How to check if a string is a substring of items in a list of strings
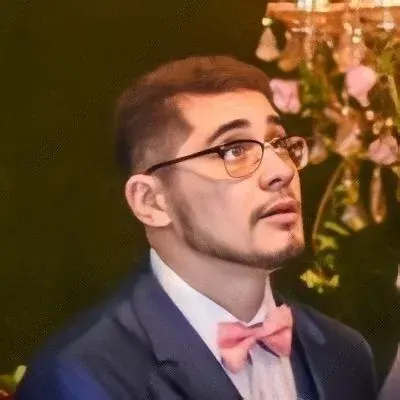
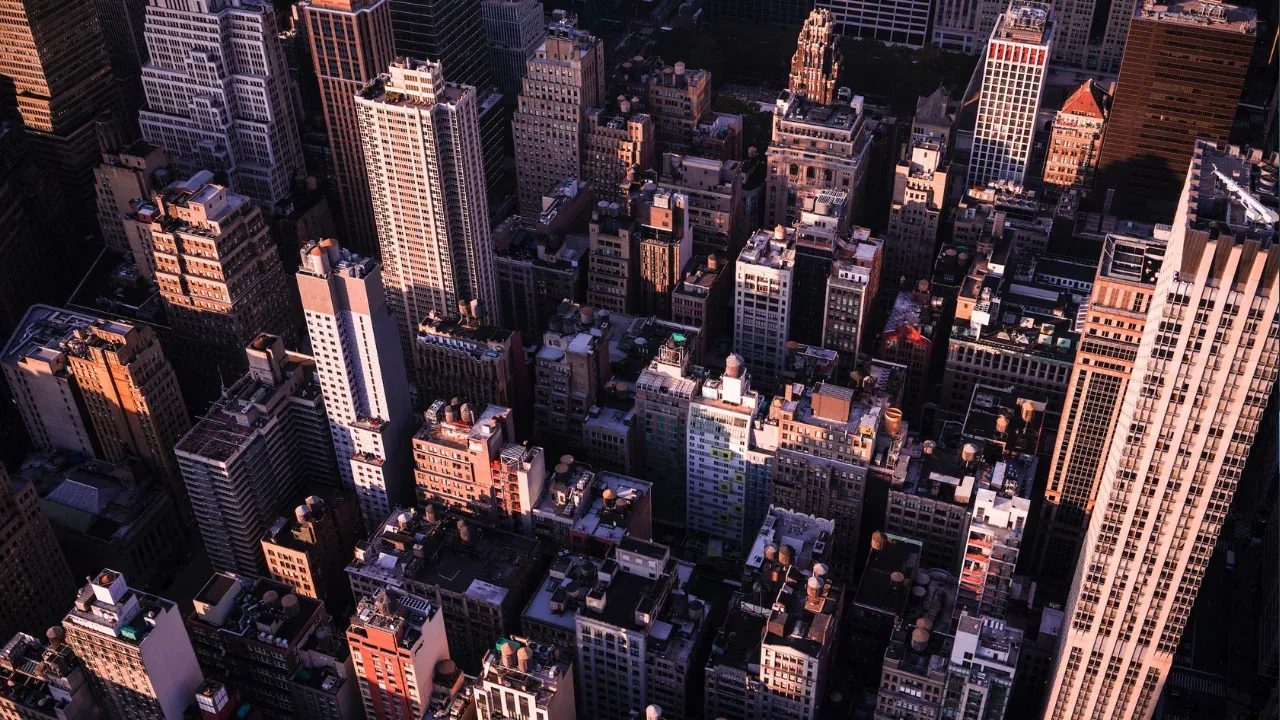
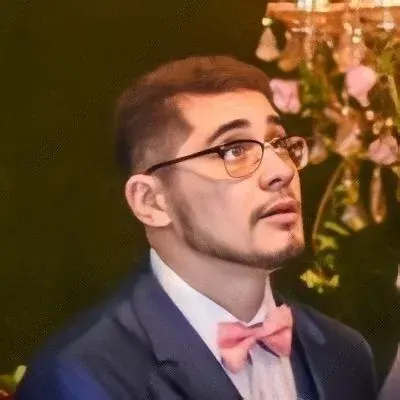
How to Check if a String is a Substring of Items in a List of Strings
So, you have a list of strings and you want to check if a specific string is a substring of any of the items in the list. 🧐
Let's consider an example to make things clearer. 🌟
xs = ['abc-123', 'def-456', 'ghi-789', 'abc-456']
In this case, we want to check if the string 'abc'
is a substring of any item in the list xs
. However, simply using the in
operator like this won't give us the desired result:
if 'abc' in xs:
# This won't work as expected! 😟
print("Substring found!")
The above code will not detect substrings like 'abc-123'
and 'abc-456'
. 😕
The Issue
The problem with the solution above is that it only checks if the exact string 'abc'
exists in the list xs
. It doesn't consider any substrings which contain the desired string. 😔
The Solution
To overcome this issue and find substrings, we need to iterate through each string in the list and explicitly check if our desired string is a substring of that particular string. Let's see how to do this! 💡
substring = 'abc'
found = False
for item in xs:
if substring in item:
found = True
break # No need to continue searching, we found a match!
if found:
print("Substring found!")
In the code above, we initialize a boolean variable found
as False
. Then, we iterate through each string in the list xs
. For every string, we test if our desired substring is present using the in
operator. If a match is found, we set found
to True
and break out of the loop because we don't need to continue searching.
Finally, we check the value of found
. If it is True
, we print the message "Substring found!". Otherwise, we can assume that our desired substring is not present in any of the strings in the list. 😞
Be Mindful of Case Sensitivity!
It is important to note that the above solution is case sensitive, meaning it will only detect substrings in strings that have the exact case as our desired substring. For example, if our desired substring is 'abc'
, it will not match with 'ABC-123'
or 'AbC-456'
.
If case sensitivity is a concern for your use case, you can convert both the substring and the strings in the list to lowercase (or uppercase) before performing the check. This can be done using the lower()
(or upper()
) string method. Here's an example: 🔄
substring = 'abc'
found = False
for item in xs:
if substring.lower() in item.lower():
found = True
break
if found:
print("Substring found!")
In this modified code, we convert both the substring and the strings in the list to lowercase using the lower()
method. This ensures that we find matches regardless of the case.
Conclusion
To check if a string is a substring of items in a list of strings, you need to iterate through each string in the list and explicitly check for the desired substring. Remember to be aware of case sensitivity and adjust your code accordingly if needed.
Now that you know how to solve this problem, go ahead and give it a try in your own projects! 👍 And don't forget to share your experience or any further questions in the comments below! Let's learn and grow together! 💪✨