How to avoid pandas creating an index in a saved csv
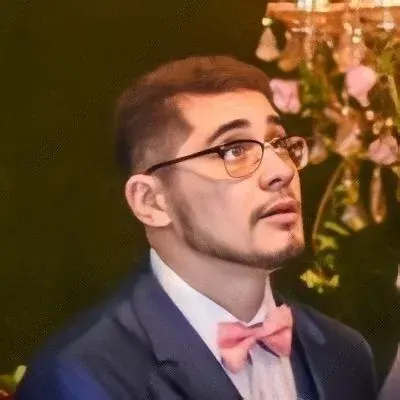
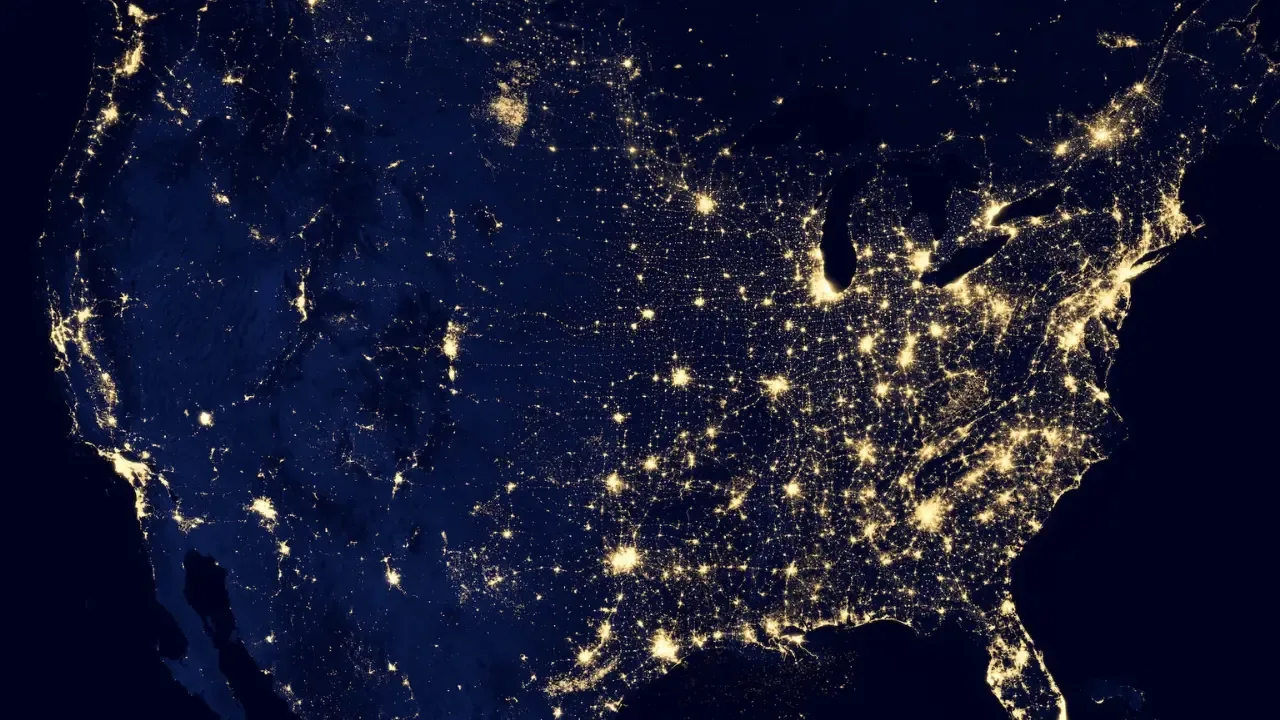
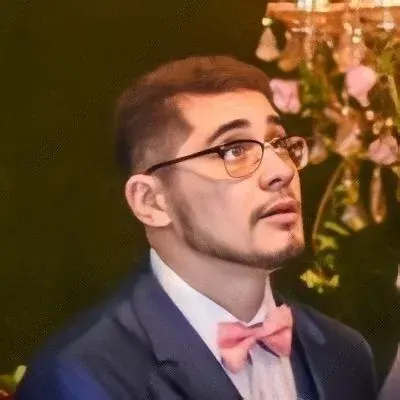
How to avoid pandas creating an index in a saved csv πΌπ
So, you were happily editing your CSV file using pandas, but when you saved it, boom! A separate column of indexes magically appeared. Not cool, right? π«
The good news is, there's a simple solution to avoid this pesky index column. Let's go through the steps together! π
The problem: Unwanted index column π€
The issue arises when you save a pandas DataFrame to a CSV file using the pd.to_csv()
function. By default, pandas adds the index column to the saved file. However, in many cases, you might not want this index column to be included.
Step 1: Import pandas π
First things first, to work with pandas, make sure you have it installed in your Python environment:
import pandas as pd
Now, let's dive into the solutions! π‘
Solution 1: Exclude the index column when reading the file βοΈ
One way to avoid the index column is to exclude it when reading the file using the pd.read_csv()
function. By setting the index_col
parameter to False
, you tell pandas not to consider any column as the index. Here's an example:
data = pd.read_csv('C:/Path to file to edit.csv', index_col=False)
By doing this, you ensure that the index column is not included when reading the file. However, remember that this solution only affects the read operation and not the subsequent write operation.
Solution 2: Exclude the index column when saving the file βοΈ
Another way to avoid the index column is by excluding it when saving the CSV file using the pd.to_csv()
function. This approach gives you more control over the output file. Simply set the index
parameter to False
to prevent the index column from being saved. Here's an example:
data.to_csv('C:/Path to save edited file.csv', index=False)
With this solution, you ensure that the index column is not included in the saved file. π
Solution 3: Use the DataFrame without an index column for editing βοΈ
If you prefer to have a DataFrame without an index column from the start, you can use the index_col
parameter when reading the file to specify which column should be treated as the index. By setting index_col
to a specific column name or the column index, you can effectively exclude the unwanted index column during the editing process. Here's an example:
data = pd.read_csv('C:/Path to file to edit.csv', index_col='Column Name')
Make sure to replace 'Column Name'
with the actual name or index of the column you want to use as the index.
Call to action: Share your solution! π’
Next time you find yourself facing the issue of a unwanted index column in your saved pandas CSV file, remember these simple solutions. You can either exclude the index column when reading the file or skip it when saving the file.
Now, it's your turn! Have you encountered this problem before or do you have any additional tips? Share your experiences and solutions in the comments below. Let's help each other out and make data manipulation a breeze! πͺπ¬