How to add pandas data to an existing csv file?
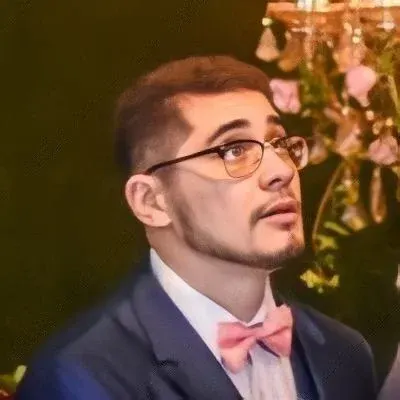

πΌπ Adding Pandas Data to an Existing CSV File? Here's How!
So, you have a shiny new dataframe in pandas πΌ and you want to seamlessly add it to an existing CSV file with the same structure... is it even possible? π€
The good news is, with pandas' powerful to_csv()
function and a few handy tricks, you can easily achieve this! In this guide, I'll walk you through the process step-by-step, addressing common issues along the way. Let's dive right in! π¦
The Scenario: Adding Data to an Existing CSV File
You have a CSV file that already contains some data and want to append your dataframe to it. Both the existing CSV file and your dataframe have the same structure. How can you accomplish this in pandas?
The Solution: Appending Data with to_csv()
To add your pandas dataframe to the existing CSV file, you need to use the to_csv()
function in 'append' mode. Here's the basic syntax:
df.to_csv('your_existing_file.csv', mode='a', header=False)
Let's break it down:
df
is the pandas dataframe you want to add to the CSV file.'your_existing_file.csv'
is the name (and path, if necessary) of your existing CSV file.mode='a'
specifies that you want to append data to the current file instead of overwriting it.header=False
ensures that the header is not written again, as the existing CSV file already has one.
And voila! Your dataframe will be gracefully added to the existing CSV file π.
Common Issues and Troubleshooting
Issue 1: Header duplication
If you notice that the header is duplicated after appending the data, make sure to set header=False
when using to_csv()
. By default, pandas includes the header in every call to to_csv()
, so including it again can result in duplicated headers.
Issue 2: Wrong data alignment
If the data in your appended dataframe does not line up correctly with the existing CSV file, double-check the column names, data types, and order of your dataframe's columns. They should match exactly with the existing CSV file.
Issue 3: Mismatched columns
In case your dataframe and the existing CSV file have mismatched column names or you want to include extra columns while adding, you can use pandas' df.reindex()
function to rearrange the columns before appending. This allows you to align the columns correctly and avoid data misalignment errors.
π£ Take Action: Share Your Experience!
Now that you know how to add pandas data to an existing CSV file, it's time to put your knowledge into practice! Try out the solution in your own code and let us know how it goes. Did you encounter any issues? How did you solve them?
Leave a comment and share your thoughts, experiences, or additional tips and tricks! Together, we can create a robust knowledge base and help fellow pandas enthusiasts. πͺπΌ
Remember, pandas' to_csv()
function is a powerful tool, and by understanding its various options, you can manipulate CSV files effortlessly. Happy coding! πβ¨
Take Your Tech Career to the Next Level
Our application tracking tool helps you manage your job search effectively. Stay organized, track your progress, and land your dream tech job faster.
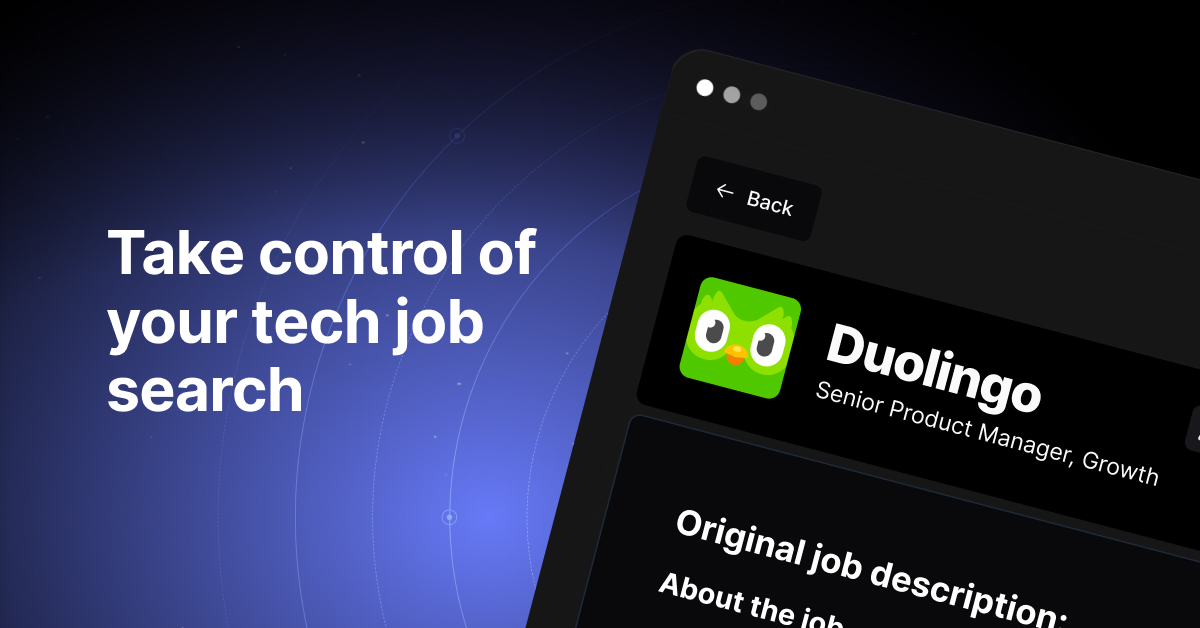