How to change a string into uppercase?
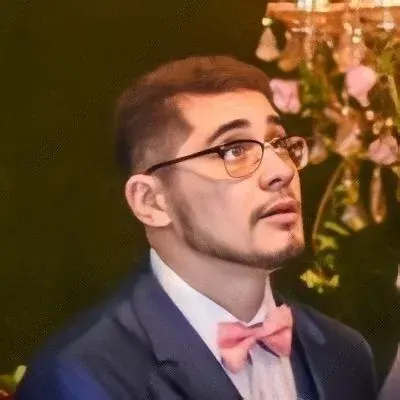
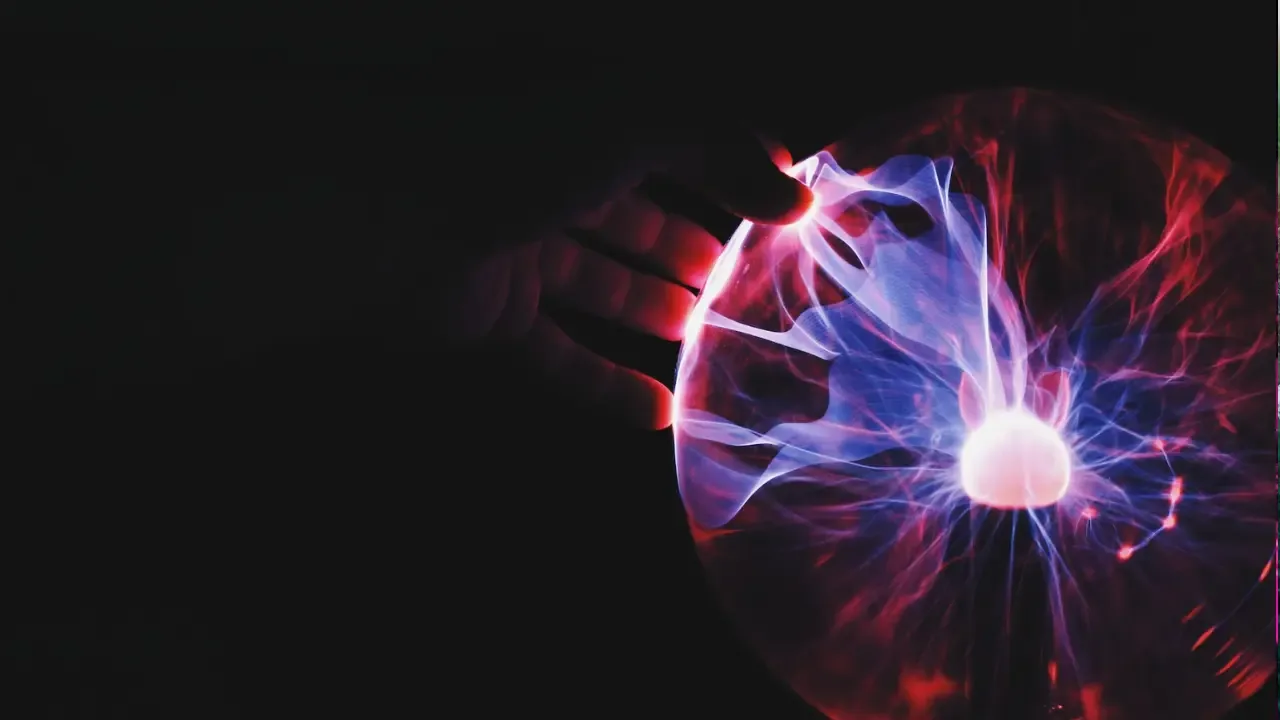
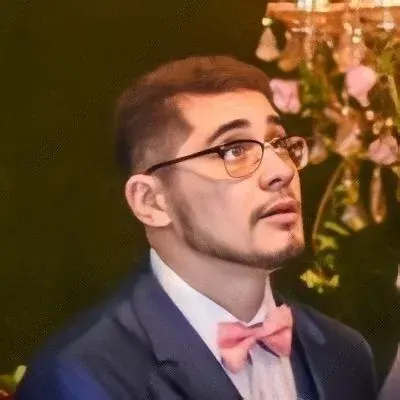
How to Change a String into Uppercase in Python?
Are you struggling with converting a string into uppercase in Python? 🤔 Don't worry, you're not alone! Many developers find this task a bit challenging at first. But fear not, because I'm here to guide you through the process. 🚀
The Problem: AttributeError on string.ascii_uppercase
Have you come across the string.ascii_uppercase
solution? It seems promising, but unfortunately, it won't work in this scenario. Let me show you why:
s = 'sdsd'
s.ascii_uppercase
When you try running this code, you'll encounter an AttributeError: 'str' object has no attribute 'ascii_uppercase'
. 😟
The Solution: Using the upper()
Method
Luckily, Python provides a simple and effective way to convert a string into uppercase: the upper()
method. 🙌
To convert a string into uppercase, you'll need to use the dot notation and call the upper()
method on your string variable. Here's how it works:
s = 'sdsd'
uppercase_s = s.upper()
print(uppercase_s)
By running this code, you'll get the desired output: SDSD
. 🎉 The upper()
method converts all alphabetic characters in a string to uppercase.
Dealing with Non-Alphabetic Characters
But what happens if your string contains non-alphabetic characters? Let's say you have a string with numbers, symbols, and spaces. Well, not to worry! The upper()
method won't affect any non-alphabetic characters. It will only convert the alphabetic characters to uppercase. Take a look at this example:
s = '123 ABC *'
uppercase_s = s.upper()
print(uppercase_s)
The output will be the same as the original string: 123 ABC *
. The upper()
method doesn't modify non-alphabetic characters.
Your Call to Action: Share Your Experience!
Now that you know how to convert a string into uppercase in Python, it's time to put your newfound knowledge to the test. Try using the upper()
method on your own strings and see what happens. Feel free to share your experience in the comments below. 👇 We would love to hear from you!
Remember, practice makes perfect! Keep coding and keep learning. Happy programming! 😄💻