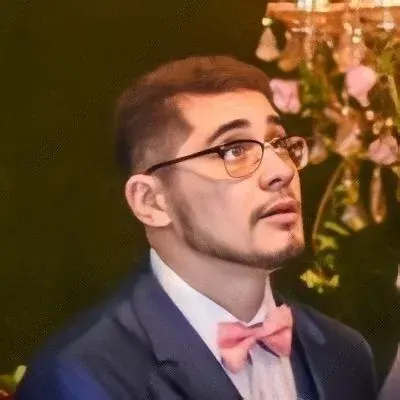
š Blog Post: How to Check if an Object has an Attribute
š Have you ever encountered a situation where you wanted to check if an object has a particular attribute before using it? š¤ It can be quite frustrating to run into an AttributeError when you try to access an attribute that doesn't exist. But worry not! In this blog post, we'll explore different methods to overcome this common issue and provide you with easy solutions. So, let's dive right in! šŖ
š Method 1: Using the hasattr()
Function
A simple and efficient way to check if an object has an attribute is by using the built-in hasattr()
function. This function takes two arguments: the object you want to check and the name of the attribute. It returns True
if the attribute exists, and False
otherwise. š§
a = SomeClass()
if hasattr(a, 'property'):
# Attribute exists!
a.property
else:
# Attribute doesn't exist
# Handle the situation accordingly
By using hasattr()
, you can easily avoid AttributeErrors and safely access the attribute when it exists. š
š Method 2: Using a Try-Except Block
Another way to handle attribute checking is by using a try-except block. You can attempt to access the attribute and catch the AttributeError
if it occurs. This way, you can gracefully handle the situation without causing your code to crash. š
a = SomeClass()
try:
a.property
# Attribute exists!
except AttributeError:
# Attribute doesn't exist
# Handle the situation accordingly
The try-except block provides you with more control and flexibility in how you handle the situation.
ā”ļø Common Issues and Considerations
āļø Remember that the hasattr()
function and the try-except block only determine if an attribute exists at runtime. They cannot guarantee that the object will always have that attribute since it can be dynamically created or modified. This is particularly relevant if you're working with external libraries or user-generated input.
š Call-to-Action: Share Your Thoughts
Now that you have learned different methods to check if an object has an attribute, it's time for you to take action! š Which method do you find most effective? Or do you have any other tricks up your sleeve? Leave a comment below and let's start a conversation! š¬
Remember, attribute checking is a common problem in programming, and sharing knowledge and experiences can benefit the entire community. So let's engage, learn from each other, and keep building amazing things together! Happy coding! šš»