How to check for NaN values
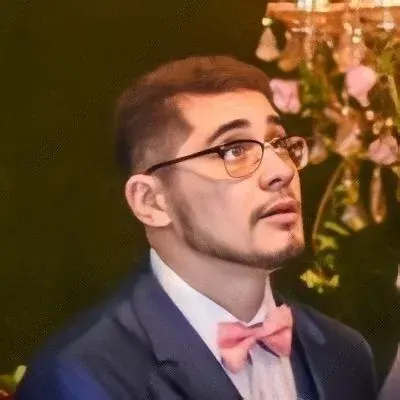
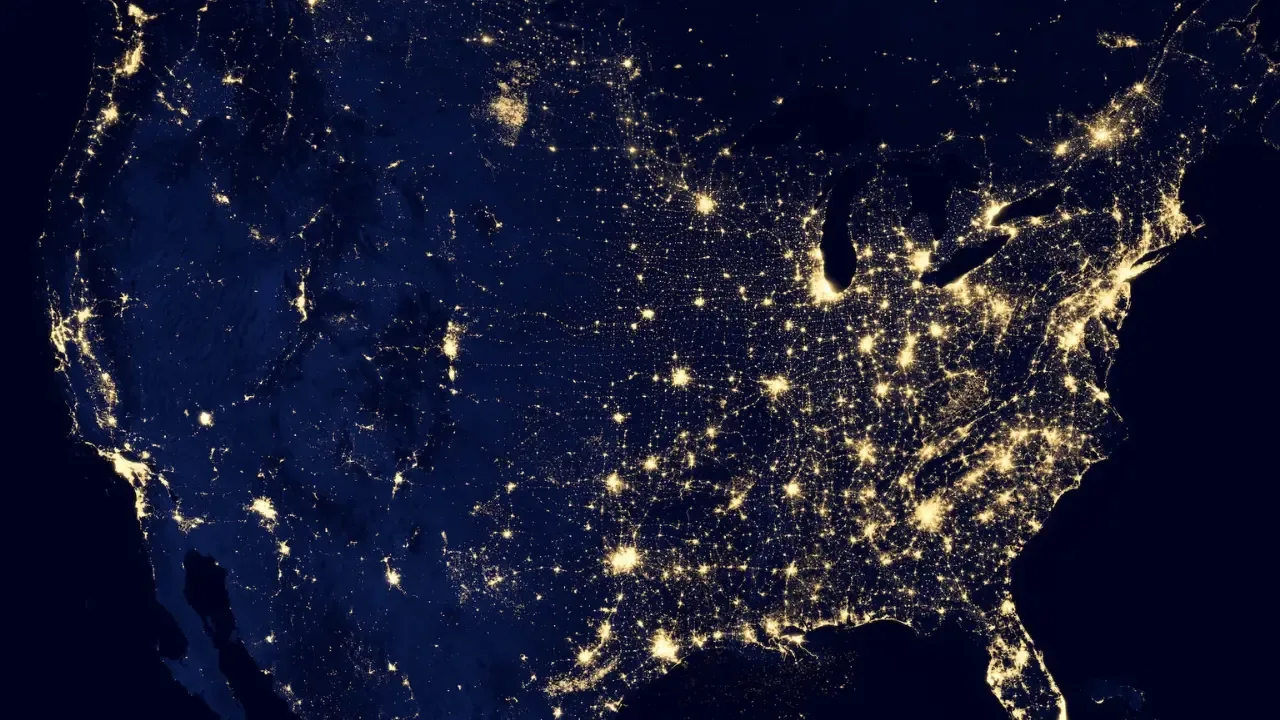
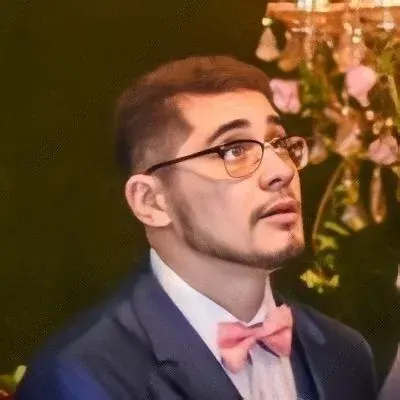
šµļøāāļø Unmask the Mysterious NaN: How to Check for NaN Values šµļøāāļø
š Hey there, tech-savvy reader! š
Have you ever encountered the cryptic "NaN" while working with numbers? š¤ Don't worry; you're not alone! NaN stands for "not a number," and it often pops up when dealing with complex calculations or missing data. But how can you check for NaN values like a true tech detective? šµļøāāļø Let's dive in and crack this case wide open!
š Understanding the NaN Phenomenon
To better grasp NaN, let's start with a quick explanation. In Python, the expression float('nan')
represents NaN. It's a special value that indicates missing or undefined numerical values. Detecting NaN can be vital when working with large datasets, statistical analysis, or any situation where missing values could skew your results.
šµļøāāļø Investigating the Case: How to Check for NaN Values in Python
Now that we understand what NaN is, it's time to equip ourselves with the knowledge to detect it. We'll explore two methods: using the math.isnan()
function and leveraging NumPy's np.isnan()
function. Let's put on our detective hats and begin!
Method 1: The math.isnan()
Function
Python's built-in math
library provides a handy isnan()
function that can detect NaN values. Here's an example:
import math
result = math.isnan(suspect_value)
if result:
print("The value is NaN!")
else:
print("All clear - not NaN!")
In this example, suspect_value
represents the value you want to investigate. The math.isnan()
function will return True
if the value is NaN, allowing you to take appropriate action.
Method 2: NumPy's np.isnan()
Function
If you're working with arrays or matrices, the popular NumPy library has got your back with its isnan()
function. Here's how you can utilize it:
import numpy as np
arr = np.array([3.14, np.nan, 42])
result = np.isnan(arr)
print(result)
In this code snippet, we create an array containing numeric values, including a NaN value. By using np.isnan()
on the array, we obtain a boolean array indicating whether each element is NaN. Perfect, isn't it?
š The Ultimate NaN Check Reference
Congratulations, fellow code sleuths! You've cracked the NaN detection case wide open. However, NaN can be tricky, sometimes hiding in unexpected places. To help you on your coding adventures, here are a few additional tips to remember:
NaN is not equal to anything, not even itself. So, using
==
to compare a value to NaN will always returnFalse
.When working with pandas DataFrames, you can conveniently use the
isnull()
function to detect NaN values.
Remember, NaN values are like elusive phantoms. Be cautious and thorough when dealing with them!
š£ Join Our Detective Squad!
There you have it, friends! You're now equipped with the knowledge to unravel the mysteries of NaN. But this is just the beginning of your tech adventures! š Keep exploring and stay curious.
š Have you ever stumbled upon NaN while working on a particular project? Share your NaN-filled experiences in the comments below! Let's connect and learn from each other.
š If you found this blog post helpful, don't forget to hit that share button! Spread the NaN detection knowledge among your fellow detectives.
Cheers to NaN-crushing victories! š
Happy coding! š»