How to check if a column exists in Pandas
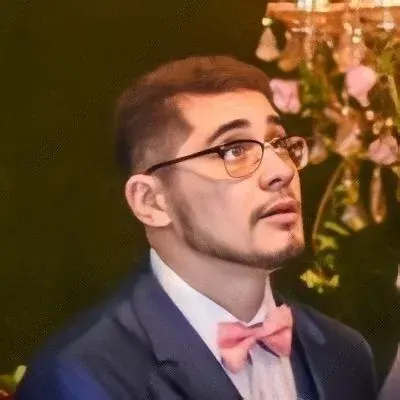
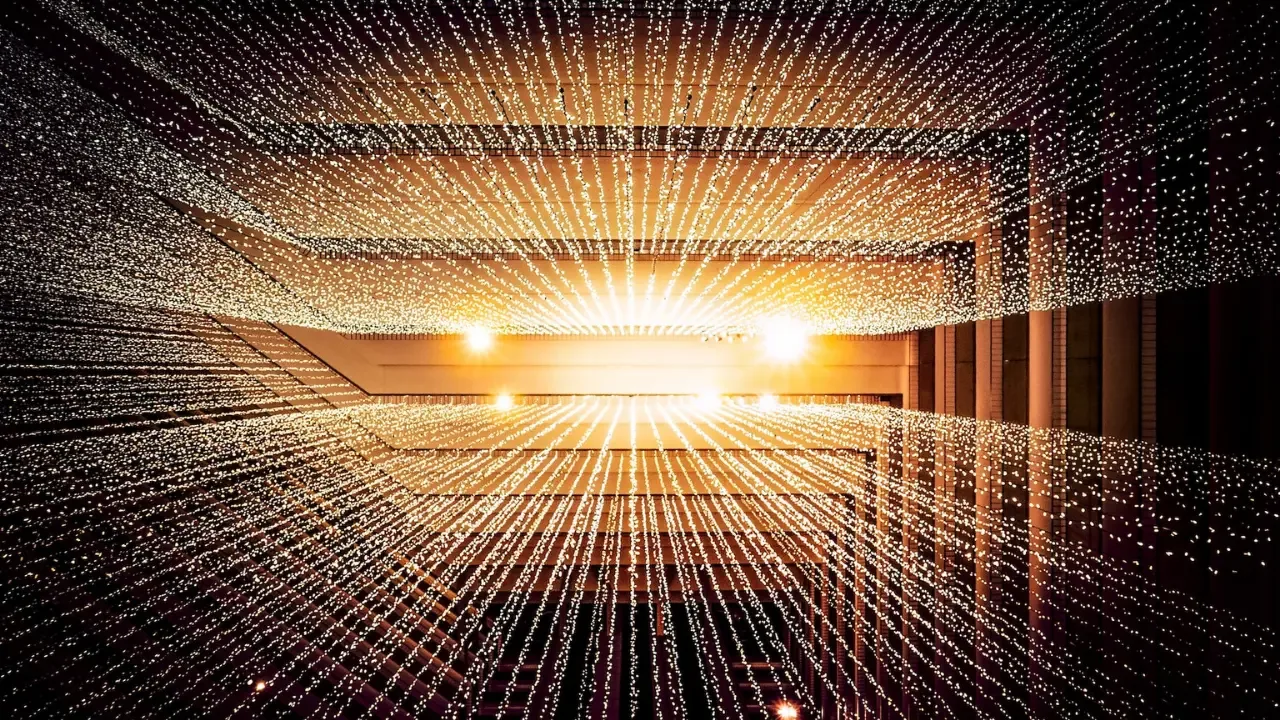
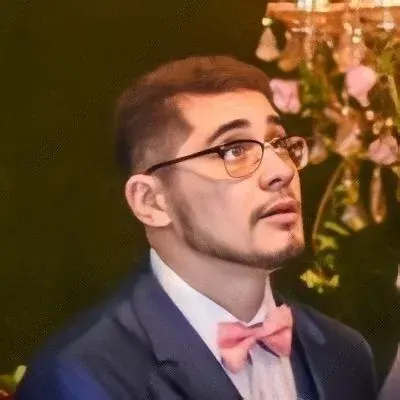
How to Check if a Column Exists in Pandas 💡
Are you wondering how to determine if a specific column exists in a Pandas DataFrame? Whether you are performing data analysis or preparing your data for machine learning, it's crucial to ensure that the column you need is present in your DataFrame. In this blog post, we will explore common issues around this problem and provide you with easy solutions to check if a column exists in Pandas.
Let's consider the following DataFrame as an example:
A B C
0 3 40 100
1 6 30 200
Our objective is to check if the column "A" exists so that we can proceed with computing the sum of "A" and "C" and store it in a new column called "sum." But what if "A" doesn't exist, and we want to compute the sum of "B" and "C" instead? 🤔
Solution 1: Using the in
Operator 🕵️♀️
One straightforward way to check if a column exists in a Pandas DataFrame is by using the in
operator with the DataFrame's columns
attribute. Here is how you can do it:
if 'A' in df.columns:
df['sum'] = df['A'] + df['C']
else:
df['sum'] = df['B'] + df['C']
In this code snippet, we check if 'A' exists in the DataFrame's columns. If it does, we compute the sum of 'A' and 'C' and store it in the 'sum' column. Otherwise, we compute the sum of 'B' and 'C'.
Solution 2: Using the try-except
Block 🚀
Another way to handle this problem is by using a try-except
block. We can try to access the 'A' column and perform the computation. If an exception occurs, we catch it and then proceed with the alternative computation.
try:
df['sum'] = df['A'] + df['C']
except KeyError:
df['sum'] = df['B'] + df['C']
This approach is beneficial when you prefer handling exceptions rather than checking membership explicitly.
Solution 3: Using the pandas.api.types
Module ✅
Pandas provides the pandas.api.types
module, which offers additional functionality for solving this problem. In particular, the is_numeric_dtype
function can help us verify if a column exists, and if it does, whether it contains numeric values.
import pandas.api.types as pd_types
if 'A' in df.columns and pd_types.is_numeric_dtype(df['A']):
df['sum'] = df['A'] + df['C']
else:
df['sum'] = df['B'] + df['C']
By combining column existence and numeric type checks, you can have more control over the data manipulation process.
Conclusion and Call-to-Action 📢
Now that you have learned multiple ways to check if a column exists in a Pandas DataFrame, you can confidently handle this common issue in your data analysis projects. Next time you encounter a similar problem, remember the solutions we discussed:
Use the
in
operator with the DataFrame'scolumns
attribute.Employ a
try-except
block to handle potential KeyError exceptions.Utilize the
is_numeric_dtype
function from thepandas.api.types
module.
Do you have any other Pandas-related questions or data analysis challenges? Let us know in the comments section below. 📝 We are here to assist you!
Happy coding! 🚀