How to apply a function to two columns of Pandas dataframe
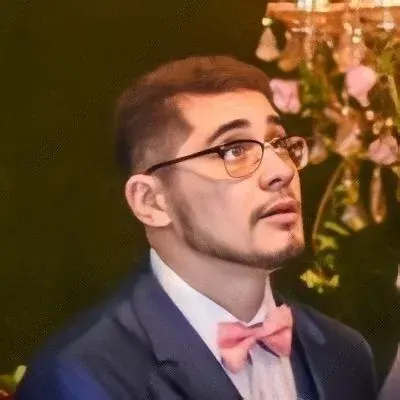
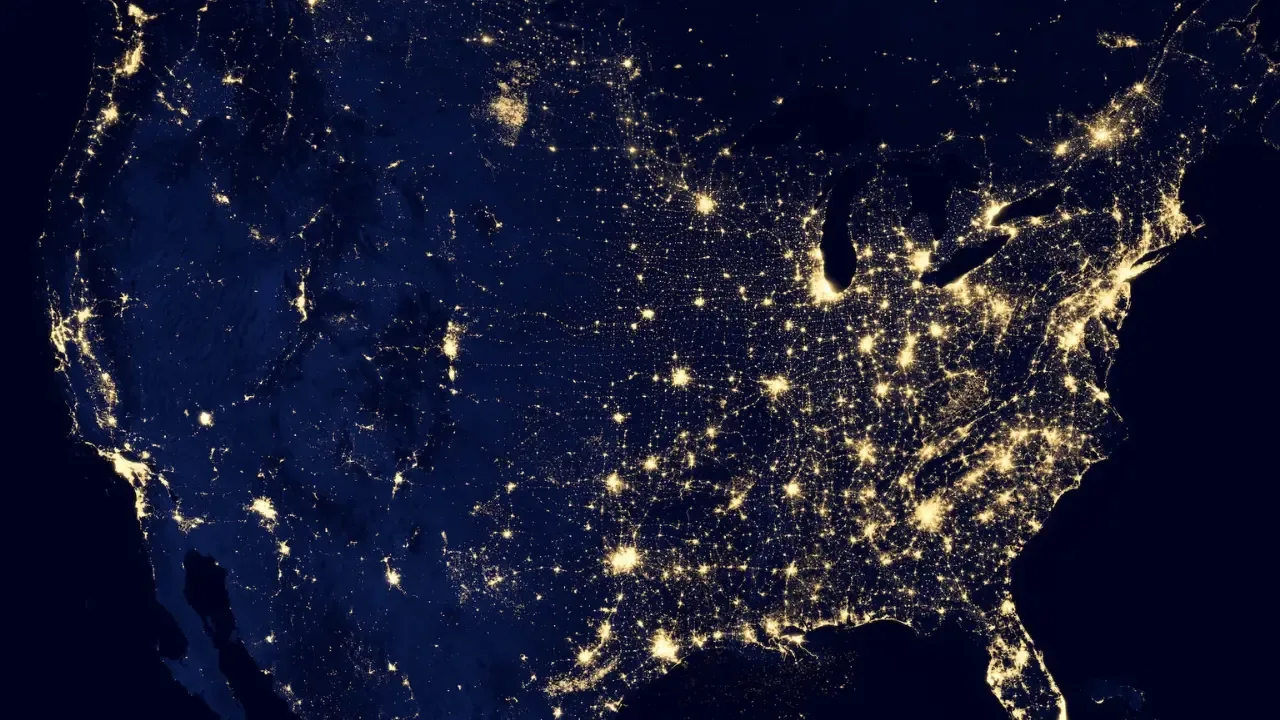
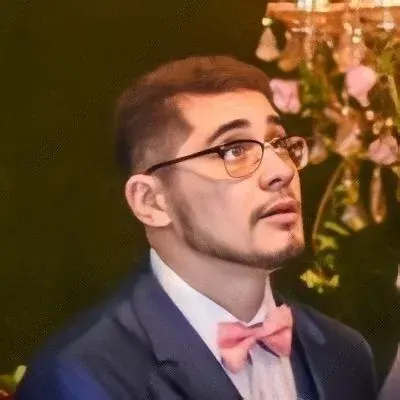
📝 How to apply a function to two columns of Pandas dataframe
Are you struggling to apply a function to two columns of a Pandas dataframe? Don't worry, you're not alone! It can be a bit tricky at first, but I'm here to help you out. In this blog post, I'll show you the common issues you may encounter and provide easy solutions to apply a function to two columns effortlessly.
💡 The Problem
Let's say you have a dataframe called df
with columns 'ID', 'col_1', 'col_2'
, and you want to apply a function to columns 'col_1', 'col_2'
to calculate a new column called 'col_3'
. You might try the following code:
df['col_3'] = df[['col_1','col_2']].apply(f)
But when you run it, Pandas throws a TypeError: ('<lambda>() takes exactly 2 arguments (1 given)'
. What's going on?
🔎 The Explanation
The error is being thrown because the apply
method is trying to pass each row of the selected columns as a single argument to the function. However, our function f
expects two separate arguments, one for each column.
🛠️ The Solution
To overcome this issue, we need to modify our approach. Instead of using apply
directly on the selected columns, we can use the apply
method on the dataframe itself and provide the axis=1
parameter. This will apply the function row-wise, allowing us to access each element of the selected columns individually.
Here's the revised code:
df['col_3'] = df.apply(lambda row: f(row['col_1'], row['col_2']), axis=1)
Now, the function f
will receive each element in 'col_1'
and 'col_2'
as separate arguments, and we can calculate the desired result for each row.
🌟 Example
Let's illustrate this with a practical example using the given sample:
import pandas as pd
df = pd.DataFrame({'ID':['1','2','3'], 'col_1': [0,2,3], 'col_2':[1,4,5]})
mylist = ['a','b','c','d','e','f']
def get_sublist(sta,end):
return mylist[sta:end+1]
df['col_3'] = df.apply(lambda row: get_sublist(row['col_1'], row['col_2']), axis=1)
print(df)
The output of this code will be:
ID col_1 col_2 col_3
0 1 0 1 ['a', 'b']
1 2 2 4 ['c', 'd', 'e']
2 3 3 5 ['d', 'e', 'f']
We successfully applied the function get_sublist
to columns 'col_1', 'col_2'
, and created the new column 'col_3'
with the desired results.
📣 Get Ready to Apply Functions Like a Pro!
Now that you know how to apply a function to two columns of a Pandas dataframe, you can tackle similar problems with confidence. Keep exploring the vast capabilities of Pandas and unlock the full potential of your data.
If you have any questions or want to share your experience, feel free to leave a comment below. Let's excel in data manipulation together! 😄💪
✨ Your Turn!
Have you ever encountered a situation where you needed to apply a function to multiple columns of a dataframe? How did you solve it? Share your stories and insights in the comments section below! Let's learn from each other and grow as a community.