How To Check If A Key in **kwargs Exists?
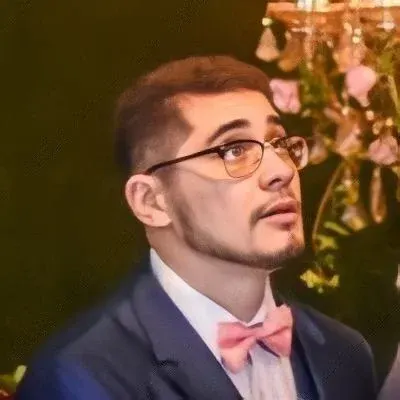
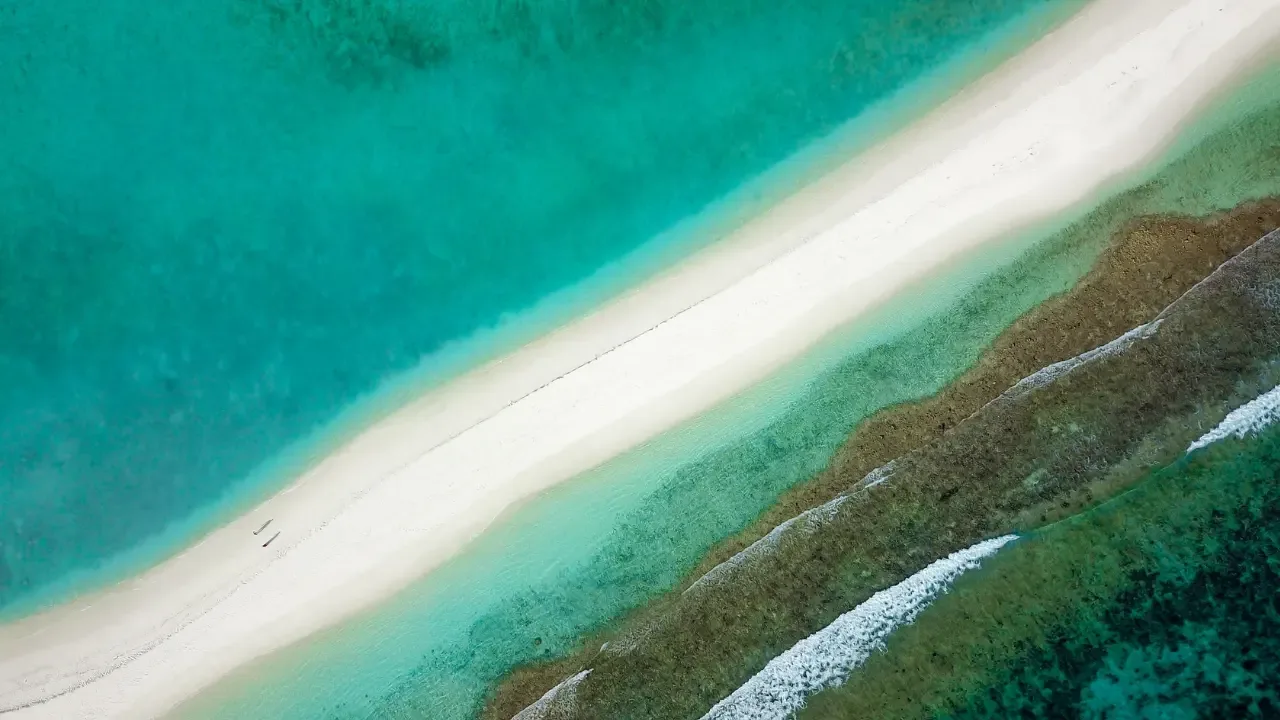
How To Check If A Key in **kwargs Exists?
So, you're working with **kwargs in Python and you're trying to figure out if a specific key exists in it. It can be a bit confusing because **kwargs, unlike regular variables, don't play by the same rules. But don't worry, we're here to help you understand why your code isn't working and show you an easy solution to this problem. Let's dive in!
The Issue
Let's take a look at the code you provided:
if kwargs['errormessage']:
print("It exists")
You might expect this code to check if the key 'errormessage'
exists in **kwargs, and if it does, print "It exists". However, if the key doesn't exist, you'll encounter a KeyError
and your code will crash. Ouch!
The Explanation
The reason this code doesn't work as expected is because **kwargs is treated as a dictionary in Python. When you try to access a key in a dictionary using square brackets ([]
), Python assumes that the key exists. If it doesn't, you'll get a KeyError
.
The Solution
To avoid the KeyError
and safely check if a key exists in **kwargs, you can use the get()
method of dictionaries. Here's how you can modify your code:
if kwargs.get('errormessage'):
print("It exists")
By using kwargs.get('errormessage')
, you're asking Python to retrieve the value associated with the key 'errormessage'
from **kwargs. If the key doesn't exist, kwargs.get()
gracefully returns None
, allowing you to handle the case where the key doesn't exist without causing your code to crash. Awesome, right?
A More Pythonic Solution
Now, some of you might be wondering if there's a more Pythonic way to check if a key exists in **kwargs. Well, you're in luck! Python provides a clean and concise way to accomplish this using the in
operator. Take a look:
if 'errormessage' in kwargs:
print("Yeah, it's here")
This code checks if the key 'errormessage'
is present in **kwargs. If it is, "Yeah, it's here" is printed. If it's not, nothing happens. Simple, elegant, and Pythonic!
The Conclusion
You now know how to check if a key exists in **kwargs without running into errors. You've learned about the differences between accessing keys in regular variables and **kwargs, and you've seen two different solutions to the problem.
The next time you find yourself working with **kwargs and need to check for the existence of a key, remember to use kwargs.get()
or the in
operator. They will save you from potential headaches and help you write more robust code.
Now go out there and conquer your coding challenges with confidence! 🚀
What's your favorite way to handle **kwargs in Python? Share your thoughts in the comments below and let's start a discussion! Don't forget to subscribe to our newsletter for more helpful tips and tricks. Happy coding! 😄
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
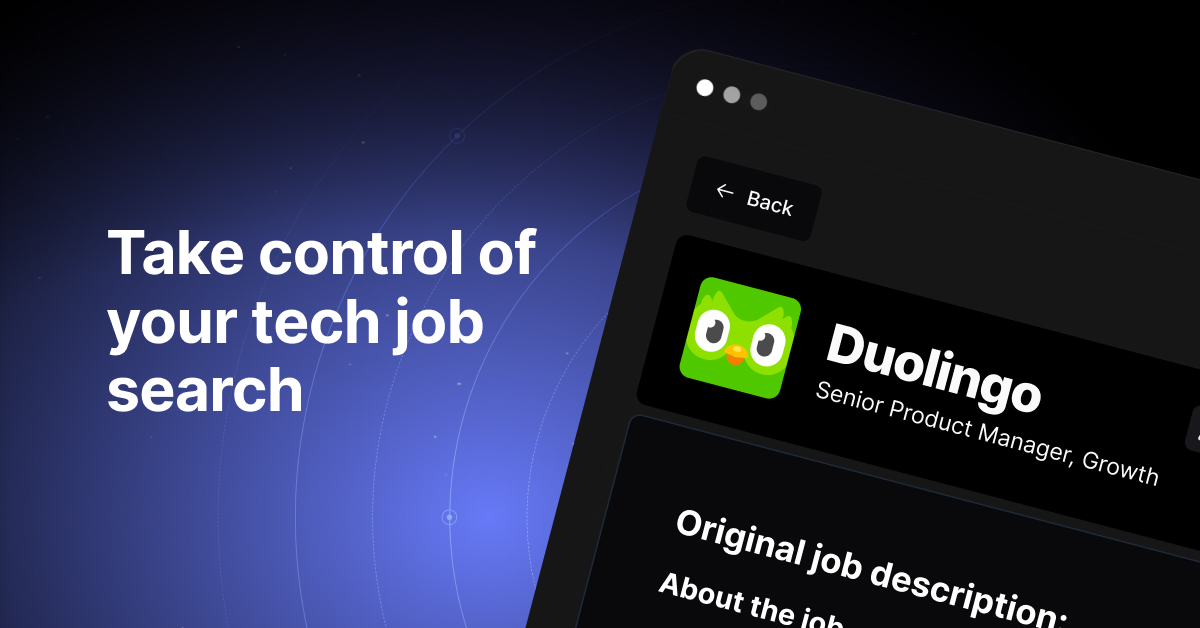