How to change the order of DataFrame columns?
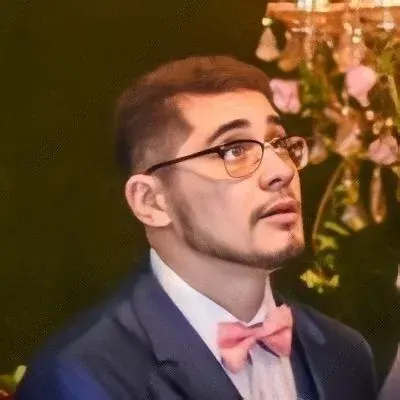
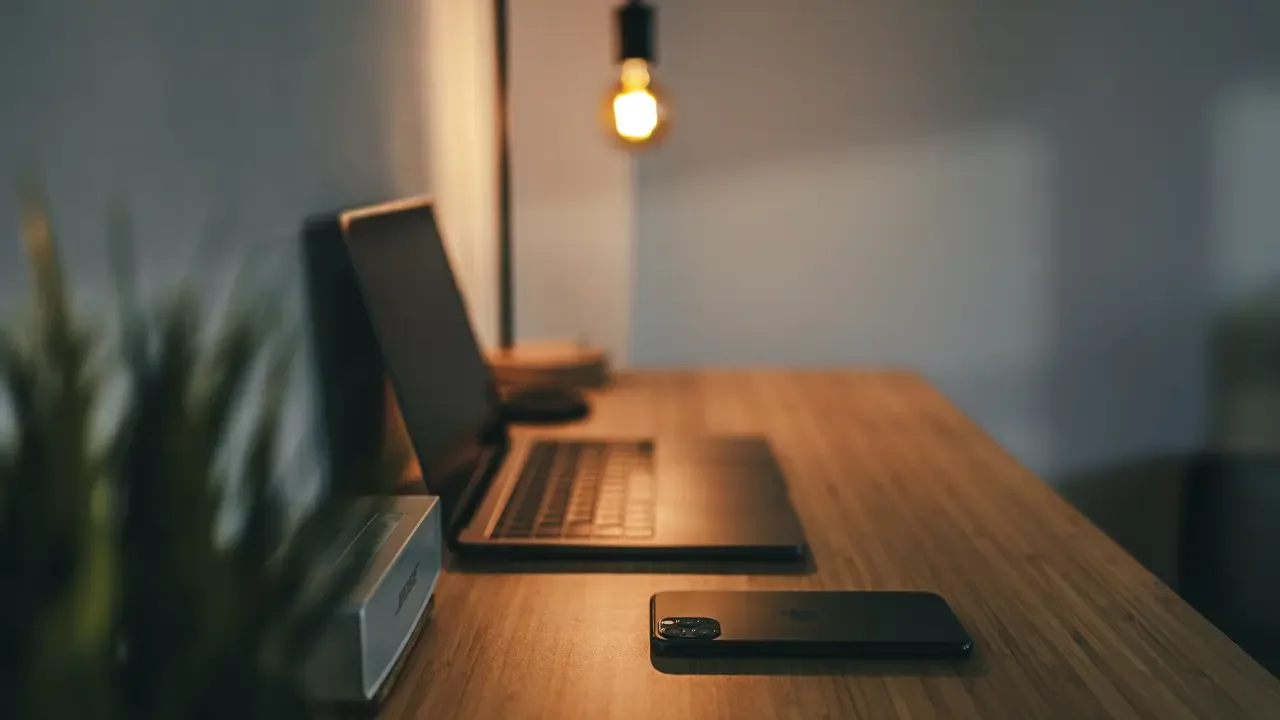
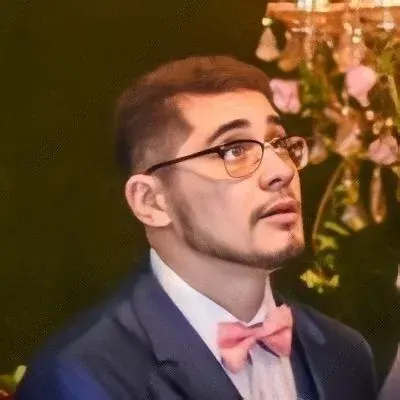
How to change the order of DataFrame columns? 🔄📊
So you've created a DataFrame and you want to change the order of the columns to make it better fit your needs. In this guide, we'll explore an easy solution to this common problem using Python's pandas library.
👩💻 Let's start with the context. Suppose you have a DataFrame called df
with the following structure:
import numpy as np
import pandas as pd
df = pd.DataFrame(np.random.rand(10, 5))
✨ Now, imagine you want to add a new column called mean
that represents the mean of each row. You can easily achieve this by assigning values to a new column:
df['mean'] = df.mean(1)
But here's the catch: you want the mean
column to be the first column in your DataFrame, while keeping the order of the other columns intact.
🔀 To reorder the columns, you can use the reindex
function provided by pandas. Here's how you can do it:
new_order = ['mean'] + [col for col in df.columns if col != 'mean']
df = df.reindex(columns=new_order)
In the example above, we create a new list called new_order
that starts with the desired column, 'mean'
, followed by all the other columns except for 'mean'
using a list comprehension. Then, we pass this new order to the reindex
function to update the DataFrame.
🎉 And just like that, your DataFrame's columns are rearranged to your liking! The mean
column now takes the first position, while the order of the remaining columns is preserved.
📝 Here's the complete code snippet for easy reference:
import numpy as np
import pandas as pd
df = pd.DataFrame(np.random.rand(10, 5))
df['mean'] = df.mean(1)
new_order = ['mean'] + [col for col in df.columns if col != 'mean']
df = df.reindex(columns=new_order)
Now you have the power to change the order of DataFrame columns effortlessly! Feel free to experiment with different column orders to suit your specific needs.
👉 Do you have any other questions about manipulating DataFrames or pandas in general? Let us know in the comments below! We're here to help you unleash the full potential of your data analysis skills. 🚀💪
Happy coding! 💻😊