How to check if a user is logged in (how to properly use user.is_authenticated)?
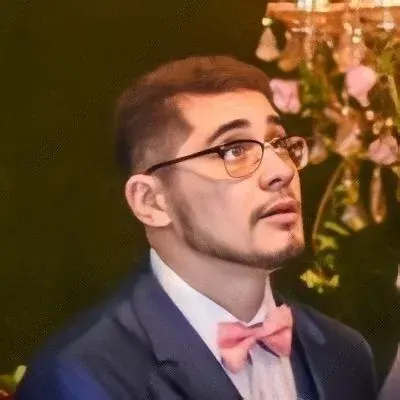
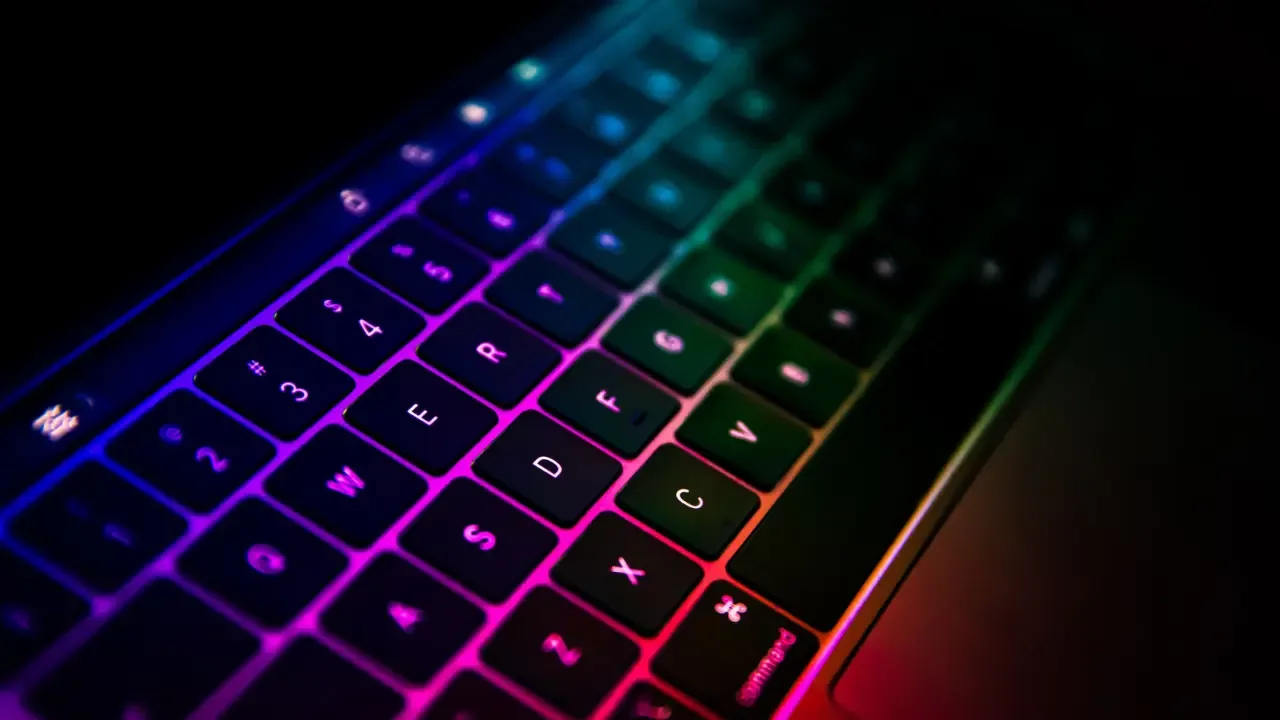
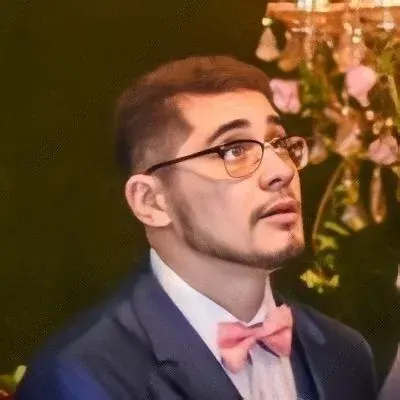
How to Properly Check if a User is Logged in (🔍🔐)
Are you trying to check if a user is logged in on your website but having trouble getting the right response? You're not alone! Many developers struggle with properly using the user.is_authenticated
attribute. But don't worry, we've got your back! In this blog post, we'll walk you through the common issues and provide easy solutions to ensure you get the correct results.
Understanding the Problem (😕❓)
Before we dive into the solutions, let's understand the problem at hand. The user.is_authenticated
attribute is a boolean value that tells you whether a user is authenticated or not. It should return True
if the user is logged in and False
if they are not. However, sometimes developers encounter unexpected results, like receiving an empty response (>
), even when they know the user is logged in.
Common Issues and Solutions (💡🔧)
Issue #1: Lack of Authentication Middleware (🚨🔌)
One common reason for incorrect responses is the lack of authentication middleware. This middleware is responsible for authenticating requests and associating the user with the request object. Without it, Django won't be able to track user authentication.
To ensure the authentication middleware is enabled, make sure you have the following line in your settings.py
file:
MIDDLEWARE = [
# other middleware classes...
'django.contrib.auth.middleware.AuthenticationMiddleware',
# other middleware classes...
]
If the line is missing, add it, and restart your server to see if the issue is resolved.
Issue #2: Incorrect Template Context (👀📂)
Another issue that can lead to incorrect responses is not passing the request
object to the template context. The user.is_authenticated
attribute depends on having access to the request
object, so if it's missing, you won't get the desired results.
To ensure the request
object is available in your template, update your view function or class-based view as follows:
from django.shortcuts import render
def my_view(request):
return render(request, 'my_template.html', {'request': request})
By explicitly passing the request
object to the template context, you give it access to the necessary information for user authentication.
Issue #3: Caching or Stale Sessions (🔒🔄)
Sometimes, caching or stale sessions can cause the user.is_authenticated
attribute to return incorrect results. Caching can store previous authentication states, leading to outdated results.
To ensure you are always retrieving the latest authentication state, try clearing your cache or refreshing your session. You can also try logging out and logging back in to create a fresh session.
Issue #4: Incorrect User Model (👤❌)
If you've extended Django's default user model or are using a custom user model, you may experience issues with the user.is_authenticated
attribute. Make sure that your custom user model is properly set up and that you are referencing it correctly in your code.
Check your AUTH_USER_MODEL
setting in your settings.py
file. It should point to your custom user model:
AUTH_USER_MODEL = 'myapp.CustomUser'
Replace 'myapp.CustomUser'
with the correct path to your custom user model.
Test Your Authentication (🔍✅)
Once you've implemented the solutions, it's time to test your authentication. Make sure to log in with a valid user account and access a page that requires authentication. Then, in your template or view, do a simple check using:
{% if request.user.is_authenticated %}
<!-- User is logged in -->
{% else %}
<!-- User is not logged in -->
{% endif %}
This check should now correctly display the corresponding message based on the user's authentication status.
Share Your Success! (🚀🎉)
Congratulations! You've successfully learned the common issues and solutions related to properly checking if a user is logged in. Now, go ahead and share your success story with us. Did you encounter any other challenges along the way? What other authentication-related topics would you like us to cover in future blog posts? Let us know in the comments section below! Together, we can make authentication easier for everyone.